Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial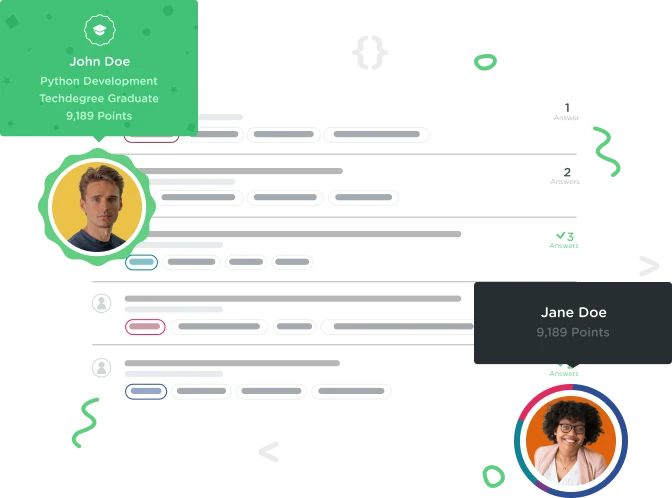
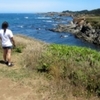
Nancy Melucci
Courses Plus Student 36,143 PointsWhy does this kill my code for part 1? (Python Basic Challenge Stage 5)
I get part 1 right, over and over again, then I enter code for part 2, and not only is it wrong, but it also blows up my part 1 code. It is so discouraging. I am never going to get past this challenge.
<
# add_list([1, 2, 3]) should return 6
# summarize([1, 2, 3]) should return "The sum of [1, 2, 3] is 6."
my_list = [1,2,3]
def add_list(my_list):
total = 0
for num in my_list:
total = total + num
return total
x= add_list(my_list)
print(x)
def summarize(my_list,x):
y=str(my_list)
print("The sum of {} is {}".format(y,x))
z = summarize(y,x)
7 Answers

John Sanchez
3,325 PointsFirst of all you should return, not print, the output of the summarize function. Second, you call summarize with the values y and x, but y is a local variable and is not defined outside of the summarize function. You should call summarize with my_list and x.
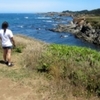
Nancy Melucci
Courses Plus Student 36,143 PointsI'm so annoying. I am looking at my code again. Should I be swapping x and y around in the function call at the end? Or in the format part? I just tried z = summarize(my_list, x) and it still gave me a type error. I'm afraid to edit again because part 1 will probably blow up.
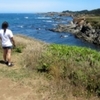
Nancy Melucci
Courses Plus Student 36,143 PointsI am trying to respond to your other question....just ignore that one. I am going to remove that one as soon as my browser will let me do that.
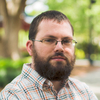
Kenneth Love
Treehouse Guest TeacherSorry this challenge is giving you so much trouble! If you have any insights on how to make this clearer, please let me know.
OK, let's check out your code. I'm gonna paste it here for future reference:
my_list = [1,2,3]
def add_list(my_list):
total = 0
for num in my_list:
total = total + num
return total
x= add_list(my_list)
print(x)
def summarize(my_list,x):
y=str(my_list)
print("The sum of {} is {}".format(y,x))
z = summarize(y,x)
First off, as far as challenges go, you never have to call the functions yourself or create things like my_list
. The Code Challenge will do all of that for you (that's how I make sure the code you wrote does what I want it to). But that won't make your challenge fail.
Your add_list
looks great. Good work, there! So, let's look at summarize
. summarize()
is supposed to take a list and then return a string. If, say, the list was [1, 2, 1]
, the response should be The sum of [1, 2, 1] is 4.
. (You're missing a period at the end of the response, btw)
In your summarize()
, you're expecting two arguments. summarize()
is only ever going to get one, though (the list to add), so that's going to cause the challenge to fail (write a function that takes two arguments in the shell and then only give it one to see how it fails). You're using .format()
correctly, but not giving it the right items. Your y
variable is fine, but your x
should be the total of the list. I bet you can find a way to get that based on what you did in step 1, huh?
Lastly, be sure you return
from your functions in CCs instead of just print()
ing.
Good luck and be sure to send me some ideas!
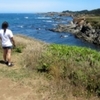
Nancy Melucci
Courses Plus Student 36,143 PointsI am sorry. I am confused. Should I have one argument, or two, in my summarize? I cannot follow. It isn't helping that my IDE is taking this code and running it. And then it fails here. And that I have to keep going back to task 1. Can you clarify the point for me? I spotted the period. Also, I saw that the code challenge called for y to be the sum and x to be the string. I apologize for the two threads and the rest of it.
Nancy M.
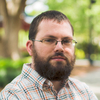
Kenneth Love
Treehouse Guest Teachersummarize only takes one argument.
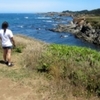
Nancy Melucci
Courses Plus Student 36,143 PointsHere's the new version of the end of the code:
def summarize(my_list, y):
x = str(my_list)
return("The sum of {} is {}." .format(x,y))
y = add_list(my_list)
print summarize(my_list)```
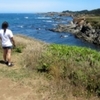
Nancy Melucci
Courses Plus Student 36,143 PointsThanks. I did it. See my email. Thanks very much.
Nancy M.