Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial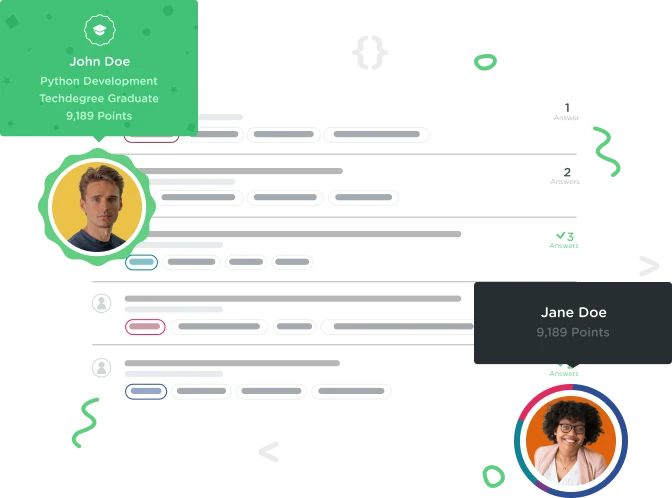

Quicken Loans
12,654 PointsCode review request: Kaprekar's constant
I remembered this interesting math equation know as Keprakar's constant and thought it would be an interesting programming exercise.
Basically, you take any 4 digit number, with at least 2 distinct digits. 6235 for example. Then rearrange it into descending (6532) and ascending (2356) and subtract the small number from the big number. The difference here is 4176. You then repeat the process with the difference number until you reach 6174 at which point you will get 6174 forever. Its really pretty simple
This video (3min) explains it in more detail: https://youtu.be/d8TRcZklX_Q
Anywho, I wrote some javascript to do this calculation and would love to get some thoughts on it. I commented on everything to explain whats going on. What could I do better?
Codepen Link: http://codepen.io/johndavidsimmons/pen/zGWgoe
2 Answers
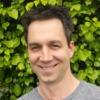
Matt Green
3,557 PointsLooks good. I would make the code a bit more modular, and do some error checking to avoid infinite loops. For modular/re-usable code I would avoid sharing information between the function, and button press code via global vars. Your code looks like it was headed in that direction with the return statement in the function, but you're not putting it to use.
I have done some changes, and put the data into an object, then returned the object. I also limited the iterations on the while loop to 50, which I'm sure is high for locating this constant (you can find the max necessary). http://codepen.io/anon/pen/vOrgdQ?editors=001
In addition to my changes, I would also do some checks on the user input before processing it, especially ensuring there are at least 2 unique integers. You could use a regex to ensure the input is only numbers, and you could throw an error to the user if the input was deemed invalid (and why).
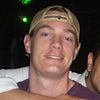
Joseph Rimar
17,101 PointsHey John,
For fun I messed around with this problem for a little before looking at your code and I came up with a similar solution using recursion. After checking out your code the only thing I could suggest is to validate that the user has entered a valid number to avoid the infinite loop you warned them about. Also, certain numbers (3332 or 3334 for example) throw a maximum call stack error and may freeze the browser.
Here's my recursive solution. I've used array.filter to validate the users input and avoid an infinite loop. The only problem is it will throw a call stack error on some numbers and I'm still trying to figure out how to avoid that.
function kaprekarConstant(num, count) {
if (typeof count == "undefined")
count = 1;
if(typeof num === 'null' )
return "Please enter a valid number"
var numArray= num.toString().split("").filter(function(a,b) {return a != b});
if(count < 2 && numArray.length < 4)
return "Please enter a valid number";
var highNumber = num.toString().split("").sort(function (a,b) {
return b-a }).join("");
var lowNumber = num.toString().split("").sort().join("");
var dNumber = highNumber - lowNumber;
if (dNumber === 6174) {
return "It took " + count + " tries to get to 6174.";
}
return kaprekarConstant(dNumber, count +1);
}
As you can see it does basically the same thing without using any loops. If you or anyone else has an idea of how to stop the call stack error please let me know. Thanks for the programing challenge, this was fun.
Joe