Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial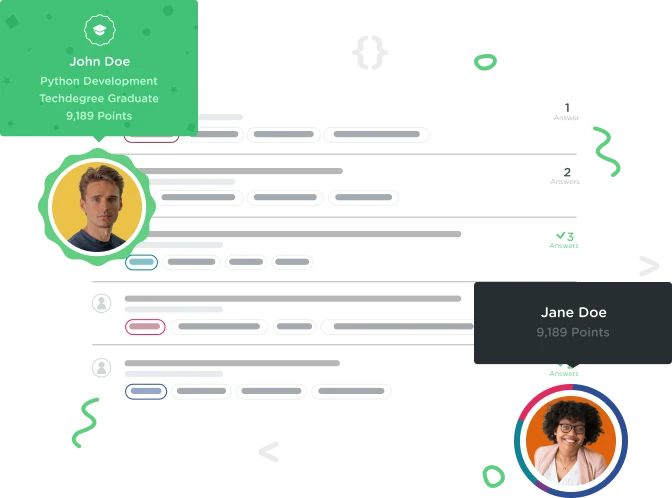

Oksana Kusyo
10,180 PointsCouldn't get the code to run while working along this Loops video. Please let me know what I am doing wrong.
let html = '';
let red;
let green;
let blue;
let randomRGB;
const randomValue = () => Math.floor(Math.random() * 256);
function randomRGB(value) {
const color = `rgb( ${value()}, ${value()}, ${value()} )`;
return color;
}
for (let i = 1; i <=10; i++ ) {
red = randomValue();
green = randomValue();
blue = randomValue();
randomRGB = `rgb( ${red}, ${green}, ${blue} )`;
html += `<div style="background-color: ${randomRGB(randomValue)}">${i}</div>`;
}
document.querySelector('main').innerHTML = html;
Mod edit: Added markdown formatting for code readability. Check out the "Markdown Cheatsheet" linked below the comment box for tips on how to format your code for the forums.
1 Answer

John Johnson
11,789 PointsYour code looks like it's missing string interpolation backtics in a few places. Remember, anywhere you are using ${}, it must be wrapped in backticks.
ex.
`rgb( ${value}, ${value}, ${value} )`;
Also, some of the code that got refactored in this lesson is still included in your script here. I've commented the things that should be removed.
I've just changed the spacing for readability
let html = '';
let red;
let green;
let blue;
// you can't have a function called randomRGB if you've already declared it as a variable
// let randomRGB;
const randomValue = () => Math.floor(Math.random() * 256);
function randomRGB(value) {
// for ${} to work, you need to use string interpolation (wrap your string in backticks)
const color = `rgb( ${value()}, ${value()}, ${value()} )`;
return color;
}
for (let i = 1; i <=10; i++ ) {
// randomValue has been updated to automatically generate the three random numbers for red, green and blue
// these bits of code were used earlier in this lesson, but aren't in use here, so you can remove all of these
// red = randomValue();
// green = randomValue();
// blue = randomValue();
// randomRGB = rgb( ${red}, ${green}, ${blue} );
// added backticks here as well
html += `<div style="background-color: ${randomRGB(randomValue)}">${i}</div>`;
}
document.querySelector('main').innerHTML = html;
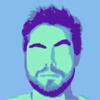
Cameron Childres
11,817 PointsGreat answer, thanks John! All I have to add is that the backticks are present but were obscured by the forum's markdown rules. I've gone ahead and edited the original post to add code formatting so they show up appropriately.
For anyone else reading this that may be unfamiliar, be sure to check out the "Markdown Cheatsheet" linked below the comment box for examples of how to make your code display properly in the community.
RenΓ© Vik
2,621 PointsRenΓ© Vik
2,621 PointsYour code contains parts of an old solution in the video in addition to the final solution. This causes you to attempt to use the same variable name for different purposes: You have declared the variable name 'randomRGB' on line 5 (from old solution), but you use the same name to declare a function on line 9 (from final solution). On line 19 it shows up as a variable again (from old solution), and on line 20 it is used as a function call (from final solution).
If you comment out line 5 and 19 (add // at the start of the lines) your code works!! Though you will have some unused code hanging around (refering to the red, green and blue variable occurances).