Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial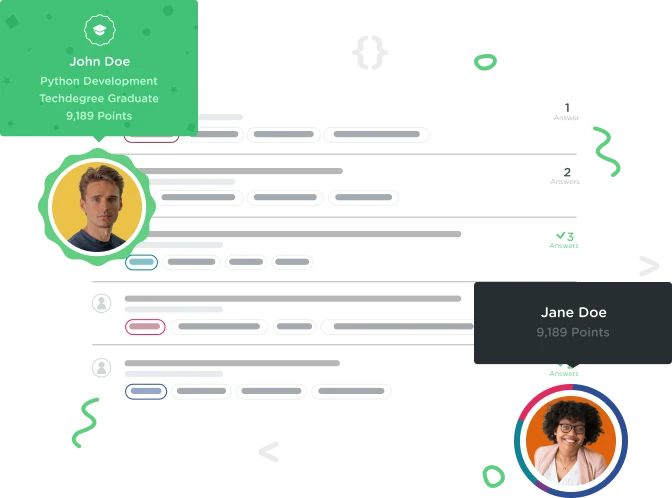

theo Vog
2,289 PointsHelp on how to override the function
Hello,
I don't know what's the problem with my code, can someone EXPLAIN me what's wrong ? I just want to understand :-)
Thanks !
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
class Doctor: Person {
override init(firstName: String, lastName: String) {
super.init(firstName: firstName, lastName: lastName)
super.lastName = lastName
}
override func fullName() -> String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Sam", lastName: "Smith")
2 Answers

theo Vog
2,289 PointsAllright! I just found the solution by my own.
But I don't understand everything. Why I don't need to write the initialization here ? Can someone provide me with super clear explanations ?
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
class Doctor: Person {
override func fullName() -> String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Sam", lastName: "Smith")
someDoctor.fullName()
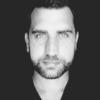
Jhoan Arango
14,575 PointsHello,
The reason why you do not have to write your own initializer it's because "Doctor" is inheriting the initializer from it's super class "Person". Not only it's inheriting the initializer, but all of the properties. By overriding the method, you are now creating your own Implementation of the method, meaning that you will not be directly using the inherited method, but your own.
If you had added an extra property to "Doctor", then you may add a new initializer to it.
I hope this helps you clear a bit. If you need a better explanation please let me know.
Good luck