Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial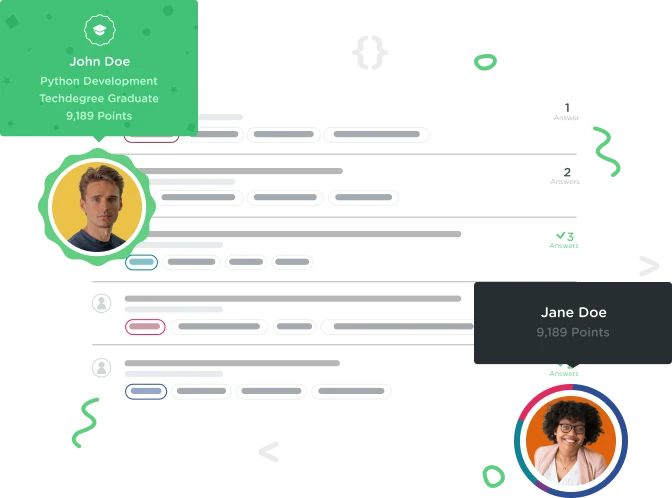
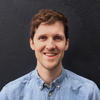
Dylan Macnab
24,861 PointsGetting the wrong order of invitees and "Unhandled promise rejection" error
I'm having two issues on this step.
The order of the invitees are all out of sync and some are missing names or have multiple names. I'm thinking it has to do with the forEach method because it doesn't happen when I manually add names using the
addInvitee()
functions on their own.I'm getting this error in my console from the removeInvitee() function:
(node:19360) UnhandledPromiseRejectionWarning: Unhandled promise rejection (rejection id: 1): NoSuchElementError: no such element: Unable to locate element: {"method":"xpath","selector":"//span[text()="Dylan Macnab"]/../button[last()]"}
(Session info: chrome=66.0.3359.181)
(Driver info: chromedriver=2.38.552518 (183d19265345f54ce39cbb94cf81ba5f15905011),platform=Mac OS X 10.13.1 x86_64)
(node:19360) [DEP0018] DeprecationWarning: Unhandled promise rejections are deprecated. In the future, promise rejections that are not handled will terminate the Node.js process with a non-zero exit code.
I'm using node v8.8.1. Any help is appreciated, thanks!
const selenium = require("selenium-webdriver");
const By = selenium.By;
const driver = new selenium.Builder()
.forBrowser("chrome")
.build();
driver.get(process.env.URL);
const invitees = [
'Dylan Macnab',
'Shadd Anderson',
'George Aparece',
'Shadab Khan',
'Joseph Michael Casey',
'Jennifer Nordell',
'Faisal Albinali',
'Taron Foxworth',
'David Riesz',
'Maicej Torbus',
'Martin Luckett',
'Joel Bardsley',
'Reuben Varzea',
'Ken Alger',
'Amrit Pandey',
'Rafal Rudzinski',
'Brian Lynch',
'Lupe Camacho',
'Luke Fiji',
'Sean Christensen',
'Philip Graf',
'Mike Norman',
'Michael Hulet',
'Brent Suggs'
];
const locators = {
inviteeForm: By.id("registrar"),
inviteeNameField: By.css("#registrar input[name='name']"),
toggleNonRespondersVisibility: By.css(".main > div input"),
removeButtonForInvitee: invitee => By.xpath(`//span[text()="${invitee}"]/../button[last()]`)
}
function addInvitee(name) {
driver.findElement(locators.inviteeNameField).sendKeys(name);
driver.findElement(locators.inviteeForm).submit();
}
function removeInvitee(name) {
driver.findElement(locators.removeButtonForInvitee(name)).click();
}
function toggleNonRespondersVisibility() {
driver.findElement(locators.toggleNonRespondersVisibility).click();
}
invitees.forEach(addInvitee);
removeInvitee("Dylan Macnab");
3 Answers
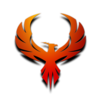
Brian Pedigo
26,783 PointsI was having the same problems as listed above. I was able to get the code working by changing the code in two places.
First I used regular concatenation isn't of template literals.
removeButtonForInvitee: invitee => By.xpath('//span[text()="' + invitee +'"]/../button[last()]')
Second, the html and css wasn't finished being built by the first function therefore, the call to removeInvitee() would fail. I fixed this by using the setTimeout function.
setTimeout(removeInvitee, 5000, "Shadd Anderson");
After that those changes it worked as show in the video.
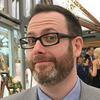
Simon McGuirk
2,673 PointsAlso having the same issue. It looks like the script is trying to identify the element before the list has completed being added hence not finding the element. I've been trying to workout how to make the script wait before trying to find the element but so far have not been successful. Hopefully @craigdennis can add this in a future revision to avoid people getting stuck at this point.

Natalia-Antonia Baluta
6,079 PointsI have the same problem, I'm really disappointed to see that nobody answered for almost one year. I think I'm just gonna skip.
Unsubscribed User
15,444 PointsUnsubscribed User
15,444 PointsHi,
Sorry I'm unable to answer the question, but I am also having a similar issue.
The order is correct, but I'm getting the UnhandledPromiseRejection, NoSuchElementError.
However, in devtools, the xpath locator works.
The error output and my code is below.
Error Output
Index.js
Been scratching my head for the last hour and can't see anything wrong with the code.
Many thanks, Berian