Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial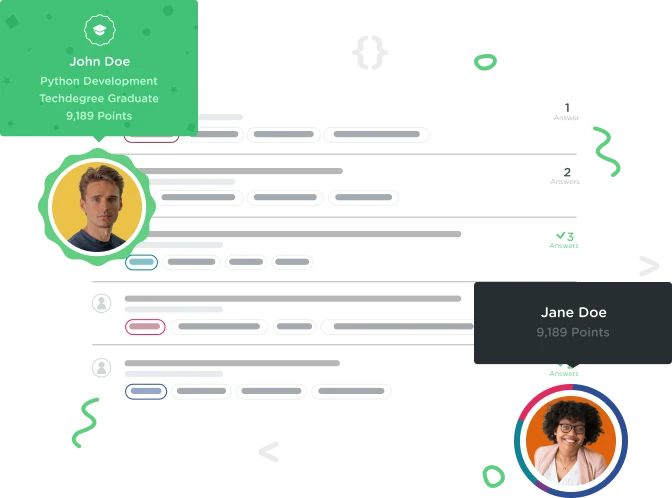

Sylwester Guzek
16,088 PointsBummer! Try again!
Dear Treehouse team,
Code is passing the tests on my laptop, but after pasting it here does not work, and does not give any error message.
Please let me know how to proceed.
Thanks
from flask import Flask, g, render_template, flash, redirect, url_for, abort
from flask_bcrypt import check_password_hash
from flask_login import (LoginManager, logout_user, login_required, login_user,
current_user)
import forms
import models
import sys
DEBUG = True
PORT = 8000
HOST = '0.0.0.0'
app = Flask(__name__)
app.secret_key = "hfkjhsdflkash"
login_manager = LoginManager()
login_manager.init_app(app)
login_manager.login_view = 'login'
@login_manager.user_loader
def load_user(userid):
try:
return models.User.get(models.User.id == userid)
except models.DoesNotExist:
return None
@app.before_request
def before_request():
"""Conect to db before each request"""
g.db = models.DATABASE
#g.db.connect()
g.user = current_user
@app.after_request
def after_request(response):
"""Close db connection after each req"""
#g.db.close()
return response
@app.route('/login',methods=('GET', 'POST'))
def login():
form = forms.LoginForm()
if form.validate_on_submit():
try:
user = models.User.get(models.User.email == form.email.data)
except models.DoesNotExist:
flash("your email or password doesn't match")
else:
if check_password_hash(user.password, form.password.data):
login_user(user) #creating cookie
flash("you have been loged in")
return redirect(url_for("index"))
else:
flash("your email or password doesn't match")
return render_template('login.html',form=form)
@app.route('/logout')
@login_required
def logout():
logout_user() #deleting cookie
flash("you have been logged out","success")
return redirect(url_for('index'))
@app.route('/register', methods = ['GET','POST'])
def register():
form=forms.RegisterFrom()
if form.validate_on_submit():
flash("Congrat, you registered", category='success')
models.User.create_user(
# username=form.username.data,
email=form.email.data,
password=form.password.data
)
return redirect(url_for('index'))
return render_template('register.html',form=form)
@app.route('/taco', methods = ('GET','POST'))
@login_required
def post():
form = forms.TacoForm()
if form.validate_on_submit():
models.Taco.create(user = g.user._get_current_object(),
protein = form.protein.data.strip(),
shell = form.shell.data.strip(),
cheese = form.cheese.data,
extras = form.extras.data.strip())
flash("Taco posted")
return redirect(url_for("index"))
return render_template('taco.html', form=form)
@app.route('/')
def index():
tacos = models.Taco.select().limit(100)
return render_template('index.html', tacos = tacos)
@app.errorhandler(404)
def not_found(error):
return render_template('404.html'), 404
if __name__=='__main__':
models.initialize()
try:
models.User.create_user(
email="user@example.pl",
password="user",
)
except ValueError:
pass
import datetime
from flask_login import UserMixin
from flask_bcrypt import generate_password_hash
from peewee import *
DATABASE = SqliteDatabase('taco.db')
class User(UserMixin, Model):
# username = CharField(unique = True)
email = CharField(unique = True)
password = CharField(max_length = 100)
joined_at = DateTimeField(default = datetime.datetime.now)
is_admin = BooleanField(default = False )
class Meta:
database = DATABASE
order_by = ('-joined_at', )
def get_posts(self):
return Taco.select().where(Taco.user == self)
# def get_stream(self):
# return Post.select().where(
# (Post.user << self.following()) |
# (Post.user == self)
# )
@classmethod
def create_user(cls, email, password, admin=False):
try:
with DATABASE.transaction(): # if following action will not finish success cancel transaction
cls.create(
# username=username,
email=email,
password=generate_password_hash(password),
is_admin=admin
)
except IntegrityError:
raise ValueError("User already exists")
class Taco(Model):
timestamp = DateTimeField(default = datetime.datetime.now)
protein = CharField(max_length = 100)
shell = CharField(max_length = 100)
cheese = BooleanField(default = False )
extras = TextField()
user = ForeignKeyField(
rel_model = User,
related_name = 'tacos'
)
class Meta:
database = DATABASE
order_by = ('-timestamp',)
def initialize():
DATABASE.connect()
DATABASE.create_tables([User, Taco], safe=True)
DATABASE.close()
from flask_wtf import FlaskForm
from models import User
from wtforms import StringField, PasswordField, TextAreaField, BooleanField
from wtforms.validators import (DataRequired, Regexp, ValidationError,
Email, Length, EqualTo)
# def name_exists(form, field):
# if User.select().where(User.username == field.data).exists():
# raise ValidationError('User with that name already exists')
def email_exists(form, field):
if User.select().where(User.email == field.data).exists():
raise ValidationError('User with that email already exists')
class RegisterFrom(FlaskForm):
# username = StringField(
# 'Username',
# validators = [
# DataRequired(),
# Regexp(
# r'^[a-zA-Z0-9_]+$',
# message = "Username should be one word letters numbers"
# ),
# name_exists
# ])
email = StringField(
'Email',
validators = [
DataRequired(),
Email(),
email_exists
])
password = PasswordField(
'Password',
validators = [
DataRequired(),
Length(min=2),
EqualTo('password2',message = "Password must match")
])
password2 = PasswordField(
'Confirm Password',
validators = [
DataRequired(),
])
class LoginForm(FlaskForm):
email = StringField("Email", validators = [ DataRequired(), Email() ])
password = PasswordField('Password', validators = [DataRequired()])
class TacoForm(FlaskForm):
protein = StringField("protein", validators = [ DataRequired() ])
shell = StringField("shell", validators = [ DataRequired() ])
cheese = BooleanField('cheese ?', validators= [ DataRequired() ])
extras = TextAreaField("extras", validators = [ DataRequired() ])
<!doctype html>
<html>
<head>
<title>Tacocat</title>
<link rel="stylesheet" href="/static/css/normalize.css">
<link rel="stylesheet" href="/static/css/skeleton.css">
<link rel="stylesheet" href="/static/css/tacocat.css">
</head>
<body>
{% with messages=get_flashed_messages() %}
{% if messages %}
<div class="messages">
{% for message in messages %}
<div class="message">
{{ message }}
</div>
{% endfor %}
</div>
{% endif %}
{% endwith %}
<div class="container">
<div class="row">
<div class="u-full-width">
<nav class="menu">
<!-- menu goes here -->
<div class="row">
<div class="three columns ">
<a href="{{ url_for('index') }}" class="icon-logo">LOGO</a>
</div>
<div class="three columns">
<!-- Say Hi -->
<h4>
{% if current_user.is_authenticated %}
Hi {{ current_user.email }}!
{% endif %}
</h4>
</div>
<div class="three columns">
<!-- Log in/Log out -->
{% if current_user.is_authenticated %}
<a href="{{url_for('logout')}}" class='icon-power' title='logout'>LOGOUT </a>
<a href="{{url_for('post')}}" class='icon-power' title='add a new taco'>add a new taco</a>
{% else %}
<a href="{{url_for('login')}}" class='icon-power' title='log in'> LOGIN</a>
<a href="{{url_for('register')}}" class='icon-profile' title='sign up'>sign up</a>
{% endif %}
</div>
</div>
</nav>
{% block content %}{% endblock %}
</div>
</div>
<footer>
<p>An MVP web app made with Flask on <a href="http://teamtreehouse.com">Treehouse</a>.</p>
</footer>
</div>
</body>
</html>
{% extends 'layout.html' %}
{% block content %}
<h2>Tacos</h2>
{% if tacos.count() %}
<table class="u-full-width">
<thead>
<tr>
<th>Protein</th>
<th>Cheese?</th>
<th>Shell</th>
<th>Extras</th>
</tr>
</thead>
<tbody>
{% for taco in tacos %}
<tr>
<th>{{ taco.protein }}</th>
<th>{{ taco.cheese }}</th>
<th>{{ taco.shell }}</th>
<th>{{ taco.extras }}</th>
</tr>
{% endfor %}
</tbody>
</table>
{% else %}
<p> no tacos yet </p>
{% endif %}
{% endblock %}