Start a free Courses trial
to watch this video
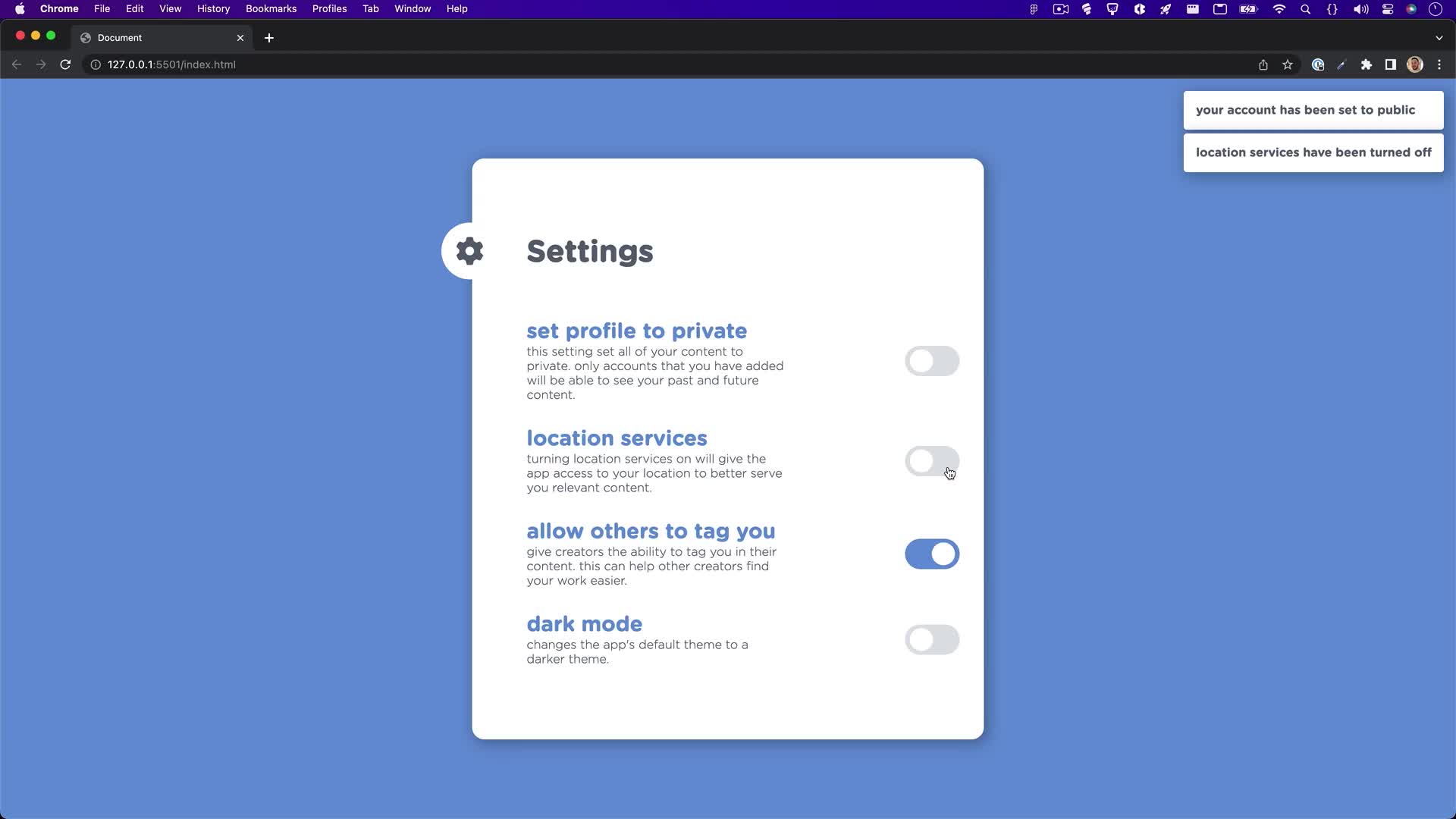
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Dustin puts forEach() into practice in a simple settings app that includes toggle switches and status messages based on the user's input.
Treehouse Content
Resources
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upI know what you're thinking. 0:00 This is pretty cool, but how or why would I need to use this in a practical way? 0:01 Well, if you've not had much experience building out complex websites and 0:07 applications, you might have not needed to loop over something like data before. 0:11 Check out this settings application that I made. 0:15 While this is pretty simple and only has four settings in the menu, you can see how 0:19 tedious this would be to build out if there were hundreds of settings in the UI. 0:24 So, what I did was I created an array to hold all of the data for the settings, 0:28 and then loop over them to display them in the UI. 0:33 I also have this notification section for 0:36 letting the user know that their setting has been applied. 0:38 I was able to do this pretty easily by using the index parameter with forEach. 0:43 So let's jump into the code and see exactly how this was done. 0:47 The first thing you'll notice in my app.js file is an array called settings. 0:52 As mentioned earlier, this array holds all the data I need for my settings. 0:56 Let's go over each item in the array really quickly. 1:01 Each toggle switch in the UI is actually a checkbox. 1:04 So we'll need it to have a unique ID that it shares with the label so 1:08 that the user can click on either the toggle switch or the text content. 1:11 The label holds a couple of paragraph tags that hold our title in description. 1:16 Next, you'll see checked. 1:22 This is set to either true or false. 1:24 And that is so that when the page loads, 1:26 the setting is either toggled on or off by default respectively. 1:29 Lastly, you'll see notification. 1:33 And this is an object that holds two properties, on and off. 1:36 This is the notification text based on the state of the toggle switch. 1:40 Now that we understand what each item in our array holds, let's look at the rest 1:44 of the code before diving into how we use forEach to display it on the page. 1:49 On line 48, you'll see a function. 1:54 This function just creates an individual setting in the UI. 1:57 I won't go over how this is done since this is outside of the scope of this 2:01 video, but if you have a basic understanding of JavaScript, 2:05 this is pretty straightforward. 2:08 Feel free to pause the video and take a look. 2:10 But basically, we're just creating a function that takes in a unique ID, 2:12 title, description, 2:17 and checkedState as parameters to build a single setting in the page. 2:19 This function won't actually do anything until we call it. 2:25 So what we'll wanna do is loop over each setting in our settings array, and 2:27 run this function.r Next, on line 72, you'll notice another function. 2:32 This one is titled createNotification. 2:37 And it does exactly that. 2:40 This function will create a notification with whatever text content you pass 2:42 in as a parameter. 2:46 So we'll need to make sure to get the correct settings notification text when we 2:48 call this function. 2:52 We'll go over how to do this in just a little bit. 2:54 On line 89, you'll see a loop. 2:57 This is a standard for loop. 3:00 I mentioned earlier I'll show you the difference between a for loop and 3:02 using the forEach method. 3:05 While they both can accomplish the same task, 3:07 there are some slight differences, for one syntax. 3:10 I find the forEach method is easier to read, understand, and write. 3:14 If you look at the for loop, you'll see we are setting the variable named i to 0. 3:19 And while i is less than the length of our settings array, 3:25 we want to increase the value of i by 1. 3:28 So each time the loop runs, i is incremented by 1 until it's no longer less 3:31 than the length of our settings array. 3:35 Each time through the loop we are calling our create setting function using 3:38 the settings array. 3:42 The index of the settings array comes from the value of i each time through the loop. 3:44 So the first time through the loop, i = 0. 3:49 So we call our createSetting function using the first index of our 3:53 settings array. 3:57 And we grab the unique ID, title, description, and checkedState. 3:59 The function then builds out our UI and appends it to the page. 4:04 i is then incremented by 1 bringing its value to 2. 4:08 And now the loop runs again, but with the second index of our settings array. 4:12 And this process keeps going until i is no longer less than the settings array's 4:16 length. 4:21 As you can see, this works perfectly and 4:23 builds out all of our settings from the data in our settings array. 4:24 Now let's comment out the for loop code and 4:28 rewrite this using our forEach method on the settings array. 4:31 We can start by writing settings, which is our array name, followed by forEach. 4:36 We can set our element parameter to setting. 4:41 Next, through each iteration of our settings array, 4:45 we want to run the createSetting function using the current settings unique ID, 4:49 text, description, and checkedState. 4:54 Remember, this element parameter that we set to setting, 4:58 holds the current value of our array. 5:01 So the first time through the loop, 5:04 we are actually at the first index of our settings array and so on and so on. 5:06 So basically, what we're writing is settings.forEach setting 5:12 createSetting using the respective data. 5:16 So let's hit Save and the browser now shows all of our settings again. 5:20 In my opinion, this forEach syntax is much easier to work with than your standard for 5:25 loop, though both loops work perfectly fine for this scenario. 5:30 Now let's check out how we can use the index parameter in our forEach method to 5:34 display the correct notification when a user toggles a setting on or off. 5:39 Let's create a variable to hold all of our toggle switches. 5:45 Remember, these are just checkbox inputs with some CSS styling to make them look 5:48 like toggle switches. 5:53 If you'd like to learn how to make them, check the teachers notes or description 5:55 for a video where I go over how to do that with just CSS pretty easily. 5:59 We'll set this variable to document.querySelectorAll. 6:03 And we'll target all inputs that are children of the setting class. 6:07 Because we used querySelectorAll, our variable holds a collection of items. 6:13 So we are able to run the forEach method on this. 6:18 So let's do that. 6:21 All right, settingToggles.forEach, and set the element parameter to toggle. 6:23 And we'll add in the index perimeter as well. 6:30 What I'll do first is add an event listener to each one of these inputs. 6:33 Because it's a checkbox input, we have access to the change event listener. 6:38 So let's write toggle.addEventListener change. 6:43 I'll write a simple if statement to test if our current toggle switch input is 6:48 checked or not. 6:52 And if it is, 6:54 we want to call our createNotification function with the on notification message. 6:54 Else, do the same thing, but use the off notification message. 7:00 So for now, let's just call our functions and 7:05 I'll explain how we can get access to the right message for our message parameter. 7:08 This next part might seem a little tricky to understand. 7:14 But because we are looping over DOM elements instead of our settings array, 7:17 you're probably wondering how we will have access to the current settings 7:21 array element that we need for the notification message. 7:25 Well, because our DOM elements were created from our settings array, 7:29 we have the same amount of DOM elements that we are looping over as we do array 7:33 elements in our settings array. 7:38 So we can just use the current index value through our forEach method on the DOM 7:41 elements to access the correct settings array element and 7:45 grab the respective notification message. 7:49 So for the first part of our condition, we'll call our function, and for 7:52 the message parameter, 7:57 we'll write settings at the current index.notification.on. 7:59 And for the else portion, 8:03 we'll just write settings at the current index.notification.off. 8:05 So let's quickly go over what we just wrote. 8:15 We have a variable named settingToggles that holds all of our toggle switches. 8:18 We are running the forEach method on this variable since this variable holds 8:23 a collection of elements. 8:27 Through each iteration, the variable toggle holds the current element, 8:30 while index holds that element's index. 8:35 We write an if statement to check if that toggle is checked or not. 8:38 And if it is, we call our createNotification function using 8:42 the settings array at the current index. 8:46 Because each element in our settings array has a notification object, 8:49 we have to specify which property we are looking for. 8:53 In this first condition of our if statement, we are looking for 8:57 the notification.on property. 9:01 In the else portion of our if statement, we are looking for 9:03 the notification.off property. 9:07 Let's now check the browser to see if this all worked. 9:10 Sweet, when I toggle the setting, the correct setting message appears. 9:15 I hope this guide was helpful. 9:19 Using the forEach method can really come in handy when needing to loop over 9:21 the data in your arrays or even just working with a collection of DOM elements. 9:24 I've only covered the JavaScript portion of the settings menu, but 9:29 if you'd like to see how I built out the UI, be sure to let me know. 9:33 Also, remember, I'll link a video down below in the teachers notes or description 9:37 on how you can set up your checkbox inputs to look like toggle switches. 9:42 I'll see you in the next one. 9:46 And until then, have fun and happy coding. 9:47
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up