Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
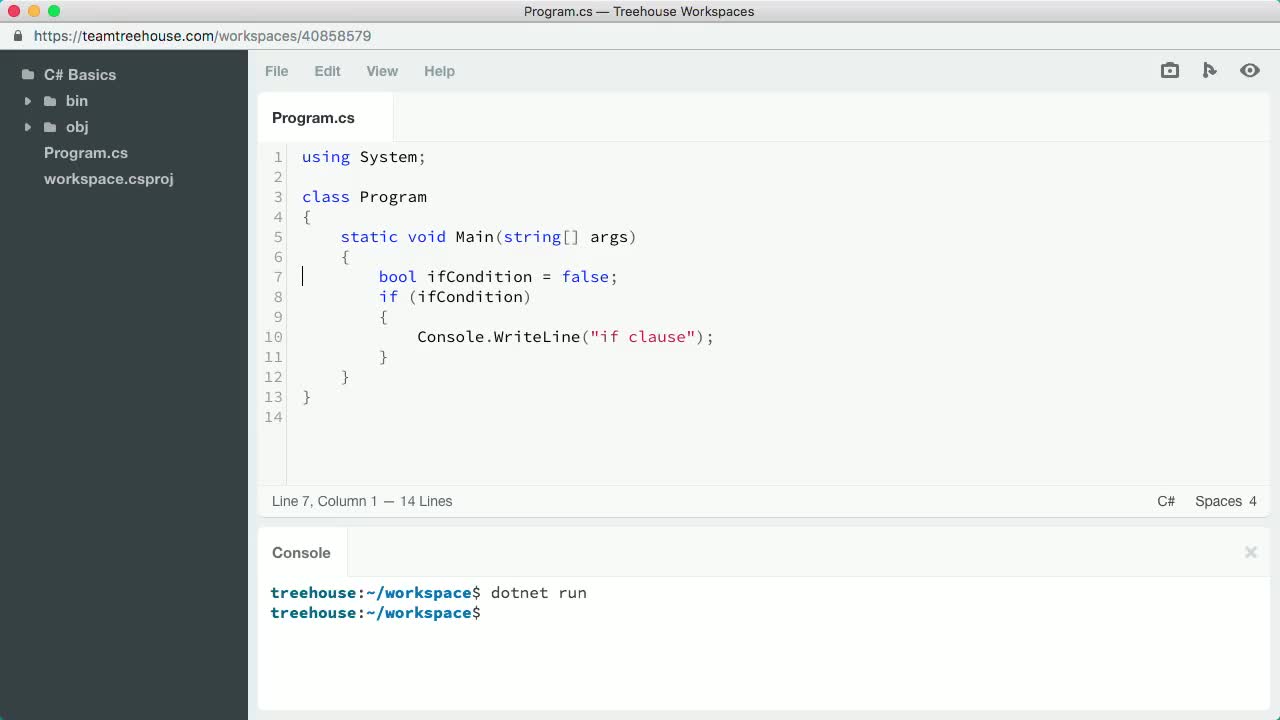
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
If you have some code that you want to run when the "if" statement doesn't run, you can add an "else" clause.
- If the condition of an
if
statement is false, the code in its body won't run.
bool ifCondition = false;
if (ifCondition)
{
Console.WriteLine("if clause");
}
- If you have some code that you want to run when the
if
statement doesn't run, you can add anelse
clause. - You add the
else
keyword right after theif
statement's block, followed by another curly brace block with one or more lines of code that you want to run instead of theif
statement's code. - C# doesn't require it, but it's conventional to have the
else
keyword aligned with theif
keyword, and theelse
code indented one level. Again, it just makes the code easier to read.
else
{
Console.WriteLine("else clause");
}
- If the
if
statement's condition is true, then theelse
clause won't run:bool ifCondition = true;
[then change back] - If you have other conditions that you want to test only when the
if
condition is false, you can add one or moreelse if
clauses between theif
andelse
clauses. - You add the keywords
else
andif
, another condition expression, and some code you want to run if that condition is true. - As with the
else
clause, theelse if
keywords should be lined up with theif
keyword, and the code following it should be indented one level.
bool ifCondition = false;
// new
bool elseIfCondition = true;
// old
if (ifCondition)
{
Console.WriteLine("if clause");
}
// new
else if (elseIfCondition)
{
Console.WriteLine("else if clause");
}
//old
else
{
Console.WriteLine("else clause");
}
- If the
if
clause istrue
, then neither theelsif
clauses nor theelse
clause will be run. - If the
if
condition and allelse if
conditions are false, then the else clause will be run. - The
else
clause isn't required. If all conditions are false and there's noelse
clause, then no code will be run.
Let's use what we've learned to fix our store program's Price
function.
static double Price(int quantity)
{
double pricePerUnit;
if (quantity >= 100)
{
pricePerUnit = 1.5;
}
else if (quantity >= 50) // Add "else"
{
pricePerUnit = 1.75;
}
else if (quantity < 50) // Add "else"
{
pricePerUnit = 2;
}
return quantity * pricePerUnit;
}
- This addresses the issue where quantities over 100 would be assigned a
pricePerUnit
of $1.50 and then getpricePerUnit
reassigned to $1.75.- The
else if
condition only gets evaluated when theif
condition is not true. - So if quantity is 100 or more, the
if
condition will be true. -
pricePerUnit
will get assigned $1.50. - And although a quantity of 100 is also greater than a quantity of 50, the
else if
condition will never even get evaluated, because theif
condition was true.
- The
- So that's one problem fixed.
- But we're still getting the error "Use of unassigned local variable 'pricePerUnit'".
- That's because as far as C# can tell, it's still possible to reach the
return
statement, wherepricePerUnit
gets accessed, without having assigned to it. - We need to set up our code in such a way that
pricePerUnit
will get assigned to, no matter what. - You and I can actually tell that
pricePerUnit
will be assigned no matter what.- If the quantity isn't greater than or equal to 100...
- And it also isn't greater than or equal to 50...
- Then it for sure will be less than 50.
- So one of these three branches is always going to be run.
- But C# can't tell this. And so it's giving us an error.
- To fix this, let's try removing the
if
and the condition from this lastelse if
clause.
static double Price(int quantity)
{
double pricePerUnit;
if (quantity >= 100)
{
pricePerUnit = 1.5;
}
else if (quantity >= 50)
{
pricePerUnit = 1.75;
}
else
{
pricePerUnit = 2;
}
return quantity * pricePerUnit;
}
- We really don't need it, because if
quantity
isn't greater than or equal to50
, it must be less than50
.- So now all we have is an
else
clause. Even if theif
and theelse if
clauses don't run, theelse
clause will. - Let's try running this now.
- The error goes away, and our program appears to be working correctly.
- Since
pricePerUnit
gets assigned in all three of these scenarios, C# can tell thatpricePerUnit
is going to have a value when it gets accessed, no matter what.
- So now all we have is an
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
Ra Bha
2,201 Points2 Answers
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up