Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial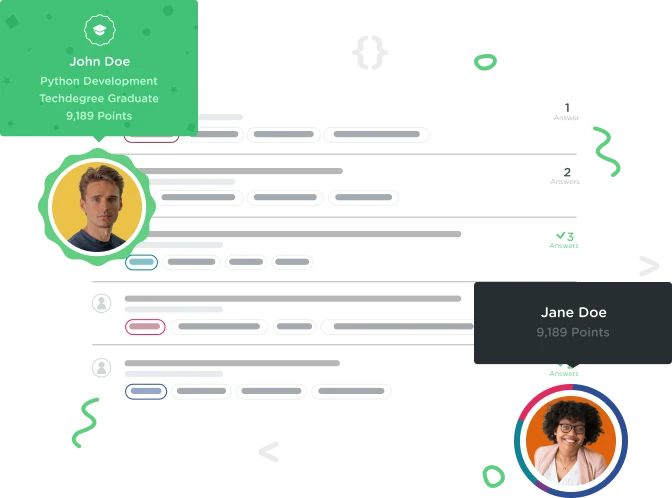

royalcompass
4,758 PointsWhat if I need two options for one question?
I would like question number 4 to be valid if a person entered usa or united states, how can this be accomplished? I initially tried by adding the logical or operator to the answer but that didn't work. Any reply is greatly appreciated.
/*
1. Store correct answers
- When quiz begins, no answers are correct
*/
let correct = 0;
// 2. Store the rank of a player
let rank;
// 3. Select the <main> HTML element
const main = document.querySelector('main');
/*
4. Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers
*/
const answer1 = prompt('What Street Do You Live On?');
if ( answer1.toUpperCase() === 'SPINKS' ) {
correct += 1;
}
const answer2 = prompt('What City Do You Live In?');
if ( answer2.toUpperCase() === 'UNION' ) {
correct += 1;
}
const answer3 = prompt('What State Do You Live In?');
if ( answer3.toUpperCase() === 'MISSISSIPPI' ) {
correct += 1;
}
const answer4 = prompt('What Country Do You Live In?');
if ( answer4.toUpperCase() === 'UNITED STATES') {
correct += 1
}
const answer5 = prompt('What Is Your Street Number?');
if ( answer5 === '307') {
correct += 1;
}
/*
5. Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown
*/
if ( correct === 5 ) {
rank = "gold";
} else if ( correct >= 3 ) {
rank = "silver";
} else if ( correct >= 1 ) {
rank = "bronze";
} else {
rank = "None :(";
}
// 6. Output results to the <main> element
main.innerHTML = `
<h2>You Got ${correct} out 5 questions correct.</h2>
<p>Crown earned: ${rank}</strong></p>.`
1 Answer
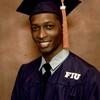
Dane Parchment
Treehouse Moderator 11,075 PointsThere are a few ways you can go about doing this. My preferred method, if you have knowledge of arrays, is to utilize the array's include method to see if the string provided is included in any of the array elements. We could do so like this:
let question4Answers = ['usa', 'united states'];
if(question4Answers.includes(question4.toLowerCase()) {
console.log("right answer!");
}
note that I converted the answer to lowercase so that we wouldn't have to worry about case sensitivity, but do note that the the array includes method is case sensitive!
if you do not know arrays however, you can still implement this via a logical OR (||) like so:
let caseInsentive4 = question4.toLowerCase();
if(caseInsentive4 === 'usa' || caseInsensitive4 === 'united states') {
console.log("right answer!");
}
That's how I would do it. There are a few other options (regular expressions, string.includes
, dictionary like structures, etc.), but these in my opinion are the most easy ones to understand, implement, and are plenty efficient. (I use the first example a lot in production code)