Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
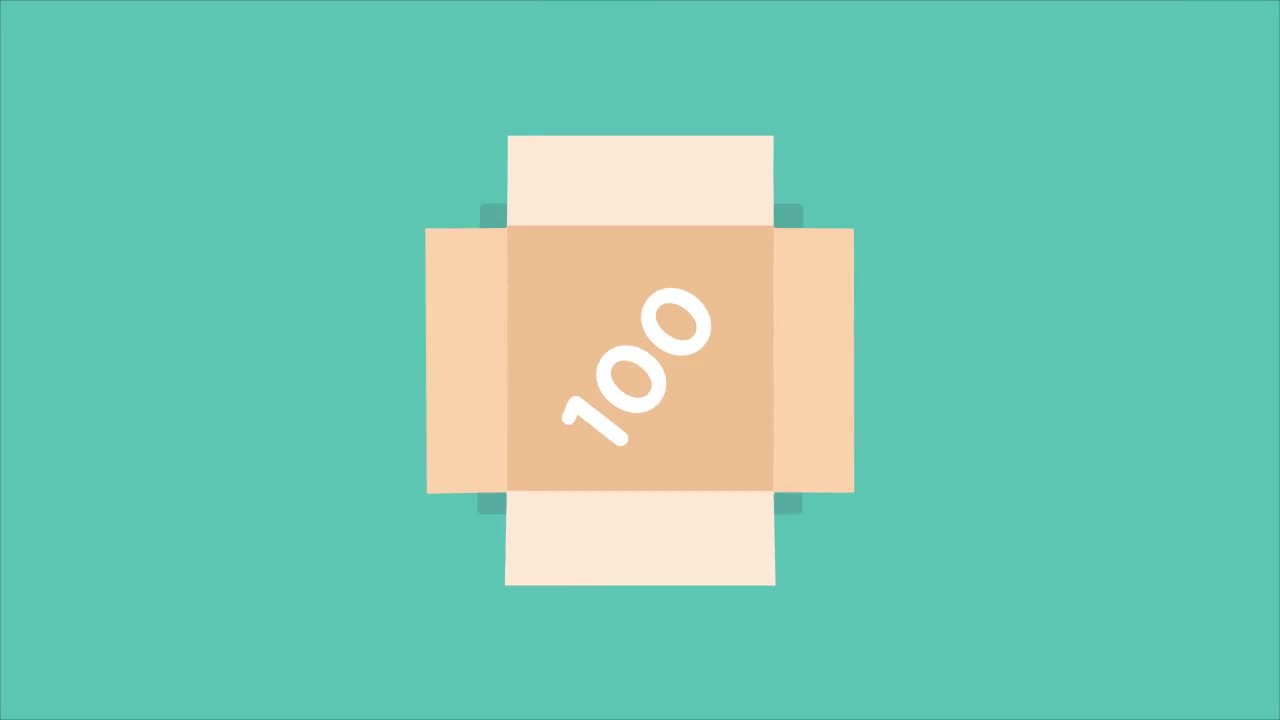
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Learn how to use variables to store and track information, as well as use and manipulate information.
Resources
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
Albert Almonte
1,824 Points0 Answers
-
David McCall
217 Points2 Answers
-
asia flowers
2,496 Points2 Answers
-
Karl Russell Sumando Menil
Full Stack JavaScript Techdegree Student 4,004 Pointsthis did not print the second message just the first one:
1 Answer
-
JAAFAR CHRAIBI
1,839 Points4 Answers
-
PLUS
Annaliese Laurila
Courses Plus Student 3,265 Points1 Answer
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up