Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial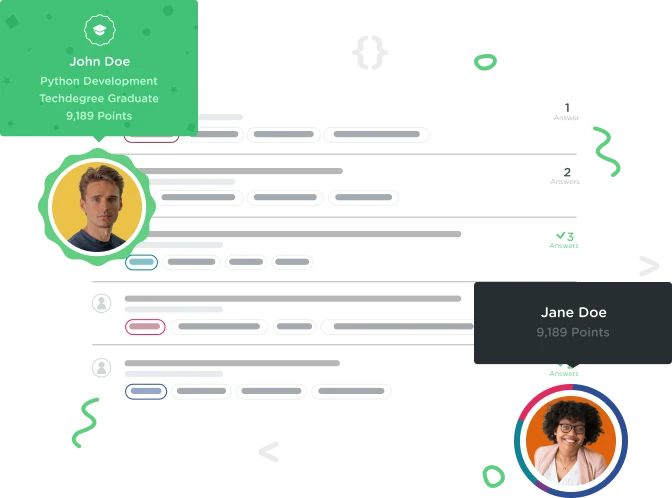

Killeon Patterson
18,425 PointsAccessing my MongDB Database
Hello, I'm trying to access my MongoDB database using
import { MongoClient, ServerApiVersion } from "mongodb";
const URI = process.env.ATLAS_URI || "";
const client = new MongoClient(URI, {
serverApi: {
version: ServerApiVersion.v1,
strict: true,
deprecationErrors: true,
},
});
try {
// Connect the client to the server
await client.connect();
// Send a ping to confirm a successful connection
await client.db("admin").command({ ping: 1 });
console.log("Pinged your deployment. You successfully connected to MongoDB!");
} catch (err) {
console.error(err);
}
console.log(db);
let db = client.db("test");
export default db;
I'm successfully connecting to MongoDB, but not my collections. The database's name is "test" and the collection name is "menus". How can I access the collections on my MongoDb?
4 Answers
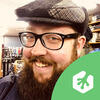
Rohald van Merode
Treehouse StaffHey Killeon Patterson 👋
Thanks for providing a link to your repo, that definitely gives some more insight into how things are setup.
Let's start of with the backend issue
When you say "there's no collection", what do you mean exactly when saying that? What is that console.log statement you've added logging on your end? Is it logging something like undefined
? Or is it a Collection
object? If it's the latter and your database is setup correctly (database and collection names are matching) you should be good.
There are however a couple of issues in your backend that may cause your issues
First off on line 23/26 of connection.js
you are exporting the collection instead of the database object. Given how you're currently trying to access specific collections in your routes the easiest would be to simply return the db object instead. This way you can also access other collections through the db in the future instead of creating and exporting another collection.
// let db = client.db("test").collection("menus");
let db = client.db("test")
Next up in your routes/record.js
file there's a syntax issue on line 17 as you're currently passing an argument to the find method. You'll instead want to not pass any arguments and call the toArray()
on what is being returned from find:
// let results = await collection.find({}.toArray());
let results = await collection.find().toArray();
After those two changes you should be able to see the results when visiting http://localhost:5050/record
Which brings me to the client side code.
As I suggested in my last comment, here is no /menu
endpoint available in your API. Since there's is nothing to be found at localhost:5050/menu
you'll get a 404 response.
With how your API is currently setup on line 10 of your server.js
file you're setting up the records
router to be at the /record
path. You'll either want to adjust this to be /menu
or adjust the endpoint you're trying to fetch from on line 49 of your RecordList
component to match the route: http://localhost:5050/record/
After this change you should get a error occurred: OK
message logged to the console. This is because of how your conditional is setup with !response.ok
. When using Axios there is no ok
property available, so you'll want to adjust that conditional to maybe be something like response.status !== 200
instead.
I hope this will help to get you going again! 😄
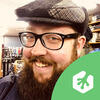
Rohald van Merode
Treehouse StaffHey Killeon Patterson 👋
You can access your collections by using the collection
method that is available on the db
object.
const menus = db.collection('menus');
Hope this helps!

Killeon Patterson
18,425 PointsHello, Thank you for your reply. I get a successful connection to Mongo, but I still don't have access to the collections. I tried console.logging the info out and it appears to have every other piece of information that isn't the collection. I get a 404 messages for my axios calls to the server for the resources.
export default function RecordList() {
const [menus, setRecords] = useState([]);
// This method fetches the records from the database.
useEffect(() => {
async function getRecords() {
const response = await axios.get('http://localhost:5050/menu/')
if (!response.ok) {
const message = `An error occurred: ${response.statusText}`;
console.error(message);
return;
}
const menus = await response.json();
setRecords(menus);
}
getRecords();
return;
}, [menus.length]);
// This method will delete a record
async function deleteRecord(id) {
await axios.get(`http://localhost:5050/menus/${id}`, {
method: "DELETE",
});
const newRecords = menus.filter((el) => el._id !== id);
setRecords(newRecords);
}
// This method will map out the records on the table
function recordList() {
return menus.map((menu) => {
return (
<Record
menu={menu}
deleteRecord={() => deleteRecord(menu._id)}
key={menu._id}
/>
);
});
}
Do you have any suggestions? Thank you
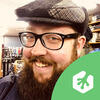
Rohald van Merode
Treehouse StaffHey Killeon Patterson,
It's hard to tell what is happening with only seeing these two snippets and would need more information/context to tell for sure what is causing these issues. Are you getting any errors when trying to access the collection? How are you trying to retrieve the data after the line I suggested above? I found a page on Retrieving Data in the MongoDB documentation that might be useful.
As for the 404 in the frontend it sounds like the /menu
route doesn't exist in your API. How is this route set up?

Killeon Patterson
18,425 PointsHello Richard,
This is the GitHub of the project "https://github.com/Kilosince/updateohstore/tree/main" (I removed my mongostring from the ATLAS_URI)
I believe the underlying issue is the 404 message I'm getting from the route in lines 49 and 64.
For context I made a project awhile ago, apparently before a lot of packages and other dependencies had become deprecated. So, I'm attempting to remake this project "https://github.com/Kilosince/PointOfSale/tree/master" with newer processes. For instance, I had not previously work with vite.
The MongoDB is the same one I've been using for some time. I've console.log(client.db("test").collection("menus")). The connection is made but there's no collection that I can see. Thank you for your time.
Killeon Patterson
18,425 PointsKilleon Patterson
18,425 PointsRohald, Thank you 😄.