Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial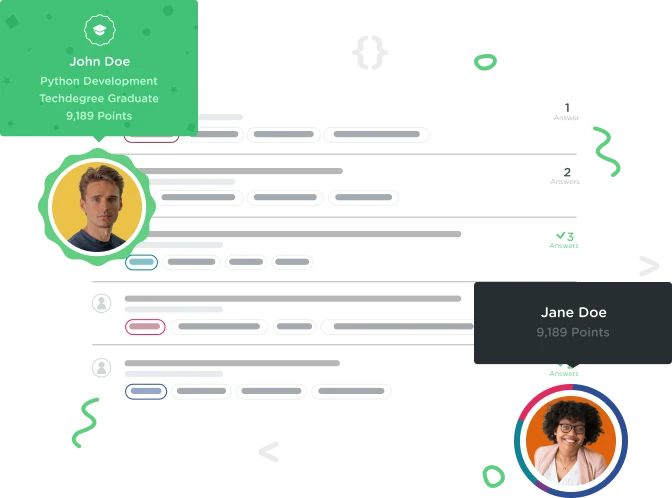
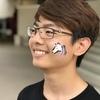
Matt Song
2,971 PointsAttributeError: 'builtin_function_or_method' object has no attribute 'remove'
I can't get past this error. I don't know what it means.
def disemvowel(word):
next_word = 0
lower_word = word.lower
upper_word = word.upper
if next_word == 0:
try:
lower_word.remove('a')
upper_word.remove('a')
next_word += 1
except ValueError:
pass
elif next_word == 1:
try:
lower_word.remove('e')
upper_word.remove('e')
next_word += 1
except ValueError:
pass
elif next_word == 2:
try:
lower_word.remove('i')
upper_word.remove('i')
next_word += 1
except ValueError:
pass
elif next_word == 3:
try:
lower_word.remove('o')
upper_word.remove('o')
next_word += 1
except ValueError:
pass
elif next_word == 4:
try:
lower_word.remove('u')
upper_word.remove('u')
next_word += 1
except ValueError:
pass
else:
return word
2 Answers
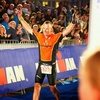
Steve Hunter
57,712 PointsHi Matt,
I'm not sure what your error is but it isn't liking the use of remove
for some reason.
Let's try walking through the solution here. We're given a word
which can be a mixture of upper and lower case letters. We want to return word
, with the cases preserved, but without the vowels.
I found this challenge easier to build the output string, rather than remove the unwanted letters - removing from something you're iterating over causes problems. I started by declaring an array of lower case vowels. I also declared an empty output
string. I then looped over word
with a for
loop. At each iteration, I tested whether the current letter (lowercased) was not in
the array of lowercase vowels. If it wasn't (i.e. we want to include it in the output string) I added with +=
to the empty output
string. Once the loop had completed, I returned the output
string.
That saves testing every vowel and preserves the case of the output string, which is required. It also took up seven lines of code so is pretty efficient.
I hope that helped you with the challenge, if not the error itself.
Steve.
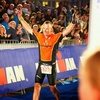
Steve Hunter
57,712 PointsA few things before I try your code out.
The output
is a string - i think you might need to use double quotes for that (I may be wrong!).
Don't lowercase word
you want to preserve the case of the input word
.
You're looping through the wrong thing. Loop through the input word
; that pulls out each letter
, then see if that letter
is not in
vowels. But lowercase it first so, for letter in word:
starts your loop. Then see if letter.lower
isn't in the array. If it isn't add letter
to output
.
Lastly, for return
the output
, don't print
it.
Try that and let me know how you get on.
Steve.
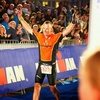
Steve Hunter
57,712 PointsChecked - you can use single quotes for the output
string declaration but the challenge is eager to have the parentheses for the lower()
method.
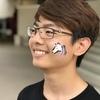
Matt Song
2,971 PointsI changed it to this and it seems to work. How would I do the upper case vowels as well?
def disemvowel(word):
lower_vowels = ["a", "e", "i", "o", "u"]
output = ""
for letter in word:
if letter not in lower_vowels:
output += letter
return(output)
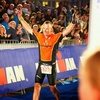
Steve Hunter
57,712 PointsThat's great work.
To check the lowercase/uppercase, just convert the letter
to lower at the point of testing - so no permanent change - that way you preserve the case out the output to be the same as the input:
def disemvowel(word):
lower_vowels = ["a", "e", "i", "o", "u"]
output = ""
for letter in word:
if letter.lower() not in lower_vowels:
output += letter
return(output)
Matt Song
2,971 PointsMatt Song
2,971 PointsI tried this, but I don't think I did it right. I'm not getting the right answer.