Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial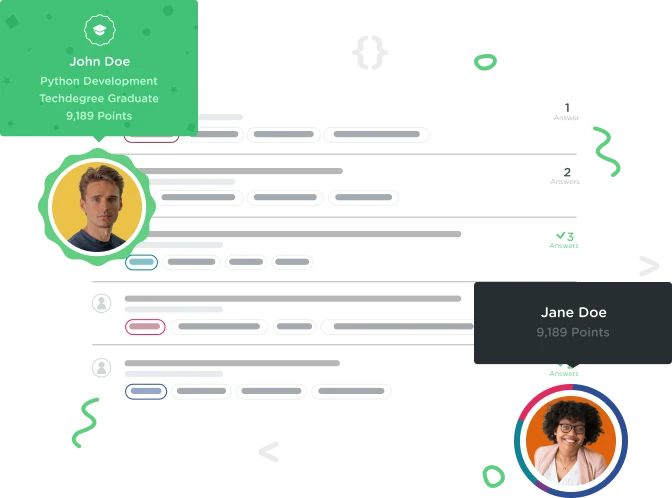

Luke Walker
1,049 PointsIn this task, we have an array of numbers and we want to compute the sum of its values. - No idea how to solve this!
Hello!
I just simply have no idea what I'm supposed to write here to solve this. I don'y know what to write to print back into the values it's asking me to change? If that is even correct to say?
Just feeling super lost.
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
1 Answer
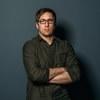
Jesse Gay
Full Stack JavaScript Techdegree Student 14,045 PointsBefore worrying about the exact syntax, let's think about what this challenge is asking. The goal is for the variable sum to store the value of the sum of all the numbers in the array. E.g. sum = 2+8+1+16+4+3+9. But, we need to do this programatically, and since it's a while loop, using a method that deals with each member of the array sequentially. The hint and first part of the challenge is: "Step 1: Create a while loop. The while loop should continue as long as the value of counter is less than the number of items in the array. (Hint: You can get that number by using the count property)"
in pseudo code:
while counter < (number of items in numbers array) {
// our code for calculating the sum
counter += 1
}
Then, for the second part: "Using the value of counter as an index value, retrieve each value from the array and add it to the value of sum...For example: sum = sum + newValue." So, we need to step through the array and add each number to sum. We know we can retrieve an item from an array via its index. E.g. numbers[0] returns 2, numbers[1] returns 8, etc. Conveniently, we have counter which will step through values from 0 to 6. So, can you think of how we can get each member of the array using this counter as an index?
pseudo code (this goes in the while loop)
let newValue = numbers[index derived from counter]
sum = sum + newValue
If you need to see the full answer, here's one solution
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
let newValue = numbers[counter]
sum = sum + newValue
counter += 1
}