Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial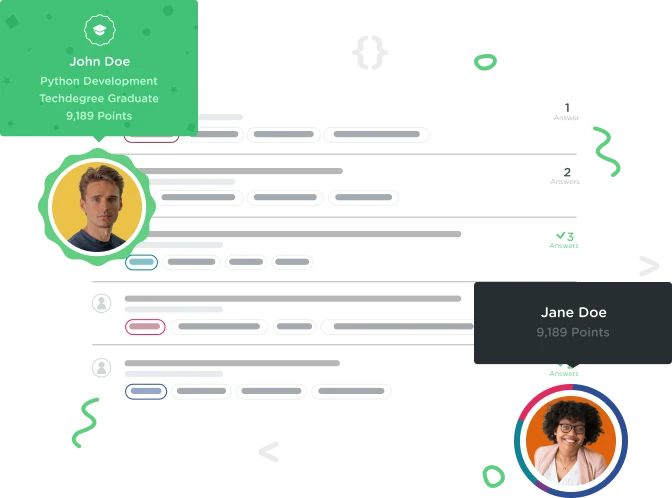
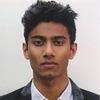
Samuel Kleos
Front End Web Development Techdegree Student 12,719 PointsA history of error catching for readyState and status since xhr?
I'm thinking back to making requests and catching network errors. I'm desperately trying to align everything together.
In terms of the callback queue,
- Are xhr (XHRHttpRequest()) objects still the WebAPI that manages async requests?
- Or have requests been handed to a promise Web API now that requests are made inside Promise() objects?
- Can you confirm the following parallels as false or accurate?
Back in the XHRHttpRequestObject() days:
- Network Errors caught with
.readyState
- Response status problems caught with
xhr.status
if (xhr.readyState === 4) {
if (xhr.status === 200) {
// do the things. the DOM things.
} else {
console.log(roomsJSON.status);
}
}
Then a bit more recently with
- Network Errors checked by
.onload
and.onerror
- Response errors still checked by
xhr.status
return new Promise( (resolve, reject) => {
const xhr = new XMLHttpRequest();
xhr.open('GET', url);
xhr.onload = () => {
if (xhr.status === 200) {
resolve(JSON.parse(xhr.responseText))
} else {
reject( Error(xhr.statusText));
}
}
xhr.onerror = () => reject( Error("A network error occured"));
xhr.send();
});
Then now to the present day with async/await keeping async operations for fetching and parsing a response body separate:
- await fetch(URL)
- await response.json();
async function fetchJSON(url) {
try {
const response = await fetch(url)
return await response.json();
} catch (err) {
throw err;
}
}
Am I correctly identifying the parallels in the history error-catching?
I desperately need your help to align my understanding because it's been 2 weeks and I'm not sure much of it ties together.
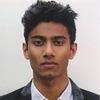
Samuel Kleos
Front End Web Development Techdegree Student 12,719 PointsReview ChatGPT Response:
No, the use of Promises did not involve a new Web API specifically for handling requests. Promises are a language feature of JavaScript that provide a standard way to handle asynchronous operations, including network requests.
Instead of handling callbacks with XMLHttpRequest
objects or other similar methods, Promises allow you to work with asynchronous operations in a more organized and readable way. You can create a Promise to represent an asynchronous operation, and then use the then
method to handle the result when it's ready.
Samuel Kleos
Front End Web Development Techdegree Student 12,719 PointsSamuel Kleos
Front End Web Development Techdegree Student 12,719 PointsReview ChatGPT Response:
Yes, you are correct in identifying the evolution of error-catching mechanisms in the history of JavaScript asynchronous requests.
In the past, with the use of XMLHttpRequest objects, network errors were typically checked using the readyState property, which would be set to a value of 4 when the request had completed. Meanwhile, response status problems could be caught using the status property of the XMLHttpRequest object.
Later on, with the use of Promises, the onload and onerror events were used to catch network errors and response errors were still checked using the status property.
Now, with the fetch API, async/await, and the Response object, network errors are caught by checking if the fetch promise is rejected, and response errors can be caught by checking the ok property of the Response object.
Your code examples illustrate these changes well, and it's good to see that you are keeping track of the evolution of these technologies!