Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial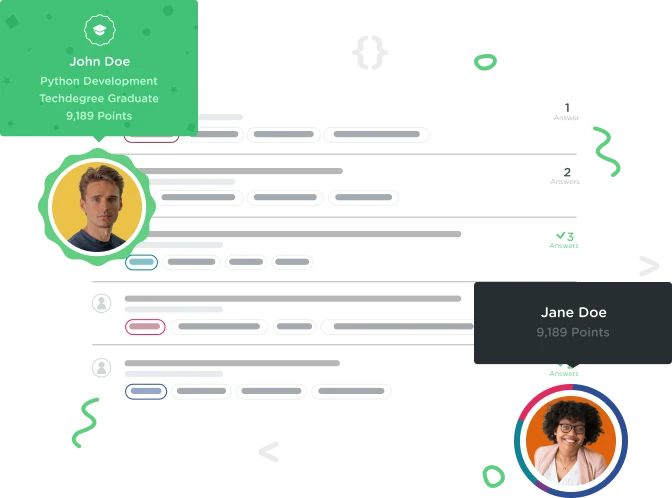

Lee Haney
395 PointsHere is the code for this video
CourseIdea.java
package com.teamtreehouse.courses.model;
public class CourseIdea {
private String title;
private String creator;
public CourseIdea(String title, String creator) {
this.title = title;
this.creator = creator;
}
public String getTitle() {
return title;
}
public String getCreator() {
return creator;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CourseIdea that = (CourseIdea) o;
if (title != null ? !title.equals(that.title) : that.title != null) return false;
return creator != null ? creator.equals(that.creator) : that.creator == null;
}
@Override
public int hashCode() {
int result = title != null ? title.hashCode() : 0;
result = 31 * result + (creator != null ? creator.hashCode() : 0);
return result;
}
}
CourseIdeaDAO.java
package com.teamtreehouse.courses.model;
import java.util.List;
import java.util.ArrayList;
public interface CourseIdeaDAO {
boolean add(CourseIdea idea);
List<CourseIdea> findAll();
}
SimpleCourseIdeaDAO.java
package com.teamtreehouse.courses.model;
import java.util.ArrayList;
import java.util.List;
public class SimpleCourseIdeaDAO implements CourseIdeaDAO {
private List<CourseIdea> ideas;
public SimpleCourseIdeaDAO() {
ideas = new ArrayList<>();
}
@Override
public boolean add(CourseIdea idea) {
return ideas.add(idea);
}
@Override
public List<CourseIdea> findAll() {
return new ArrayList<>(ideas);
}
}
Main.java
package com.teamtreehouse.courses;
import com.teamtreehouse.courses.model.CourseIdea;
import com.teamtreehouse.courses.model.CourseIdeaDAO;
import com.teamtreehouse.courses.model.SimpleCourseIdeaDAO;
import java.util.HashMap;
import java.util.Map;
import spark.ModelAndView;
import spark.template.handlebars.HandlebarsTemplateEngine;
import static spark.Spark.*;
public class Main {
public static void main(String[] args) {
CourseIdeaDAO dao = new SimpleCourseIdeaDAO();
// show something or "get" something.
get("/", (req, res) -> {
Map<String, String> model = new HashMap<String, String>();
model.put("username", req.cookie("username"));
return new ModelAndView(model, "index.hbs");
}, new HandlebarsTemplateEngine());
// create something
post("/sign-in", (req, res) -> {
Map<String, String> model = new HashMap<>();
String username = req.queryParams("username");
// set the cookie
res.cookie("username", username);
model.put("username", username);
return new ModelAndView(model, "sign-in.hbs");
}, new HandlebarsTemplateEngine());
}
}
Jeremy Dyck
12,013 PointsJeremy Dyck
12,013 PointsThank you!