This course will be retired on July 14, 2025.
Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
Preview
Video Player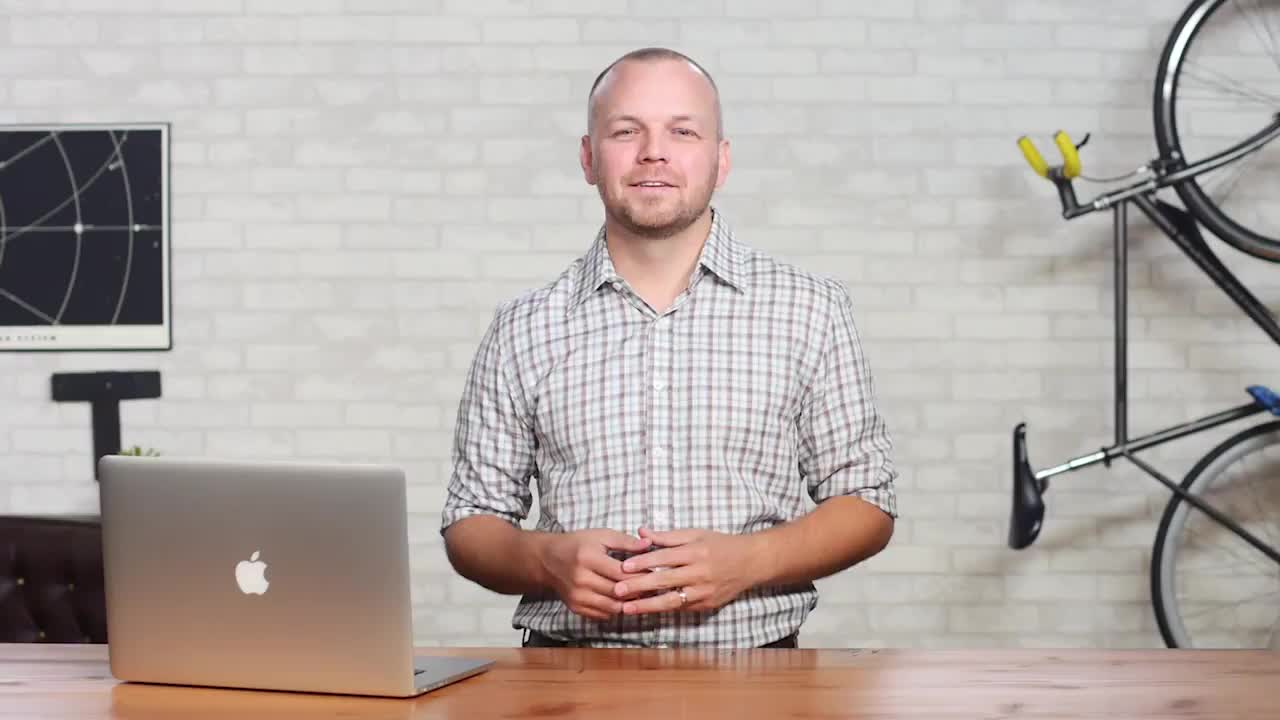
00:00
00:00
00:00
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Arrays are fast for retrieving and updating items, but there are faster collections for adding, removing, and searching for items.
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
Earlier in this course,
we learned what arrays are good at.
0:00
They're blazingly fast at retrieving or
0:04
setting an item when we already know
the index we want to set or retrieve.
0:06
So, what are they not great for?
0:11
The items in the array are stored in
the computer's memory, one after another.
0:13
Right after the end of an array,
the computer can store other data.
0:17
This is in order to make the most
use of the limited memory space that
0:21
a computer has.
0:25
This is also why arrays
have a fixed length.
0:26
In order to store data right
after the end of the array,
0:29
the computer needs to know
how long the array is.
0:33
Once we've created an array of a certain
length, we can't change our minds and add
0:37
more space to the end of it, because we'd
be stomping on data that's already there.
0:41
So what do we do if we have an array
that's three items long and
0:46
we want to add a fourth item to the end?
0:50
The array is full, so
there's no room to put more items.
0:52
Similarly, what if we want to insert
an item at the beginning of an array or
0:56
somewhere in the middle?
1:00
Let's investigate this
problem with some code.
1:02
Let's create an array of three integers
to keep track of people's ages.
1:06
So I'll say int[] ages = { 24, 31, 56}.
1:13
Now let's attempt to add another
age to the end of the array.
1:21
So I'll say ages[]ages.Length = 64.
1:25
We get a System.IndexOutOfRangeException,
1:33
because the array is only three items
long and we're trying to add a fourth.
1:36
What if we wanted to add another age,
but keep the ages in the array sorted?
1:41
So if we wanted to add 16, we'd need to
add it to the beginning of the array at
1:46
index 0, but
24 is already at index 0 here.
1:51
We'd also be adding a fourth item to
an array that only has room for three.
1:56
The only way to add a fourth item
is to create a larger array and
2:02
copy everything from our
original array to the new array.
2:07
Every array inherits
from a base array class.
2:11
Let's take a look at the array
class documentation.
2:14
You can find this by searching for
system data array, or
2:18
just click on the link
in the teacher's notes.
2:22
This class contains many methods
that can help us deal with the race.
2:28
The method we want is the CopyTo method.
2:34
So scroll down here and find CopyTo.
2:38
Here we go.
2:44
It says, copies all the elements of
the current one dimensional array
2:45
to the specified one dimensional array,
starting at the specified array index.
2:49
So first we need to create a new
array that is the size we want.
2:55
So I'll say int[]
3:00
agesCopy = new int[4].
3:04
Now we can copy everything
from ages into agesCopy.
3:11
If we want to insert an age at the begging
of our array, then we'll want to put
3:16
the new items starting at
index one of agesCopy.
3:20
So I'll say ages.CopyTo(agesCopy,
and we'll start at index 1.
3:27
Now if we look at the contents of
agesCopy, we'll see that our three
3:34
items are in there and we have a space
to put a fourth item at index 0.
3:39
So now I can say, agesCopy[0] = 16.
3:44
We do something similar if we
wanted to add an item at the end or
3:55
somewhere in the middle of the array.
3:58
As you can see, it's not easy
to add an item to a full array.
4:01
Finally, we want to overwrite the ages
array with the agesCopy array.
4:06
However, we need to be careful here.
4:11
Consider the situation where
another class in our program
4:14
already has a reference to the ages array.
4:18
Remember this ages here is only
a variable that refers to the ages array.
4:21
Even if we set ages equal to agesCopy,
other parts of
4:28
the program that are referring to
this array won't see this change.
4:32
We've only changed one variable.
4:37
If If we wanted to make it so the all
parts of our program that we're referring
4:40
to the original array are now referring
to our new array, we'd need to go and
4:44
reset all of those variables.
4:49
This seems like a lot of trouble just
to add one more item to an array
4:52
not to mention it's very
slow to add just one item.
4:56
Creating a second array and
5:00
copying everything every time we want
to add an item is very inefficient and
5:01
can slow down a computer
program if we do this a lot.
5:06
We also run into the same sort of
troubles when we want to remove
5:09
items from an array.
5:12
In that case we need to shift all
of the items after the removed item
5:14
over by one to fill in the missing index.
5:18
As you can imagine, this makes removing
items from an array very slow.
5:21
Although arrays are very fast for
retrieving and
5:26
setting items, they're the slowest
collection for adding or removing items.
5:28
Due to the difficulty in adding,
removing and
5:34
resizing, arrays are rarely
used directly like this in C#.
5:36
Instead there's a collection type
that works just like an array, but
5:41
it takes care of all this copying for us.
5:45
This makes it easier to add and
remove items.
5:48
This collection is called a list.
5:51
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up