Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial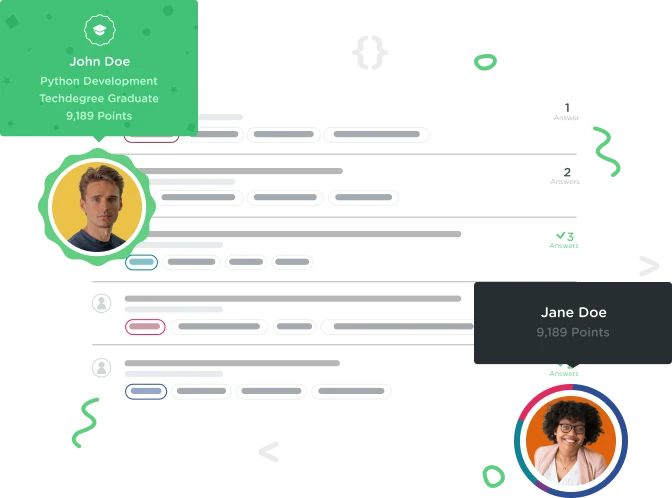

Anika Mehta
107 PointsWhy did he change first fill() method?
Why did he changed first public void fill() what does the code mean?
1 Answer

Yanuar Prakoso
15,196 PointsHi Anika
Just to confirm this is your first fill() method: (let us just call it first case)
public void fill() {
pezCount = MAX_PEZ;
}
And then Craig changed it to this:
public void fill() {
fill(MAX_PEZ); //this method fill using full mag size of full one dispenser but still tested using another public void fill(int pezAmount) method
}
public void fill(int pezAmount) {
int newAmount = pezCount + pezAmount;
if (newAmount > MAX_PEZ) {
throw new IllegalArgumentException("Too many PEZ!!!");
}
pezCount = newAmount;
}
If you are just curious why he must changed the first public void fill () method from:
public void fill() {
pezCount = MAX_PEZ;
}
//to the second code:
public void fill() {
fill(MAX_PEZ); //this method fill using full mag size of full one dispenser but still tested using another public void fill(int pezAmount) method
}
The main reason is because there are people who only know that to fill the dispenser you use dispenser.fill() method. Thus it will try to refill using full size refill dispenser (MAX_PEZ). However, it now is not the only case. We can fill using any number of refills as long as it does not exceed MAX_PEZ. Therefore it will make a test if someone still using dispenser.fill() method by testing if it is possible to do so (refill with full size refill). Inside the code there is code:
pezCount(MAX_PEZ);
This code basically called the second fill method (the public void fill(int pezAmount)) and passing the value of MAX_PEZ as argument in place of int pezAmount. This is the real practical example of method overloading. Thus the compiler then tested if it is possible to refill using MAX_PEZ amount of refill. If inside the dispenser there are still some candies left the system will THROW a warning telling you "Too many PEZ". Then you need to decide if you want to refill using the second fill method or wait until all candies in the dispenser are out and the dispenser isEmpty(). If you still curious I add extra explanation. But it might be long and boring. So if you already get the answer you may skip.
I will explain from the general case to the specific. If you feel that you do not need the general background story behind this code you can just skip to the technical ones.
So here is the explanation. In the first case the code assumes that we only will refill our PezDispenser only and only if all candy inside the dispenser is all been eaten or dumped. So if you use method dispenser.fill() in the Example.java it will fill the dispenser with all 12 candies (as in the MAX_PEZ constant). This is the first case.
However, this is not how real life PezDispenser works right? We can reload with any number of candies as we like as long as it does not exceeed MAX_PEZ = 12. Therefore we goes into the second case:
Now This is more technical explanation. Java allows us to use what it is call method overloading. It means we can make two different method which do two different things but using the same name. However, there is a caveat, those two different methods must have different arguments as inputs. As you can see in the second code:
public void fill() {
//code here bla...bla...bla
}
public void fill(int pezAmount) {
//code here bla...bla...bla...
}
both methods are called fill but those two received different arguments (no argument for the first fill and int pezAmount for the second one) and the rest is just like what I said above: This code basically called the second fill method (the public void fill(int pezAmount)) and passing the value of MAX_PEZ as argument in place of int pezAmount. This is the real practical example of method overloading. Thus the compiler then tested if it is possible to refill using MAX_PEZ amount of refill. If inside the dispenser there are still some candies left the system will THROW a warning telling you "Too many PEZ". Then you need to decide if you want to refill using the second fill method or wait until all candies in the dispenser are out and the dispenser isEmpty().
I hope this can help you a little.