Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial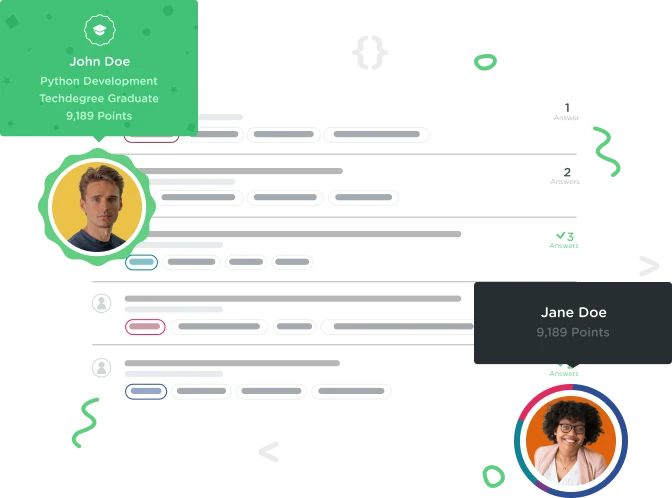

Peter Ayad
Front End Web Development Techdegree Graduate 20,766 PointsHello I am having trouble passing this assignment..says my tests are failing..any help would be appreciated..
public static List<Score> getFirstThreeNintendoScoresDeclaratively(List<Score> scores) { // TODO: Filter the scores stream to be of platform 'Nintendo Entertainment System' List<Score> nintendoScores = new ArrayList<>(); for (Score score : scores) { String platform = score.getPlatform().toLowerCase(); if (platform.contains("Nintendo")) { nintendoScores.add(score); if (nintendoScores.size() >= 3){ break; } } } // TODO: Limit it to 3 scores // TODO: Return a newly collected list using the collect method return Collections.emptyList(); }
Execution failed for task ':test'.
There were failing tests. See the report at: file:///C:/Users/Peter/IdeaProjects/lcc-java-fp-highscores-1.0.3-COLLECT/build/reports/tests/test/index.html
Task :test
com.teamtreehouse.challenges.highscores.MainTest > limitsCollectedResults FAILED java.lang.AssertionError: Ensure you are limiting the stream Expected: is <3> but: was <0> at org.hamcrest.MatcherAssert.assertThat(MatcherAssert.java:20) at com.teamtreehouse.challenges.highscores.MainTest.limitsCollectedResults(MainTest.java:62)
com.teamtreehouse.challenges.highscores.MainTest > collectedTheFirstThree FAILED java.lang.AssertionError: Make sure you are returning the correct scores Expected: ((a collection containing "FIRST" and a collection containing "SECOND" and a collection containing "THIRD") and not a collection containing "TOO_MANY") but: (a collection containing "FIRST" and a collection containing "SECOND" and a collection containing "THIRD") a collection containing "FIRST" at org.hamcrest.MatcherAssert.assertThat(MatcherAssert.java:20) at com.teamtreehouse.challenges.highscores.MainTest.collectedTheFirstThree(MainTest.java:84)
8 tests completed, 2 failed
Task :test FAILED
Execution failed for task ':test'.
There were failing tests. See the report at: file:///C:/Users/Peter/IdeaProjects/lcc-java-fp-highscores-1.0.3-COLLECT/build/reports/tests/test/index.html
7 Answers

saykin
9,835 PointsHey Peter, you're supposed to solve assignment Declaratively, as mentioned by Craig in the video. You have solved it Imperatively, I would advise you to re watch the video and see how Craig solves the specific problem in the video and apply that solution to this assignment by using stream().
While you can bypass the test system by solving it like you have, you won't be learning how to use streams properly.
public static List<Score> getFirstThreeNintendoScoresDeclaratively(List<Score> scores) {
// TODO: Filter the scores stream to be of platform 'Nintendo Entertainment System'
// TODO: Limit it to 3 scores
// TODO: Return a newly collected list using the collect method
List<Score> nintendoScores = new ArrayList<>();
for (Score score : scores) {
String platform = score.getPlatform().toLowerCase();
if (platform.contains("Nintendo")) {
nintendoScores.add(score);
if (nintendoScores.size() >= 3){
break;
}
}
}
return Collections.emptyList();
}
You have two errors here, the test is looking specifically for "Nintendo Entertainment System" (notice the upper case letters), but you are calling on .toLowerCase which will cause an error.
You are also returning Collections.emptyList() instead of the list you are storing the objects in nintendoScores.
Solving these two issues, will pass the test, but it isn't how Craig intends for you to solve this problem, he wants you to use streams to solve it.

Peter Ayad
Front End Web Development Techdegree Graduate 20,766 PointsHey saykin, thanks for the heads up, but im still alittle confused about how to type this code, not sure if im supposed to create a predicate of boolean type like in the video or just use the filter method:
private static boolean isNintendoScores(Score score) { String score = score.getNintendoScores().equals("Nintendo"); return score.contains("Nintendo"); }
public static List<Score> getFirstThreeNintendoScoresDeclaratively(List<Score> scores) { // TODO: Filter the scores stream to be of platform 'Nintendo Entertainment System' return scores.stream() .filter(Main::isNintendoScores) .limit(3)
// TODO: Limit it to 3 scores
// TODO: Return a newly collected list using the collect method
.collect(Collectors.toList());
}
or if its supposed to be somewhat like this:
public static List<Score> getFirstThreeNintendoScoresDeclaratively(List<Score> scores) { // TODO: Filter the scores stream to be of platform 'Nintendo Entertainment System' scores.stream() .filter(score -> score.getNintendoScores().equals("Nintendo")) .filter(score -> score.getPlatform().equals("Nintendo")) .limit(3)
// TODO: Limit it to 3 scores
// TODO: Return a newly collected list using the collect method
.collect(Collectors.toList());
}
Any help would be greatly appreciated.

saykin
9,835 PointsYour first solution is the closest.
In the boolean method, you want to get the platform name and check if it equals "Nintendo Entertainment System". Right now you are looking for the scores.
In the getFirstThreeNintendoScoresDeclaratively() method, all you're missing is to add your data to a list. You have already written down the code.
.collect(Collectors.toList());
You just need to add it to the end after .limit(3) If you fix your boolean method and add the results to a list, the tests should pass.

Peter Ayad
Front End Web Development Techdegree Graduate 20,766 PointsHey saykin, im still having trouble im not sure if im typing the boolean method correctly,, the eror keeps telling me that (name), (score), (platform), etc is already defined??, so im not sure how to write it, im alil confused on this.. Any help would be appreciated..
private static boolean isNintendoScores(String name) { String platform = name.getPlatform().equals("Nintendo"); return platform.contains("Nintendo"); }
public static List<Score> getFirstThreeNintendoScoresDeclaratively(List<Score> scores) { // TODO: Filter the scores stream to be of platform 'Nintendo Entertainment System' return scores.stream() .filter(Main::isNintendoScores) .limit(3) .collect(Collectors.toList()); // TODO: Limit it to 3 scores // TODO: Return a newly collected list using the collect method
}
C:\Users\Peter\IdeaProjects\lcc-java-fp-highscores-1.0.3-COLLECT\src\main\java\com\teamtreehouse\challenges\highscores\Main.java:40: error: cannot find symbol String platform = name.getPlatform().equals("Nintendo"); ^ symbol: method getPlatform() location: variable name of type String

Peter Ayad
Front End Web Development Techdegree Graduate 20,766 PointsHello, im still not able to pass this test, and im confused about what i have to do, im not sure that my boolean method is correct, but ive been trying for the days to work the problem, with no luck..please can anyone help, i know it is something that's small, that im not noticing..
private static boolean isNintendo(String name) { String title = name.getTitle().equals("Nintendo"); return platform.getTitle; }
public static List<Score> getFirstThreeNintendoScoresDeclaratively(List<Score> scores) { // TODO: Filter the scores stream to be of platform 'Nintendo Entertainment System' return scores.stream() .filter(Main::isNintendo) .limit(3) .collect(Collectors.toList()); // TODO: Limit it to 3 scores // TODO: Return a newly collected list using the collect method
}
private static boolean isNintendo(Score score) { String Title = score.getTitle().equals("Nintendo"); return title.contains("Nintendo"); }
public static List<Score> getFirstThreeNintendoScoresDeclaratively(List<Score> scores) { // TODO: Filter the scores stream to be of platform 'Nintendo Entertainment System' return scores.stream() .filter(Main::isNintendo) .limit(3) .collect(Collectors.toList()); // TODO: Limit it to 3 scores // TODO: Return a newly collected list using the collect method
} im getting errors left and right, keeps telling me it cannot locate .getTitle, so im not sure whats going on, but if someone can please help, I would appreciate it..

Peter Ayad
Front End Web Development Techdegree Graduate 20,766 PointsHello anyone please, honestly I am getting a little discouraged about this project, ive been on this for more than a week, and i have not been able to figure out what is wrong with my boolean code. I understand that i have to find the platform name and see if it equals nintendo, but it keeps giving me an error("Cannot find symbol .getPlatform()"), or anything that I write in the body of the boolean method, just if someone can guide me in the right direction, I would be truly grateful..
private static boolean isNintendo(String platform) { String name = platform.getPlatform().equals("Nintendo"); return platform.contains("Nintendo"); }
public static List<Score> getFirstThreeNintendoScoresDeclaratively(List<Score> scores) { // TODO: Filter the scores stream to be of platform 'Nintendo Entertainment System' return scores.stream() .filter(Main::isNintendo) .limit(3) .collect(Collectors.toList()); // TODO: Limit it to 3 scores // TODO: Return a newly collected list using the collect method
}
C:\Users\Peter\IdeaProjects\lcc-java-fp-highscores-1.0.3-COLLECT\src\main\java\com\teamtreehouse\challenges\highscores\Main.java:40: error: cannot find symbol String name = platform.getPlatform().equals("Nintendo"); ^ symbol: method getPlatform() location: variable platform of type String
C:\Users\Peter\IdeaProjects\lcc-java-fp-highscores-1.0.3-COLLECT\src\main\java\com\teamtreehouse\challenges\highscores\Main.java:48: error: incompatible types: invalid method reference .filter(Main::isNintendo) ^ incompatible types: Score cannot be converted to String
C:\Users\Peter\IdeaProjects\lcc-java-fp-highscores-1.0.3-COLLECT\src\main\java\com\teamtreehouse\challenges\highscores\Main.java:50: error: cannot find symbol .collect(Collectors.toList()); ^ symbol: variable Collectors location: class Main
Can anyone please help, I don't know if im missing something, or if im writing something out wrong..Please help. Thank you
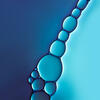
Romero M
Full Stack JavaScript Techdegree Student 149 PointsI put your code into my editor and compared your solution to mine.
As a reminder, here is your code
private static boolean isNintendo(String name) {
String title = name.getTitle().equals("Nintendo");
return platform.getTitle;
}
private static boolean isNintendo(String name)
Here you are defining your own parameters. You need to use the parameters that have already been defined which in this case is Score and score because it's asking us to filter the scores stream to be of platform "Nintendo Entertainment System".
String title = name.getTitle().equals("Nintendo");
The method in here should be .getPlatform instead of .getTitle() because .getPlatform has already been defined for us.
title is not a perfect name for this string. I would perhaps suggest using "system". For name.getTitle() you cannot retrieve any information from name because there's no data inside of it.
Spoiler❗️
private static boolean isNintendo(String name) {
String title = name.getTitle().equals("Nintendo");
return platform.getTitle;
}