Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial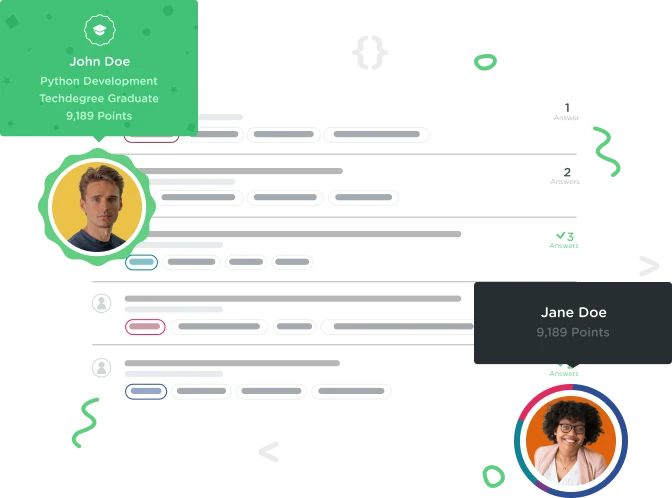
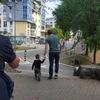
Aleksa Milovanovic
2,470 Pointserror: extra argument 'y' in call super.init(x: x, y: y)
class SuperEnemy: Enemy {
let isSuper: Bool = true
override init(x: Int, y: Int) {
super.init(x: x, y: y) { //I have problem with this line of code
self.life = 50
}
}
}
Xavier D
Courses Plus Student 5,840 PointsXavier D
Courses Plus Student 5,840 PointsHi Aleksa,
I believe the reason why you are have that error is because of the parentheses after the call to Enemy's init method within the SuperEnemy's init method. You are getting that error because those parentheses (as well as anything within it...) are not required in the call.
Also, those parentheses contains:
self.life = 50
I was wondering why is that line of code is enclosed in parentheses after the call to the base class' init method. When you use the "self" keyword from within a class, you are referring to an instance of the class itself. Thus, when you create an instance of SuperEnemy, you basically implied that its instance will start of with a life of 50 by default.
However, if that was your intention, it will not work because you did not declare that your subclass has a property to set to that 50 Int value. I only see that a Bool property was declared, and that constant was defined to value of true. Basically, before you can set a life property, you first have to create/declare it as a property belonging to the class, and right now your subclass doesn't think life exist. Now you can declare it to have a default value before initialization with defining it this way at the beginning of the class:
let life = 50
Or you can also defer assigning a value until initialization...but then you'll have to declare the property to have no value this way at the top of the class:
let life: Int
If you do it this way, you'll need to create an init() for it. I'm guessing you wanted to do it this way; however, you'll need to remove those parentheses and it's line of code needs to be stated prior to the super class's init method(not after), being that when overriding, all of the class' non-optional properties that do not have default values must eventually contain values prior to initialization and the call to its superclass' init method. Thus, your subclass' override() init must look like:
Again...if you do it this way, don't forget to declare the life property at the beginning of the subclass.
XD