Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial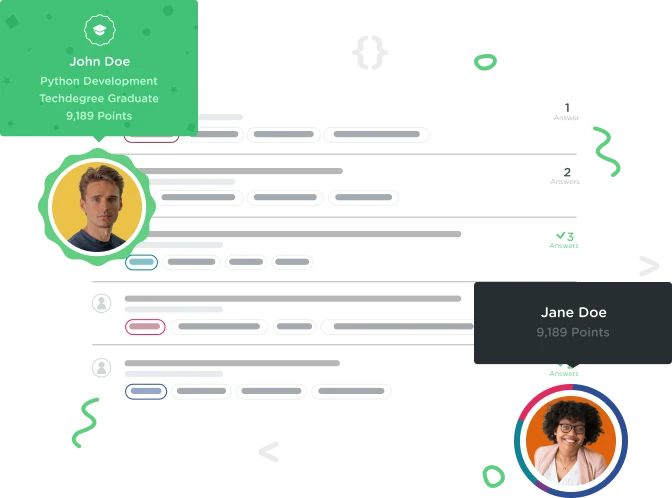
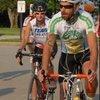
Gerald Tinney
15,454 PointsDid you instantiate a new JsonSerializer? cant get past this challenge, please help
help!
using System;
using System.IO;
using System.Collections.Generic;
using Newtonsoft.Json;
namespace Treehouse.CodeChallenges
{
public class Program
{
public static List<WeatherForecast>DeserializeWeather(string fileName )
{
var serializer = new JsonSerializer ();
using (StreamReader reader = new StreamReader(fileName))
using (var jsonReader = new JsonTextReader(reader))
{
}
var weatherForecasts = new List<WeatherForecast>();
return weatherForecasts;
}
public static void Main(string[] arg)
{
}
public static WeatherForecast ParseWeatherForecast(string[] values)
{
var weatherForecast = new WeatherForecast();
weatherForecast.WeatherStationId = values[0];
DateTime timeOfDay;
if (DateTime.TryParse(values[1], out timeOfDay))
{
weatherForecast.TimeOfDay = timeOfDay;
}
Condition condition;
if (Enum.TryParse(values[2], out condition))
{
weatherForecast.Condition = condition;
}
int temperature;
if (int.TryParse(values[3], out temperature))
{
weatherForecast.Temperature = temperature;
}
double precipitation;
if (double.TryParse(values[4], out precipitation))
{
weatherForecast.PrecipitationChance = precipitation;
}
if (double.TryParse(values[5], out precipitation))
{
weatherForecast.PrecipitationAmount = precipitation;
}
return weatherForecast;
}
}
}
using System;
/* Sample JSON
[
{
"weather_station_id": "HGKL8Q",
"time_of_day": "06/11/2016 0:00",
"condition": "Rain",
"temperature": 53,
"precipitation_chance": 0.3,
"precipitation_amount": 0.03
},
{
"weather_station_id": "HGKL8Q",
"time_of_day": "06/11/2016 6:00",
"condition": "Cloudy",
"temperature": 56,
"precipitation_chance": 0.08,
"precipitation_amount": 0.01
},
{
"weather_station_id": "HGKL8Q",
"time_of_day": "06/11/2016 12:00",
"condition": "PartlyCloudy",
"temperature": 70,
"precipitation_chance": 0,
"precipitation_amount": 0
},
{
"weather_station_id": "HGKL8Q",
"time_of_day": "06/11/2016 18:00",
"condition": "Sunny",
"temperature": 76,
"precipitation_chance": 0,
"precipitation_amount": 0
},
{
"weather_station_id": "HGKL8Q",
"time_of_day": "06/11/2016 19:00",
"condition": "Clear",
"temperature": 74,
"precipitation_chance": 0,
"precipitation_amount": 0
}
]
*/
namespace Treehouse.CodeChallenges
{
public class WeatherForecast
{
public string WeatherStationId { get; set; }
public DateTime TimeOfDay { get; set; }
public Condition Condition { get; set; }
public int Temperature { get; set; }
public double PrecipitationChance { get; set; }
public double PrecipitationAmount { get; set; }
}
public enum Condition
{
Rain,
Cloudy,
PartlyCloudy,
PartlySunny,
Sunny,
Clear
}
}
Challenge Task 4 of 4
Inside the using block, instantiate a new JsonSerializer named serializer. Call the Deserialize method on the serializer object. The Deserialize method should take type parameter of List<WeatherForecast> and take the jsonReader object as a method parameter. Assign the result of calling the Deserialize method to the weatherForecasts variable.
2 Answers
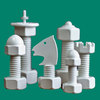
Steven Parker
229,744 Points I got your message.
It doesn't look like you've written anything inside the using block.
Your instantiation of the new JsonSerializer looks good, but it's above the using block instead of inside it. Also, you'll need to put the initial assignment of weatherForecasts up above the using block.
Then, you're only missing the final assignment of weatherForecasts done with the serializer. If you construct a call to serializer.Deserialize with the arguments the challenge specifies, it will look something like this:
weatherForecasts = serializer.Deserialize<List<WeatherForecast>>(jsonReader);
If this still seems unclear, you may want to re-watch the last course video,

Ulyssa Sneed
8,894 Pointsthis didnt work for me