Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial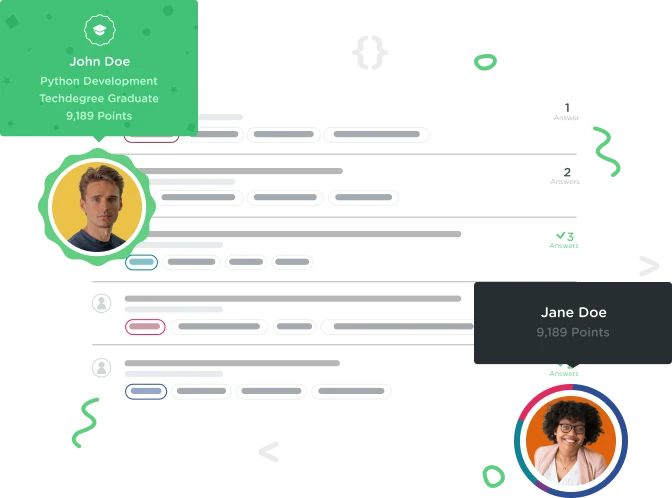
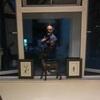
james viggers
1,397 Points!= always fireing
here is my code so far:
import sys
player_names = []
add_player_to_list = input("Would you like to add a player to the list? Yes/No: ")
print(add_player_to_list)
if add_player_to_list.lower() != "yes" or "no":
sys.exit("Sorry i dont understand")
else:
while add_player_to_list.lower() == "yes":
player_name = input("Please enter the players name ")
player_names.append(player_name)
add_player_to_list = input("Would you like to add another player to the list? Yes/No: ")
if len(player_names) <= 1:
sys.exit("There are not enough players to continue")
print("There are {} players in the team\nTeam Roster".format (len(player_names)))
player_index_number = 1
for player in player_names:
print("Player {} : {}".format(player_index_number,player))
player_index_number += 1
goalkeeper = int(input("Select a goalkeeper from the list by selecting the player number. (1-{})".format(len(player_names))))
goalkeeper_index = goalkeeper -1
print("The chosen goalkeeper is {} ".format(player_names[goalkeeper_index]))
Can anyone please explain to me why this part below thats at the top of my code is allways fireing even when i type yes or no as it is really confusing me:
if add_player_to_list.lower() != "yes" or "no": sys.exit("Sorry i dont understand")
1 Answer
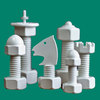
Steven Parker
230,274 PointsYou can't use logic ("and" / "or") to combine terms, but you can use it to combine complete expressions:
if add_player_to_list.lower() != "yes" or "no": # this won't do what you expect
if add_player_to_list.lower() != "yes" and add_player_to_list.lower() != "no": # this will
Also, I think what you are calling "fireing" is more commonly referred to as "running".
james viggers
1,397 Pointsjames viggers
1,397 PointsThanks, was wondering why it kept being triggered.
james viggers
1,397 Pointsjames viggers
1,397 Pointscan you please tell me why the and command works but the or does not? i would of thought being either or would be the correct way to do this and the and would have to have both requirements met to be true....
Steven Parker
230,274 PointsSteven Parker
230,274 PointsIn logic, "and" requires both joined expressions to be true. But "or" only requires either expression to be true.
But mainly, you must always have complete expressions on both sides of the logic keyword. For example,
add_player_to_list.lower() != "no"
is a complete comparison expression, but just"no"
is not.james viggers
1,397 Pointsjames viggers
1,397 Pointsright i got it working like this:
Would this be the right way to do things as i have two loops going on at the same time...
Steven Parker
230,274 PointsSteven Parker
230,274 PointsMaking your code compact and efficient is a skill you will acquire with with experience and practice. One aspect of that is called the "DRY" principle, and will be covered in later courses. But making the code work will always be of primary importance.