Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial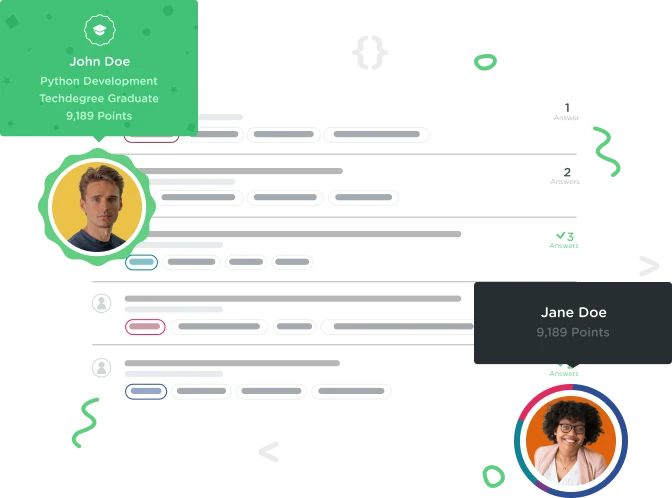

Isaac Miller
4,448 PointsTypeError in console
Edit: I just realized, in the beginning of the video Guil mentions that the fetch API was introduced in ES2015, has something changed between ES2015 and ES6?
Hi all, I keep getting the following error in the console: "TypeError: Cannot read properties of undefined (reading 'map') at getProfiles (promises.js:8)"
For reference, "console.log(json)" returns the following: ƒ json() { [native code] }
I am on the 'Full-Stack JavaScript' track, the majority of the track has really been a breeze up until this course. This video in particular seems to jump around a bunch and wasn't the easiest to follow compared to all previous courses. Maybe I just got spoiled with the previous courses or some things have changed in the API's since the video has been created? My code is below, hopefully it's not too hard to read, as I became very frustrated when working through it and wasn't focused on indentation/spacing and such. I'm hoping I am just missing something simple here. Any help is much appreciated! My code is below:
const astrosUrl = 'http://api.open-notify.org/astros.json';
const wikiUrl = 'https://en.wikipedia.org/api/rest_v1/page/summary/';
const peopleList = document.getElementById('people');
const btn = document.querySelector('button');
function getProfiles(json) {
console.log(json)
const profiles = json.people.map( person => {
const craft = console.log(person.craft);
return fetch(wikiUrl + person.name)
.then( response => response.json() )
.then(profile => {
return {...profile, craft };
})
.catch( err => console.log( 'Error fetching Wiki:', err) )
});
return Promise.all(profiles);
}
// Generate the markup for each profile
function generateHTML(data) {
data.map( person => {
const section = document.createElement('section');
peopleList.appendChild(section);
section.innerHTML = `
<img src=${person.thumbnail.source}>
<span>${person.craft}</span>
<h2>${person.title}</h2>
<p>${person.description}</p>
<p>${person.extract}</p>
`;
});
}
btn.addEventListener('click', (event) => {
event.target.textContent = "Loading..."
fetch(astrosUrl)
.then( response => response.json )
.then(getProfiles)
.then(generateHTML )
.catch( err => {
peopleList.innerHTML = '<h3>Something went wrong!</h3>'
console.log(err);
})
.finally( () => {
event.target.remove();
});
});
2 Answers

Kevin D
8,646 PointsIt could be possible that your json
variable needs to be invoked. If it's printing out ƒ json() { [native code] }
when you console log it, then it seems like a function and not an object that you're expecting back.
If that assumption is correct, then the TypeError
you're getting back makes sense because you're trying to call .map
on a property that's undefined.
For example, if you're doing json.people.map
I think this is what's happening:
-
json
exists - But since you haven't invoked the function, you're just using the function declaration
- So when you try to access
people
, it doesn't exist and isundefined
- Which means that you're essentially doing
undefined.map
Just guessing, but maybe try doing json().people.map
? Hope this helps a little in your debugging journey
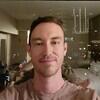
Jeremy Yochum
Front End Web Development Techdegree Graduate 16,023 PointsIn your event listener, the then( response => response.json )
statement is missing parentheses. I did the same thing, and my people map was undefined because there was no object to reference.
Julie Branyan
Full Stack JavaScript Techdegree Graduate 16,337 PointsJulie Branyan
Full Stack JavaScript Techdegree Graduate 16,337 PointsHi Isaac - Could you post a link to your workspace? This is a bit difficult to troubleshoot without it. If not, there is a markdown cheat sheet when posting that shows how to display the code block in a format that is easier to read. Thank you!