Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
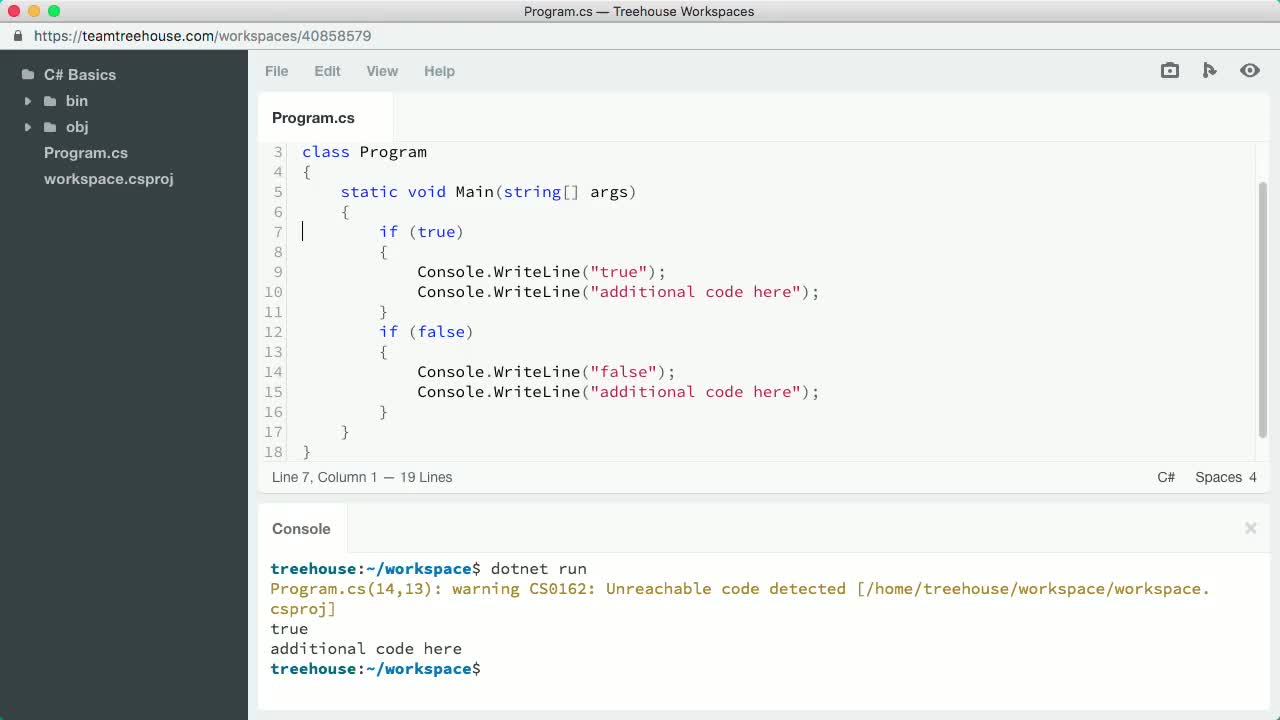
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
We've learned about comparison operators, so we can test whether the user wants to order 50 or more cans, or 100 or more. Now we just need to add code that applies a discounted price if those conditions are true.
We've learned about comparison operators, so we can test whether the user wants to order 50 or more cans, or 100 or more. Now we just need to add code that applies a discounted price if those conditions are true.
- Like most programming languages, C# has an
if
statement that executes some code only if a condition expression is true. - Starts with
if
keyword- followed by condition, which in these examples are the boolean values
true
andfalse
- followed by an opening curly brace
- followed by one or more lines of code (like a method body, these are usually indented to make them easier to read, although this isn't enforced)
- followed by closing curly brace
- followed by condition, which in these examples are the boolean values
- Because condition for first
if
alwaystrue
, code it contains will always be run. - Because condition for second
if
alwaysfalse
, code it contains will never be run.- We get an error when attempting to run it: "warning CS0162: Unreachable code detected"
- This isn't an actual error, so the program will run anyway, but C# does try to warn you that you've written code that won't be run under any circumstances.
if (true)
{
// This code will always be run.
Console.WriteLine("true");
Console.WriteLine("additional code here");
}
if (false)
{
// This code will never be run.
Console.WriteLine("false");
Console.WriteLine("additional code here");
}
- Just putting the values
true
andfalse
isn't very useful, though. - Actual programs will use expressions that evaluate to
true
orfalse
, like comparison operators.- Again, notice that we get a warning about the middle
if
statement. Because 75 is always going to be less than 100, the condition will always evaluate tofalse
, and the code will never be run.
- Again, notice that we get a warning about the middle
if (75 > 50)
{
// This code will always be run.
Console.WriteLine("75 > 50");
}
if (75 > 100)
{
// This code will never be run.
Console.WriteLine("75 > 100");
}
if (50 == 50)
{
// This code will always be run.
Console.WriteLine("50 == 50");
}
- Actual programs aren't likely to compare hard-coded numbers, either. Usually, they'll test the value of a variable.
- Notice that this time, the warning goes away.
- Because we're using a variable in our comparisons, we could theoretically change its value to something else:
int number = 50;
- If we do that,
if
conditions that previously evaluated tofalse
could change totrue
, and vice-versa, which is howif
statements usually work in practice. So there's nothing to warn us about.
- Because we're using a variable in our comparisons, we could theoretically change its value to something else:
int number = 75;
if (number > 50)
{
// This code will always be run.
Console.WriteLine("number > 50");
}
if (number > 100)
{
// This code will never be run.
Console.WriteLine("number > 100");
}
if (number == 50)
{
// This code will never be run.
Console.WriteLine("number == 50");
}
- Let's try using
if
in our program:
static double Price(int quantity)
{
if (quantity >= 100)
{
pricePerUnit = 1.5;
}
if (quantity >= 50)
{
pricePerUnit = 1.75;
}
if (quantity < 50)
{
pricePerUnit = 2;
}
return quantity * pricePerUnit;
}
- Whoops! We get errors:
Program.cs(10,13): error CS0103: The name 'pricePerUnit' does not exist in the current context [/home/treehouse/workspace/workspace.csproj]
Program.cs(14,13): error CS0103: The name 'pricePerUnit' does not exist in the current context [/home/treehouse/workspace/workspace.csproj]
Program.cs(18,13): error CS0103: The name 'pricePerUnit' does not exist in the current context [/home/treehouse/workspace/workspace.csproj]
Program.cs(20,27): error CS0103: The name 'pricePerUnit' does not exist in the current context [/home/treehouse/workspace/workspace.csproj]
- That's right, we forgot to declare the
pricePerUnit
variable before assigning to it. - I'll go up to the first instance and make it a declaration:
static double Price(int quantity)
{
if (quantity >= 100)
{
double pricePerUnit = 1.5;
}
if (quantity >= 50)
{
pricePerUnit = 1.75;
}
if (quantity < 50)
{
pricePerUnit = 2;
}
return quantity * pricePerUnit;
}
- Hmm, that's strange. Now we get three errors and a warning:
Program.cs(14,13): error CS0103: The name 'pricePerUnit' does not exist in the current context [/home/treehouse/workspace/workspace.csproj]
Program.cs(18,13): error CS0103: The name 'pricePerUnit' does not exist in the current context [/home/treehouse/workspace/workspace.csproj]
Program.cs(20,27): error CS0103: The name 'pricePerUnit' does not exist in the current context [/home/treehouse/workspace/workspace.csproj]
Program.cs(10,20): warning CS0219: The variable 'pricePerUnit' is assigned but its value is never used [/home/treehouse/workspace/workspace.csproj]
- This last warning especially is interesting: "The variable 'pricePerUnit' is assigned but its value is never used"
- We use the
pricePerUnit
variable right here when we're calculating a return value! Why is it saying it's never used?
- We use the
- Remember we showed you that variables declared within a function are only in scope within that function?
- The same is true for
if
statements. If you declare a variable within anif
statement's block, it's only in scope within that block.
- The same is true for
- The solution is to declare the variable before any of the
if
blocks. Then it will be in scope for all of them, as well as afterward.
static double Price(int quantity)
{
double pricePerUnit;
if (quantity >= 100)
{
pricePerUnit = 1.5;
}
if (quantity >= 50)
{
pricePerUnit = 1.75;
}
if (quantity < 50)
{
pricePerUnit = 2;
}
return quantity * pricePerUnit;
}
- But we still get an error when we use
pricePerUnit
to calculate a return value. It says "Use of unassigned local variable 'pricePerUnit'".- Why is that? We assign values to
pricePerUnit
in the lines above! - The problem is that we assign to
pricePerUnit
in theif
blocks. What if none of theif
blocks had a true condition? - None of them would run, and we'd get to the
return
statement with no value assigned topricePerUnit
! - Now, you and I know that's not actually possible. No matter what value is assigned to
quantity
, one of those threeif
statements is going to be true. A value will be assigned topricePerUnit
no matter what. - But C# doesn't know that. All it sees is a possibility that we'll reach the
return
statement with no value assigned topricePerUnit
. And the result is an error. - So we need a way to say that one of those three
if
statements will be evaluated, no matter what.
- Why is that? We assign values to
- There's an additional problem here, too.
- This code works fine for
quantity >= 50
andquantity < 50
, but ifquantity >= 100
,price_per_unit
gets set to1.75
because100
is also>= 50
.
- This code works fine for
Up next, we'll look at else if
and else
clauses for if
statements, which will help us fix these issues.
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
Ra Bha
2,201 Points1 Answer
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up