Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial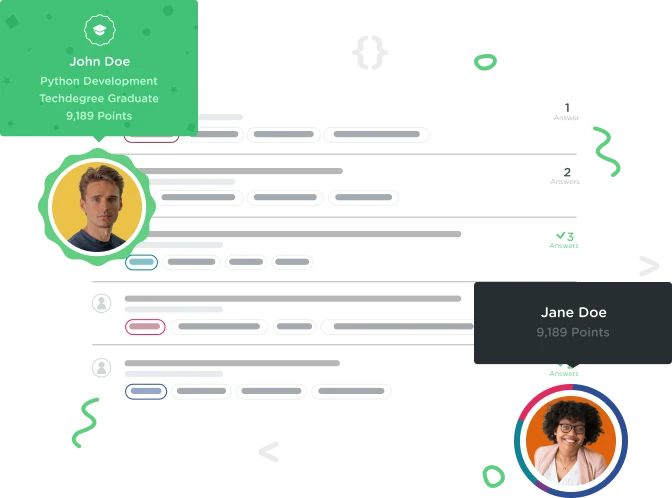

vikashkhanna
Courses Plus Student 586 Pointscompiler is not able to detect the error in the code
enum ParserError: ErrorType { case EmptyDictionary case InvalidKey }
struct Parser{ var data: [String : String?]? func parse() throws { guard (data?.keys) != nil else { throw ParserError.EmptyDictionary } guard data?.keys.contains("someKey") != nil else { throw ParserError.InvalidKey } } }
let data: [String : String?]? = ["someKey": nil] var parser = Parser()
do{ parser.data = data try parser.parse() }catch ParserError.InvalidKey{ print("Invalid Key") }
enum ParserError: ErrorType {
case EmptyDictionary
case InvalidKey
}
struct Parser{
var data: [String : String?]?
func parse() throws {
guard (data?.keys) != nil else {
throw ParserError.EmptyDictionary
}
guard data?.keys.contains("someKey") != nil else {
throw ParserError.InvalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
var parser = Parser()
do{
parser.data = data
try parser.parse()
}catch ParserError.InvalidKey{
print("Invalid Key")
}
1 Answer

Roberto Pando
9,486 PointsReally close, first you're checking if data is completely empty and you can do that by trying to unwrap it, secondly it wants to know if data contains a key named someKey, no need to check if is nil you probably got confused and tried to check that the key someKey was not empty: Your code:
func parse() throws {
guard (data?.keys) != nil else {
throw ParserError.EmptyDictionary
}
guard data?.keys.contains("someKey") != nil else {
throw ParserError.InvalidKey
}
}
changed to:
func parse() throws {
guard let data = data else {
throw ParserError.EmptyDictionary
}
guard data.keys.contains("SomeKey") else {
throw ParserError.InvalidKey
}
}
After that you just need to exhaust the errors by adding a catch block for .EmptyDictionary