Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial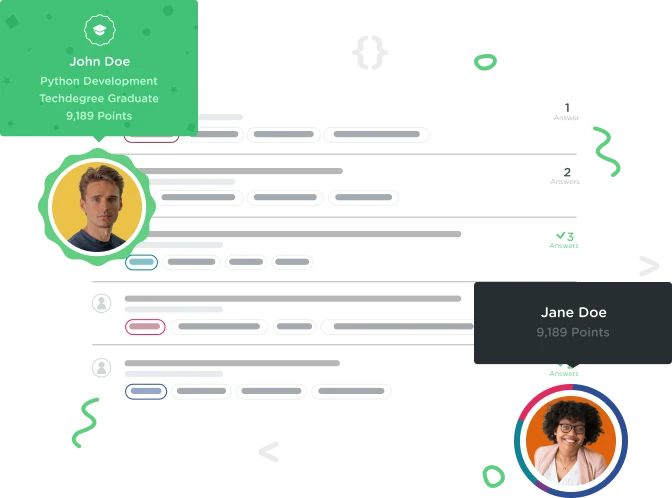

zach poll
1,087 PointsWhere should I put this loop?
const yourAnswer = prompt('Please type a number!'); const yourNumber = parseInt(yourAnswer);
if(yourNumber) { const randomNumber = Math.floor( Math.random() * yourNumber) + 1;
alert(${randomNumber} is a random number between ${yourNumber} and 1!
);
} else {
alert('You need to provide a number. Try again!');
}
// I was messing around trying to create a loop to trigger the the prompt for yourNumber to re "prompt" if the answer provided was not a number instead of having to refresh the page but i'm not really sure how or where to add the loop.
2 Answers
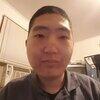
Joseph Yhu
PHP Development Techdegree Graduate 48,635 Pointslet yourAnswer; // Initialize the variable.
do {
// Prompt the user for a number and assign it to the yourAnswer variable.
yourAnswer = prompt('Please type a number!');
} while (isNaN(yourAnswer)); // Continue prompting the user while yourAnswer is not a number.*
*Something like isNaN('123')
will return false, so you don't have to parse the yourAnswer
variable into a number in the do-while loop. You can do it after the loop.
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/isNaN
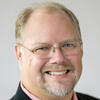
Jason Larson
8,355 PointsYou have a few things that need to be changed. First, if you're going to be asking for the answer and converting it, you don't want those variables to be constants. You left out the backticks in your alert where you display the randomnumber. Your alert with the randomnumber is a little odd grammatically, as most people would expect the lower number to be first. As for the loop, you would want to use a while loop. Here is a version that should do what you want:
let yourAnswer = prompt('Please type a number!');
let yourNumber = parseInt(yourAnswer);
while (!yourNumber){
alert(`You need to provide a number. Try again!`);
yourAnswer = prompt('Please type a number!');
yourNumber = parseInt(yourAnswer);
}
const randomNumber = Math.floor( Math.random() * yourNumber) + 1;
alert(`${randomNumber} is a random number between 1 and ${yourNumber}!`);