Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial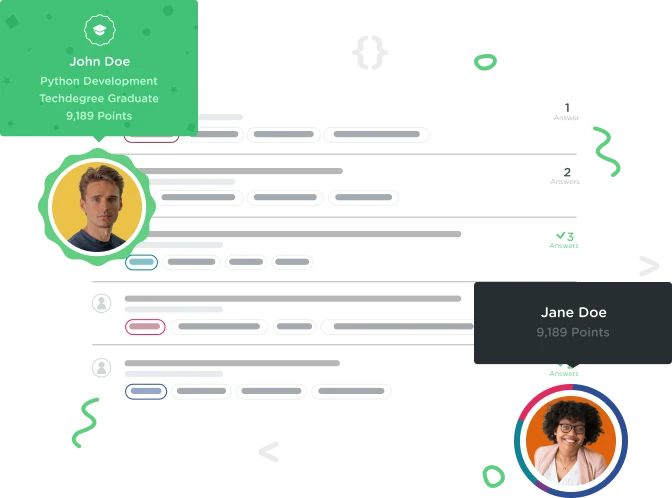
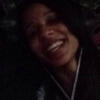
Fradely Dilone
24,037 Pointsconst wikiUrl = 'https://en.wikipedia.org/api/rest_v1/page/summary/'; Nothing is working
const wikiUrl = 'https://en.wikipedia.org/api/rest_v1/page/summary/';
4 Answers

erino
6,485 PointsThe current problem appears to be that api-open-notify and Wikipedia disagree on how to spell the name of one of the Russian astronauts, so the two data sources aren't matching up. Wikipedia is spelling the name as Anatoli Ivanishin, while api-open-notify is spelling it Anatoly Ivanishin, with the difference being the last letter of the first name. I don't know how to make the code account for this issue, but maybe someone else does.
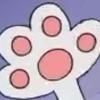
Shiyu Hong
12,681 PointsThe following code works for me. Hope it helps. Adding a bit of error handling so that information for the rest of the astronauts can be displayed.
First, in getProfiles function, I changed Promise.all() to Promise.allSettled(). Promise.all() needs all promises to be fulfilled, while Promise.allSettled() can have rejects. See MDN reference here: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise/all
Second, in generateHTML function, I added a error handling to ensure person.value.type === "standard", otherwise don't do anything for that iteration. This ensures any names not found in Wikipedia can be skipped.
const astrosUrl = 'http://api.open-notify.org/astros.json';
const wikiUrl = 'https://en.wikipedia.org/api/rest_v1/page/summary/';
const peopleList = document.getElementById('people');
const btn = document.querySelector('button');
function getProfiles(json) {
const profiles = json.people.map( person => {
return fetch(wikiUrl + person.name)
.then (response => response.json())
.catch (err => console.log('Error fetching Wikipedia:', err));
});
return Promise.allSettled(profiles);
}
function generateHTML(data) {
data.map( person => {
const section = document.createElement('section');
peopleList.appendChild(section);
if (person.value.type === "standard") {
section.innerHTML = `
<img src=${person.value.thumbnail.source}>
<h2>${person.value.title}</h2>
<p>${person.value.description}</p>
<p>${person.value.extract}</p>
`;
}
})
}
btn.addEventListener('click', (event) => {
event.target.textContent = 'Loading...';
fetch(astrosUrl)
.then (response => response.json())
.then(getProfiles)
.then(generateHTML)
.catch( err => {
peopleList.innerHTML = '<h3>Something went wrong</h3>';
console.log(err);
})
.finally(() => event.target.remove());
});
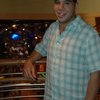
Nick Davies
Full Stack JavaScript Techdegree Student 13,040 PointsShiyu Hong's code worked, but the last astronaut isn't showing up. i would love to get that to work :)
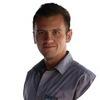
Florin Dumitrasc
7,771 PointsThat worked for me too, but only displaying the pictures and the sections. The text is all "undefined".
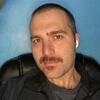
Jonathan Drake
Full Stack JavaScript Techdegree Student 11,668 PointsSame issue...current problem is Sergey Korsakov comes back on the JSON data as "Sergey Korsakov." The period at the end of his name screws up the url production in getProfiles. I resolved it specifically by using an if statement to catch the period and change the value of person.name to the name without the period.
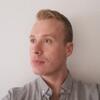
Michael Kristensen
Full Stack JavaScript Techdegree Graduate 26,251 PointsThis appears to be a common problem in this course, and the AJAX request returns with a 404 error (Source not found), because it is looking for the wrong name. Sadly, the only real way of fixing this in the code, is to implement a manual fix;
if (person.name != "yulia_pereslid"){
return getJSON(wikiUrl + person.name);
} else {
return getJSON(wikiUrl + "yulia_peresild");
}
- Of course, by now, this name error is no longer relevant, as she isn't on the list anymore, but another set of names with problems of the same nature has most likely taken over.
Phil Wright
3,654 PointsPhil Wright
3,654 PointsHi Fradely.
How is it not working? That assigns correctly, see output from console below:
What are you trying to do, and what are you expecting to happen?
Phil