Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial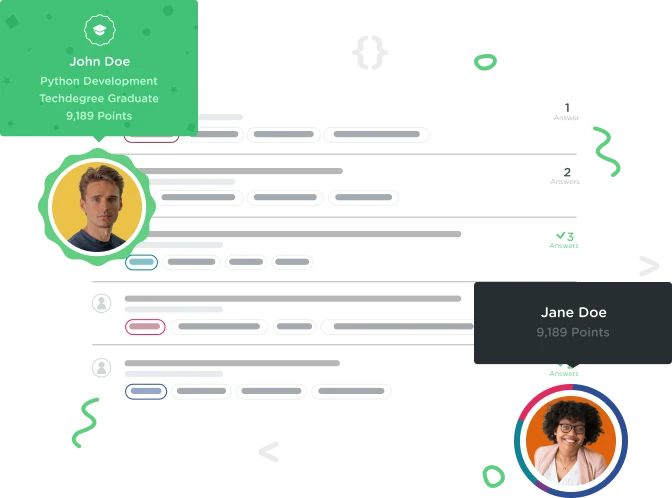

Bartosz Ziółkowski
352 PointsGetter method - what is it actually? what is it used for?
Getter method - I do not understant it.
1 Answer

adrian miranda
13,561 PointsIn an object, you will normally store one or more variables. Now you could allow users of your object to directly access those variables. However, it is usually best to only allow the user to access the variables through getters and setters.
So instead of this:
public class rectangle {
public int height;
public int width;
}
which would allow anyone to directly pull the value of height and width out of the object, we might prefer to do:
public class rectangle {
private int height, width;
public int getHeight() {
return height;
}
.... /* other stuff */
}
Now, people can't directly access height width, because they are private. Instead they could call getHeight() or getWidth()---------------------------------------------------------------------.
In the simplest cases, these can seem pretty silly. Where these become more valuable is when you aren't always doing the same basic return whatever it is.
For example, you might want to only give access to some of the variables, but not the others.
Or you might have variables that you want to perform a calculation to derive the value. For example, suppose you originally had an area
variable in the object. Later, you decided to instead calculate as height * width
. Your user wouldn't know anything about whether you saved it or calculated it, either way they would call getArea()
.
Again, this is a pretty simple example, but in more complex examples, you may have good reason to keep the user for knowing about how the class works. Maybe you want to shield details from the user, so they just don't have to think about it. Or maybe you want to be able to change how the class works, without everyone who uses it needing to change the way they use it. Using getters and setters is a simple but useful way of moving your code in that direction.