Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial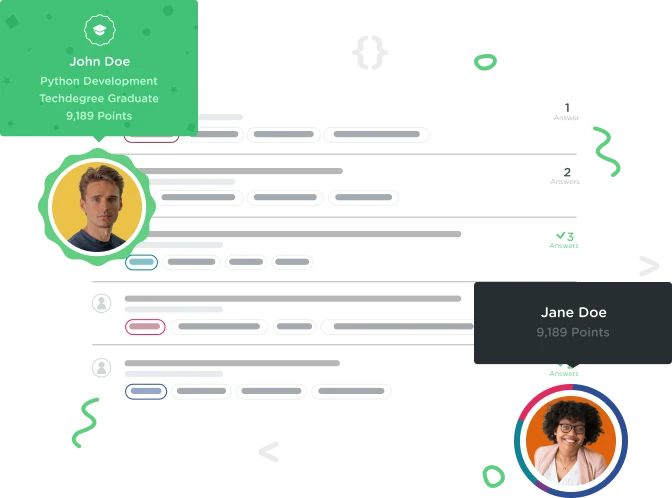

Cesar Manrique
3,708 PointsBummer! Uh oh. java.lang.ClassCastException: sun.reflect.generics.reflectiveObjects.ParameterizedTypeImpl cannot be cas
need help
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.Arrays;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
import java.util.HashMap;
import java.util.Map;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
public Map<List<String>,Integer> getCategoryCounts(){
Map<List<String>,Integer> CategoryCounts = new HashMap<List<String>,Integer>();
for (BlogPost post: mPosts) {
List<String> pCategory = new ArrayList<String>();
pCategory.add(post.getCategory());
for(String Category : pCategory){
Integer count = CategoryCounts.get(Category);
if(count == null){
count = 0;
}
count++;
CategoryCounts.put(pCategory,count);
}
}
return CategoryCounts;
}
}
1 Answer

Yanuar Prakoso
15,196 PointsHi Cesar
First of all you only need to Map the blog category (which is a String) with how many times (frequency) it came up in a List of Blog Posts which is an integer. Thus the Map you set it should be Map<String, Integer> not between List of Strings and Integer.
Next you do not need any List in this method. You can directly map the relation between the category and the frequency of it come up using HashMap. Here is my proposed Code:
public Map<String, Integer> getCategoryCounts(){
Map<String, Integer> getCategoryCounts = new HashMap<String, Integer>();
for (BlogPost post : mPosts){
if(getCategoryCounts.get(post.getCategory()) == null){
getCategoryCounts.put(post.getCategory(), 0);
}
int count = getCategoryCounts.get(post.getCategory());
count++;
getCategoryCounts.put(post.getCategory(), count);
}
return getCategoryCounts;
}
I hope this will help you pass the challenge. If you can conclude the logic behind the code by reading my source code that will be great. If you have not and curious about it I can offer further explanation below. However, I apologize if it will a bit long and boring.
As I said above you need to fix your Map declaration with reason mentioned above. Then you get to loop FOR each post in mPosts which is a List of Blog Posts. Then this is the reason you do not need to declare a new List to hold the data of blog post categories. It is because you can always access it using post.getCategory() method then you get the catogory of the blog post. Using this data you can update the data in the Map using getCategoryCounts.get(post.getCategory()) method.
IF the category is not yet inside the Map then the system will pass back a null value. This null is not zero and if it not treated correctly it will invoke error. Thus we use IF statement if null value is pass back to us then we understand that the category is not yet set as key in the Map. Therefore, we PUT it as key in the map using:
if(getCategoryCounts.get(post.getCategory()) == null){
getCategoryCounts.put(post.getCategory(), 0);
}
The 0 (zero) inside the put Map method meaning this is a new category key in the map thus it is not yet get any frequency. We will add one after we exit the IF statement code. :
int count = getCategoryCounts.get(post.getCategory());
count++;
getCategoryCounts.put(post.getCategory(), count);
IF the category already exist inside the Map as key the it will get you the counts or frequency of how many times it surface. Then we can directly update the count by incrementing (using count++) and then put it again in the map.
This loop goes on until all mPosts are inspected. Finally after all already put in Map as arraged we return the Map :
return getCategoryCounts;
That is all from me. I hope it will help you a little.