Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial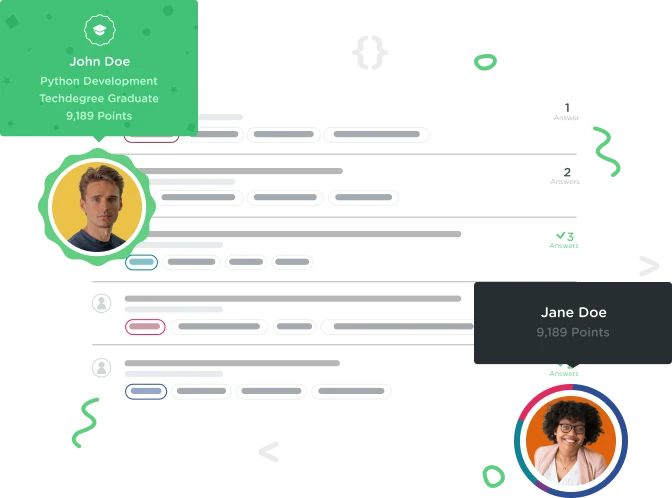

ISAIAH S
1,409 PointsCode Challenge "Throwing Exceptions"
BUMMER! Are you sure you threw the IllegalArgumentException if the requested amount of laps would make the battery less than zero?
task 1 of 1
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
if (mBarsCount < 0) {
throw new IllegalArgumentException("Not enough battery remains");
}
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
3 Answers
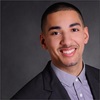
Mario Blokland
19,750 PointsHey ISAIAH S,
it is actually almost the same like in the video before this exercise. I altered your code a little bit with pseudo code:
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
try {
// This here calls the drive method from below
drive(1);
} // Catch the error here {
// print the error
}
}
public void drive(int laps) {
// Create a new variable and set the value to (mBarsCount - laps).
/* If you do that, the new value can be
checked first, before assigning it to mBarsCount.
If you don't, let's say if the value goes below 0, a bomb
will explode and after that, the exception will be thrown. You don't want that.
You want to throw the exception before the bomb goes off
*/
if (theNewVariable < 0) {
// throw exception
}
/* set mBarsCount to the value of the new variable.
This code here (after the if statement)
won't get executed if the new value is below 0,
because then an exception will be thrown first
*/
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
I hope this helps a little bit more.
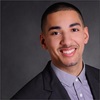
Mario Blokland
19,750 PointsHi,
the problem here is that you call the method drive and then check if the value is < 0. But by the time you check the value of mBarsCount you already altered the value to less than 0. So yes, your function will still throw an exception but the variable by then has already been altered to an invalid value. So you have to find a way which won't allow to so set the value below 0.

ISAIAH S
1,409 PointsHow?

Eric De Wildt
Courses Plus Student 13,077 PointsThere are two drive methods and I know that's a little confusing, but the method we want is the one that changes the value of mBarsCount. You are right about not wanting mBarsCount to go below 0 because that's not possible. But ask yourself is it possible to drive 7 laps on 6 bars of charge?