Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial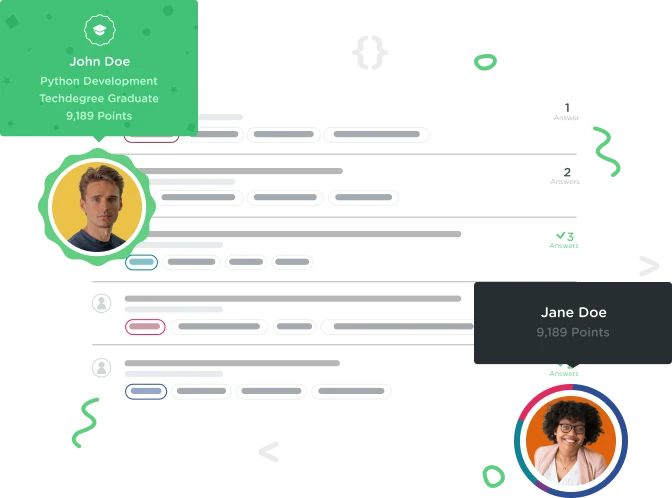
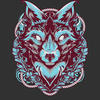
Neros E.
528 PointsThe Quest to Understand Constructors.
I’m having the hardest of times understanding what constructors are, I've gone through many other post and none really make it click for me, so whatever wizardly help I can get will change for the better a lot about my understanding of programming, thank you!
Update: I now know what Initializing means thanks to the awesome people below, these are my follow up questions on constructors if other wizards land by...apologies for the chimp level doubts, help is extremely appreciated.
Questions: -I’m not clear on where the argument (“Neros”) came from and its role all this, is it the object of the characterName variable inside the constructor or an extension of it of some kind? since java can't run the constructor without it.
-If so how does java know that "Neros" is an object of this variable when there hasn't been any assignment, like (characterName = "Neros";)?
Main code:
1 public class Example {
2
3 public static void main(String[] args) {
4 System.out.println("We are making a new PEZ dispenser");
5
6 PezDispenser dispenser = new PezDispenser("Neros");
7 System.out.printf("The dispenser is %s %n",
8 dispenser.getCharacterName()); } }
9 class PezDispenser{
10 private String mCharacterName;
11
12 public PezDispenser(String characterName) {
13 this.mCharacterName = characterName; }
14
15 public String getCharacterName() {
16 return mCharacterName; }
RETURN: We are making a new PEZ dispenser
The dispenser is Neros
I know this is all very elemental but I'm very new and getting the basics down is critical to me. Any help would be extremely appreciated, please feel free to break it down to potato level.
Original questions: -Constructors initialize a newly created object/instance but what does initialize even mean? Where in the code below is the object being "initialized", is it the same as instantiating? -What line in the main code below is the constructor exactly? line 6 or line 12 or are they both intrinsically the same?
example: of both line 6 and 12 constructor being the same, referencing the main code :
6 = New PezDispenser (value/object assigned by user) //class Instance automatically searches for any contructor-
- with the same name, stores object in objReference
12 = public PezDispenser (Str objReference) //So all references are null until object/value is assigned?
13 { this. mObjRefence = objReference }
2 Answers
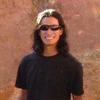
Daniel Vargas
29,184 PointsHi, I' not a expert but I'm going to try to explain you.
A class is like a blueprint and you can create objects from that blueprint. The act of creating a new object from that class is called instantiation (you create an instance of that class).
The initialization of the object is made by the constructor, this means the computer will asign some space in memory for this object (even if you don't use it later on that space in memory will be reserved).
The constructor of the class PezDispenser is the one at the line 12, then when you create the new object at line 6, the program calls that constructor and initialize the object.
Let me know if I was able to explain you well enough.
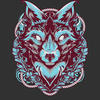
Neros E.
528 PointsThank you very much for your answers Daniel, with your answer and various sources I now understand the concept of constructors a lot more and definitely know what they are now, best wishes to you.
Allan Clark
10,810 PointsAllan Clark
10,810 PointsVery good answer! I did want to add on that usually when we are talking in terms of 'initialization' it's about the state the object is in just after construction. That is to say what the constructor sets the member variables to when the object is constructed.
mCharacterName is initialized to the value passed into the constructor.
If there were other member variables in this class that are not explicitly set by the constructor they would be initialized to the default value for their data type.
int default initializes to 0 bool default initializes to false String (also any other object) default initializes to null
Neros E.
528 PointsNeros E.
528 PointsThank you for the explanation Daniel and Allan! If you don’t mind answering I have 2 additional questions to add on your answers. I’m not clear on where the argument (“Neros”) came from and its role all this, is it the object of the characterName variable inside the constructor or an extension of it of some kind? since java can't run the constructor without it.
If so how does java know that "Neros" is an object of this variable when there hasn't been any assignment, like (characterName = "Neros";)?
Daniel Vargas
29,184 PointsDaniel Vargas
29,184 PointsWhen you defined your constructor:
You're telling the program to accept an argumente (a String) when you initialize the object. Then this String is passed to the member variable mCharacterName. Since you defined your constructor like this, java will always expect that String. You could defined a constructor that accepts more or even less arguments, but don't worry about that yet, you will learn that in the future here at treeehouse.
If you don't define a constructor java will implicitly generate one for you with no parameters:
And like Allan said when you don't explicitly give a value to the member variables in the object (in this case mCharacterName) java will initialize them to their default value, in the case of Strings this default value is
null
I know this all seem a little confusing, but you don't have to understand everything at the beginning. It will make more sense as you progress with your studies.