Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial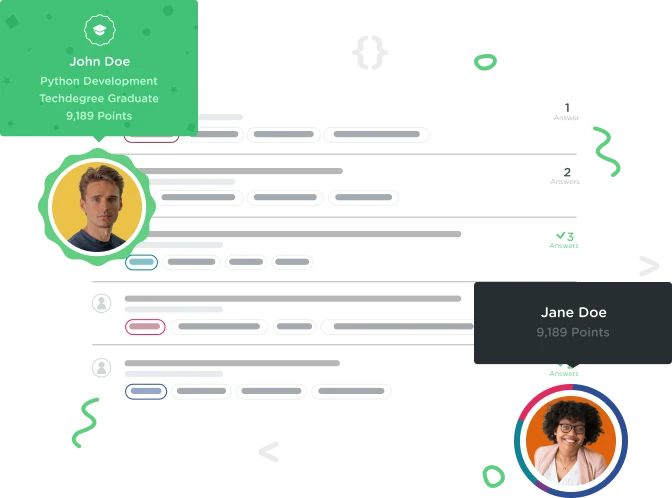

Lana Wong
3,968 PointsI'm confused on the HINT
INSTRUCTIONS:
In the editor, you have a struct named Parser whose job is to deconstruct information from the web. The incoming data can be nil, keys may not exist, and values might be nil as well. In this task, complete the body of the parse function. If the data is nil, throw the EmptyDictionary error. If the key "someKey" doesn't exist throw the InvalidKey error. HINT: To get a list of keys in a dictionary you can use the keys property which returns an array. Use the contains method on it to check if the value exists in the array.
I am confused on what the HINT is telling me.
enum ParserError: Error {
case emptyDictionary
case invalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let someKey = data["someKey"] else {
throw ParserError.invalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
1 Answer
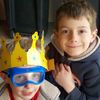
Chris Stromberg
Courses Plus Student 13,389 PointsFirst check to make sure data is not nil, if it is, throw the PaserError so you can exit the function without crashing.
guard let validData = data else {
throw ParserError.emptyDictionary
}
Second you need to create an Array that holds all of the keys of your dictionary, using the .keys property.. This array will only contain the keys for your dictionary. You can use the .contains property on this array to check for the String you supply as an argument. This will return a bool, if false is returned, you will throw an error.
let dataKeys = Array(validData.keys)
guard dataKeys.contains("someKey") else {
throw ParserError.invalidKey
}
}