Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial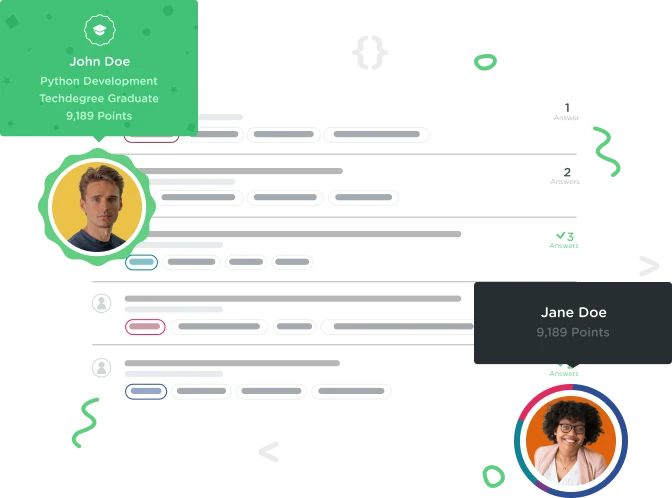

Lewis Fierle
392 Points.format
This function .format keeps showing up in the code and Im a bit confused as to the when to use, and what exactly it does. It appears to relate to inputs but im just a little unsure about when I might use .format and what exactly its doing in my code.
Thanks!
3 Answers
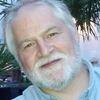
Jeff Muday
Treehouse Moderator 28,717 PointsThe .format()
method of a string is very useful for creating strings that can be formed from a template. These can be used anywhere you feel is appropriate.
The .format()
method has some distinct advantages over simple concatenation of strings especially in complex scenarios where you might want to name the parameters or reuse them multiple times.
Here is a nice explanation:
https://www.w3schools.com/python/ref_string_format.asp
message = "My name is {} {}.".format('Bart','Simpson')
print(message)
message = "My name is Mr. {last}-- Mr. {first} {last}. But you can call me {first}.".format(first='Bart', last='Simpson')
print(message)
This will return
My name is Bart Simpson.
My name is Mr. Simpson-- Mr. Bart Simpson. But you can call me Bart.
Suppose we have a madlib
madlib = """
==========Storytime========
This is a story of a famous {animal}. This {animal}'s name is {name}.
Not only was this a {adjective} {animal}, but this {animal} could perform {skill}!
One day {name} the {animal} made a friend named {friend}.
{friend} was amazed that {name} the {animal} was so {adjective} at {skill}.
{name} served {friend} some {dessert} that proved to be {adjective}.
It was a {adjective} day afterall!
The end.
"""
print(madlib.format(animal='Unicorn', name='Susan',
adjective='dazzling', skill='magic',friend='Eggbert', dessert="chocolate ice-cream"))
print(madlib.format(animal='Vampire',
name='Doctor Oddball', adjective='bizzare', skill='Hip-hop dancing',
friend='Waldo the Clown', dessert="blueberry pancakes"))
the output
==========Storytime========
This is a story of a famous Unicorn. This Unicorn's name is Susan.
Not only was this a dazzling Unicorn, but this Unicorn could perform magic!
One day Susan the Unicorn made a friend named Eggbert.
Eggbert was amazed that Susan the Unicorn was so dazzling at magic.
Susan served Eggbert some chocolate ice-cream that proved to be dazzling.
It was a dazzling day afterall!
The end.
==========Storytime========
This is a story of a famous Vampire. This Vampire's name is Doctor Oddball.
Not only was this a bizzare Vampire, but this Vampire could perform Hip-hop dancing!
One day Doctor Oddball the Vampire made a friend named Waldo the Clown.
Waldo the Clown was amazed that Doctor Oddball the Vampire was so bizzare at Hip-hop dancing.
Doctor Oddball served Waldo the Clown some blueberry pancakes that proved to be bizzare.
It was a bizzare day afterall!
The end.
But .format()
is also great for formatting numbers. There are more than 20 different types of formatting built in. This is a very compelling reason to use it.
import math
print("A tribute to Pi, a very important number")
print(math.pi)
print("--Pi rounding--")
print("pi to 2 decimal places is {:.2f}".format(math.pi))
print("pi to 4 decimal places is {:.4f}".format(math.pi))
print("--In science/engineering--")
print("pi in scientific format is {:E}".format(math.pi))
output
A tribute to Pi, a very important number
3.141592653589793
--Pi rounding--
pi to 2 decimal places is 3.14
pi to 4 decimal places is 3.1416
--In science/engineering--
pi in scientific format is 3.141593E+00
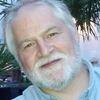
Jeff Muday
Treehouse Moderator 28,717 PointsJon,
Very cool way to use the concept!
Cheers,
Jeff

Lewis Fierle
392 PointsThanks that cleared some things up!
Jon Koch
1,448 PointsJon Koch
1,448 PointsThanks for that :)
Just for fun I made it take input:
madlib = """This is a story of a famous {animal}. This {animal}'s name is {name}. Not only was this a {adjective} {animal}, but this {animal} could perform {skill}! One day {name} the {animal} made a friend named {friend}. {friend} was amazed that {name} the {animal} was so {adjective} at {skill}. {name} served {friend} some {dessert} that proved to be {adjective}. It was a {adjective} day afterall! The end. """ print(madlib.format(animal=input("animal "), name=input("name "), adjective=input("adjective "), skill=input("skill "),friend=input("friend "), dessert=input("dessert ")))