Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial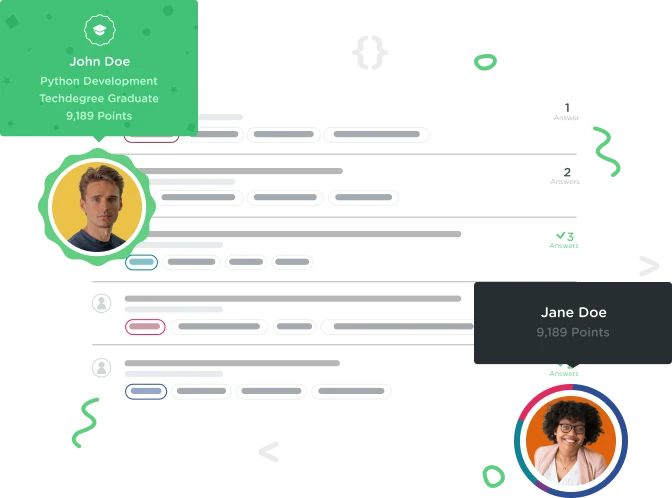

Kevin Daniel Pantasdo
6,369 PointsApp did not ask permission for likes
After deleting the app in the simulator and running the code again, the app requires me to sign in. So far so good.
However, after singing in, app did not ask permission to like.. Thus even after including the options:@{@"scope" : @[@"likes"]} in the simpleAuth, status code is still 400. Does that mean that there is something wrong with my code regarding the syntax?
Most likely if error code is 400, it has to be in the THPhotoCell implementation right? But I have proofread the code letter by letter and I'm pretty sure there's nothing wrong.

Andrew Ware
3,594 PointsSee this post about Instagram's new API endpoints policy. https://teamtreehouse.com/forum/instagram-api-changes
Basically, they are no longer allowing you to make POST requests for likes without applying and getting approved. I would suggest just observing the material covered in these videos concerning HTTP requests via endpoints and not worrying too much about the fact that your app isn't ACTUALLY liking the photos on Instagram's side.
Kevin Daniel Pantasdo
6,369 PointsKevin Daniel Pantasdo
6,369 PointsAs I have mentioned, status code is 400 and instagram won't ask permission to like...
This is my code:
PhotosViewController.m
PhotoCell.m