Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
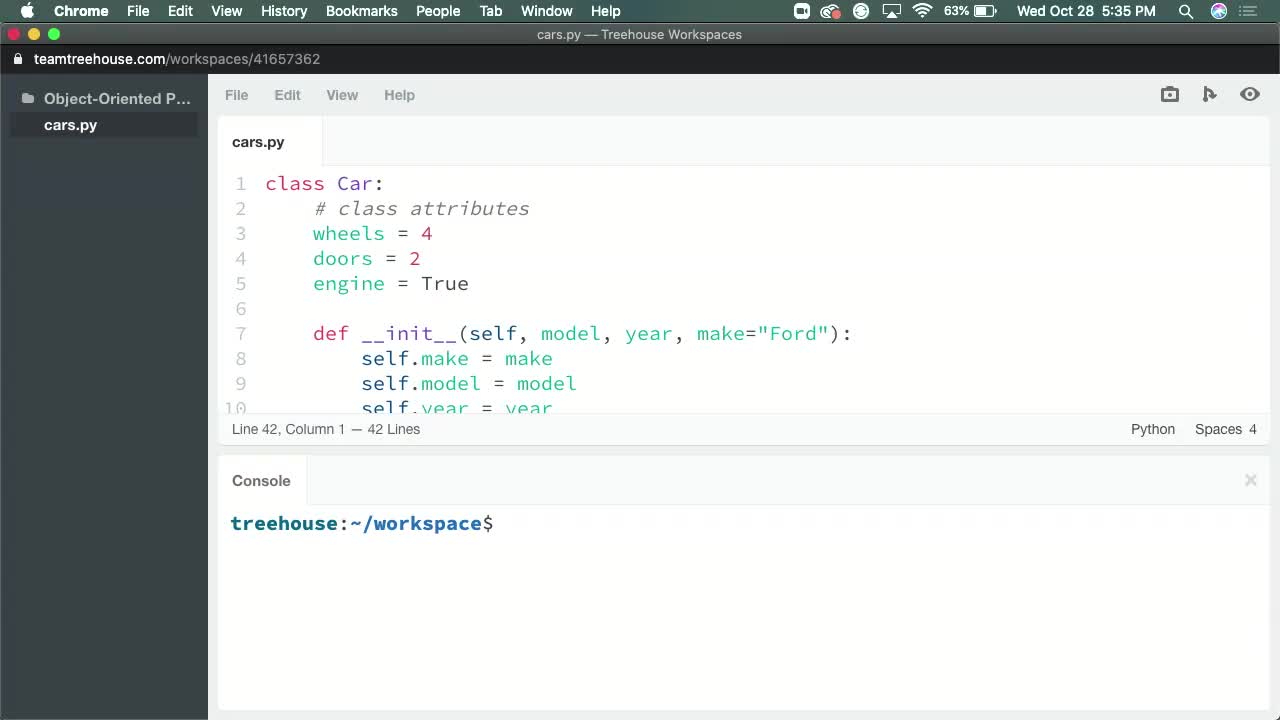
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
In this step, learn how to tackle iterations using your created objects.
class Candy:
def __init__(self, name, color):
self.name = name
self.color = color
def __str__(self):
return f'{self.color} {self.name}'
class CandyStore:
def __init__(self):
self.candies = []
def __iter__(self):
yield from self.candies
def add_candy(self, candy):
self.candies.append(candy)
nerds = Candy('Nerds', 'Multi')
chocolate = Candy("Hersey's Bar", 'Brown')
my_store = CandyStore()
my_store.add_candy(nerds)
my_store.add_candy(chocolate)
for candy in my_store:
print(candy)
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
Karyna Reut
1,611 Points1 Answer
-
Sam Appleton
3,832 PointsUsing (self,*cars) instead of (self,cars) for multiple instances
Posted by Sam AppletonSam Appleton
3,832 Points1 Answer
-
Will Duong
94 Pointspython keeps not liking "add_car(one/two/three)", keeps saying 'add_car' is not defined
Posted by Will DuongWill Duong
94 Points1 Answer
-
Kylie Nonemaker
1,190 Points2 Answers
-
amandae
15,727 Points3 Answers
-
Guillaume Crt
6,395 Pointsusing __init__ method instead of class attribute in this example ?
Posted by Guillaume CrtGuillaume Crt
6,395 Points2 Answers
-
aaron roberts
2,004 Points3 Answers
-
jayda hendrickson
3,413 Points1 Answer
-
Mohamed Abdelaal
Python Development Techdegree Student 106 Points1 Answer
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up