Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial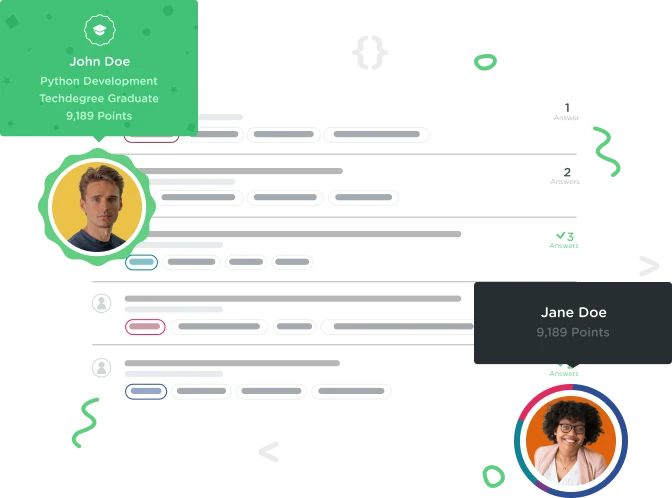

Inokentiy Kadilov
28,532 PointsAm I missing something? The code works in Xcode.
extension String { func transform(_ argument:(String) -> String) -> String { return argument(self) }
}
extension Character { var isVowel: Bool { let vowels: [Character] = ["a", "e", "i", "o", "u"] return vowels.contains(self) } }
func removeVowels(from value: String) -> String { var output: String = " "
for char in value.characters {
if !(char.isVowel){
output.append(char)
}
}
let newString:String = output
return newString
}
removeVowels(from: "apple")
extension String {
func transform(_ argument:(String) -> String) -> String {
return argument(self)
}
}
extension Character {
var isVowel: Bool {
let vowels: [Character] = ["a", "e", "i", "o", "u","A", "E", "I", "O", "U"]
return vowels.contains(self)
}
}
func removeVowels(from: String) -> String {
var output: String = " "
for char in from.characters {
if !(char.isVowel){
output.append(char)
}
}
let string:String = output
return from
}
1 Answer
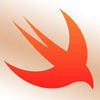
Jeff McDivitt
23,970 PointsThis is how I handled this one. I am sure there are multiple ways to do it
extension String{
func transform(_ argument : (String) -> String) -> String {
return argument(self)
}
}
func removeVowels(from value: String) -> String {
var output = ""
for char in value.characters {
if !(char == "a" || char == "A" || char == "e" || char == "e"
|| char == "i" || char == "I" || char == "o" || char == "O"
|| char == "u" || char == "U") {
output.append(char)
}
}
return output
}