Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial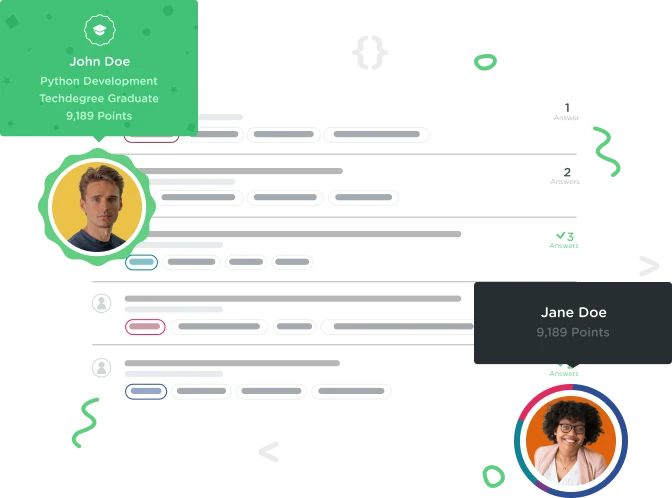

Muhammad Raza
3,678 PointsThis whole section promises fetch needs to have a better example that works. Since the API keeps throwing error
when you start the given example to work with the instructor it doesn't work to start with because of the promise.all() method none of them give any result since there is error in one the returned results. So to someone who is learning to the method to begin with its not fair that you have put such an complicated example and then it has bugs to begin with.
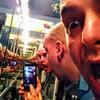
Bob Swaney
13,010 PointsWe (the community) might be able to help you work through the problems you are experiencing if you point out the exact part that is throwing the error and maybe post some of your code to make sure that you didn't miss anything. I recently completed that section of the JavaScript track and I didn't really have any issues with it. Im not trying to be a smarty-pants or anything, I'm just saying that it's possible that we might be able to help you out a little better if we saw an example of your code to see where the problem lies.
1 Answer
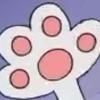
Shiyu Hong
12,681 PointsI posted this answer under a similar question (https://teamtreehouse.com/community/const-wikiurl-httpsenwikipediaorgapirestv1pagesummary-nothing-is-working) but hope this helps you out as well.
This is to deal with the issue that nothing displays using current data as of June 19 2020 from the two APIs, due to a name not found in Wikipedia.
The following code works for me. Hope it helps. Adding a bit of error handling so that information for the rest of the astronauts can be displayed.
First, in getProfiles function, I changed Promise.all() to Promise.allSettled(). Promise.all() needs all promises to be fulfilled, while Promise.allSettled() can have rejects. See MDN reference here: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise/all
Second, in generateHTML function, I added a error handling to ensure person.value.type === "standard", otherwise don't do anything for that iteration. This ensures any names not found in Wikipedia can be skipped.
const astrosUrl = 'http://api.open-notify.org/astros.json';
const wikiUrl = 'https://en.wikipedia.org/api/rest_v1/page/summary/';
const peopleList = document.getElementById('people');
const btn = document.querySelector('button');
function getProfiles(json) {
const profiles = json.people.map( person => {
return fetch(wikiUrl + person.name)
.then (response => response.json())
.catch (err => console.log('Error fetching Wikipedia:', err));
});
return Promise.allSettled(profiles);
}
function generateHTML(data) {
data.map( person => {
const section = document.createElement('section');
peopleList.appendChild(section);
if (person.value.type === "standard") {
section.innerHTML = `
<img src=${person.value.thumbnail.source}>
<h2>${person.value.title}</h2>
<p>${person.value.description}</p>
<p>${person.value.extract}</p>
`;
}
})
}
btn.addEventListener('click', (event) => {
event.target.textContent = 'Loading...';
fetch(astrosUrl)
.then (response => response.json())
.then(getProfiles)
.then(generateHTML)
.catch( err => {
peopleList.innerHTML = '<h3>Something went wrong</h3>';
console.log(err);
})
.finally(() => event.target.remove());
});
chels17
7,762 Pointschels17
7,762 PointsAgreed! I've been having so much difficulty with this entire section and it really needs to be updated :( I eventually found something that fixes some of it from the comments here, but I still think it needs to be updated.