Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial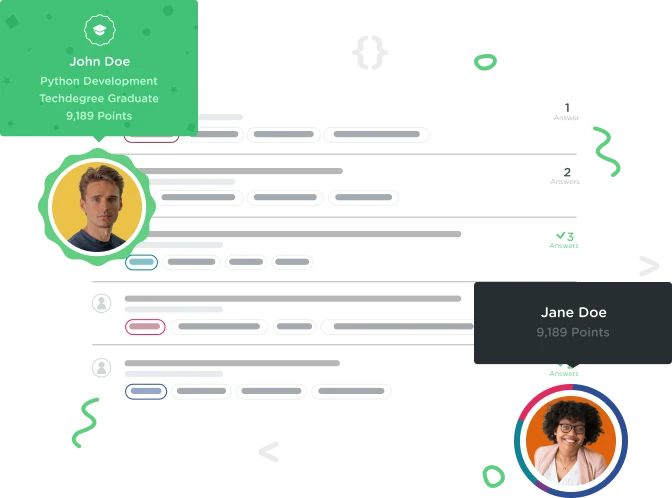

Megan Bullington
6,245 Points++ and -- to be removed from Swift
When I type in the code as it appears in this lesson, a yellow triangle appears on the side with the message "++ is deprecated: it will be removed in Swift 3". The code still executes, but I am interested to know how I would go about creating the code for this lesson without using ++ or --. My Google searches found that I could replace levelScore++ with levelScore +=1. For the section of code: totalScore = levelScore++ I can't replace levelScore++ with levelScore += 1. How would you write that line without using ++?
2 Answers
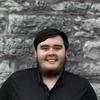
Michael Hulet
47,912 PointsYou would have to split it into 2 lines, like this:
totalScore = levelScore
levelScore += 1
The reason ++
and --
are being removed is made evident in this example, actually. In Swift, operators (like +
or -
, or even ++
and +=
) are just functions that you can define (try overriding one!), and like any other function, they can return
a value. The difference that makes you split it into 2 different lines is that ++
returns a value (which is different based on context), but +=
returns Void
(which is nothing at all). This means that in this line:
totalScore = ++levelScore
1 is added to levelScore
, and that new value is assigned to totalScore
. In this line, though:
totalScore = levelScore += 1
1 is added to levelScore, but it doesn't hand the new value to totalScore
, and so you get a compiler error, instead. One reason ++
and --
are being removed is because they stand out, because they're the only 2 assignment operators that return a value.
The other reason ++
and --
are being removed is because in Swift 1 & 2, ++
and --
are both prefix and postfix operators (which means they can be put before or after the variable name), and they perform differently in each context. Let's take my above example:
totalScore = ++levelScore
In this context, 1 is added to levelScore
, then that value is assigned to totalScore
. This is not the same when it's used as a postfix operator, however. When you do this:
totalScore = levelScore++
The value of levelScore
before 1 is added is assigned to totalScore
, and then 1 is added to levelScore. That is why in the first part of my answer, I assigned levelScore
to totalScore
, and then added 1 to levelScore
.
In short, ++
and --
are being removed not only because they stand out among their peers, but also because they come with very specific semantics that relatively few programmers know about
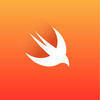
Steven Deutsch
21,046 PointsIf you check out the accepted proposal to remove the ++ and -- operators from Swift:
Under the advantages section:
"When the return value is needed, the Swift += operator cannot be used in-line, since (unlike C) it returns Void."
Under the disadvantages section, point #3 reads:
"Swift already deviates from C in that the =, += and other assignment-like operations returns Void (for a number of reasons). These operators are inconsistent with that model."
Steven Deutsch
21,046 PointsSteven Deutsch
21,046 PointsVery interesting point Michael Hulet
Steven Deutsch
21,046 PointsSteven Deutsch
21,046 PointsUPDATE: There was a different discussion that the first line of this comment references, but one member of said discussion deleted all of his comments, so this line is out of context. This comment is kept in its entirety for archival purposes
Right, I receive the same warning as well due to the unexpected functionality of no value being returned.
Off topic, but how about this change to Swift 3:
https://github.com/apple/swift-evolution/blob/master/proposals/0054-abolish-iuo.md
NOTE: We could override the += operator's implementation to return a value? We could also recreate the depreciated ++ and -- operators if we truly wanted to do so.
Michael Hulet
47,912 PointsMichael Hulet
47,912 PointsI haven't looked over all the changes for Swift 3, but that seems like it'll lead to more Swifty code in the long run, but will be kinda annoying to deal with short-term, especially because Interface Builder-generated declarations are currently
ImplicityUnwrappedOptional
s.You could definitely do that, and though it isn't recommended for public clarity, but tbh I'm totally doing that, because I learned C/Objective-C first (and that's the default in those languages), so it feels more natural to me for it to be that way
Jarren Bird
8,125 PointsJarren Bird
8,125 PointsThis is a good and helpful post, Michael. Thank you.