Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
Well done!
You have completed Practice Writing Loops in Python!
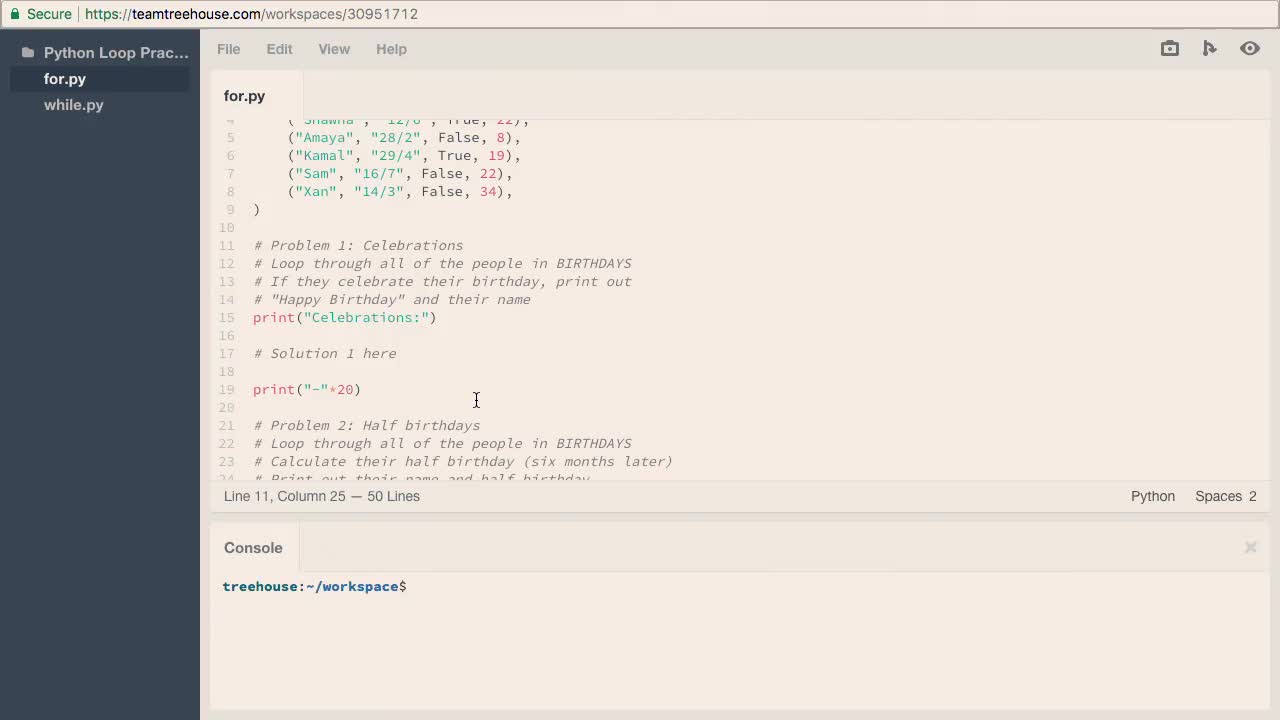
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Here's how I did it!
This video doesn't have any notes.
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
Caroline Todeschini
3,444 Points1 Answer
-
amandae
15,727 Points1 Answer
-
Tanner B
926 Points1 Answer
-
PLUS
Brett McGregor
Courses Plus Student 1,903 Points1 Answer
-
David Gabriel
Python Web Development Techdegree Student 979 Points2 Answers
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up