Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial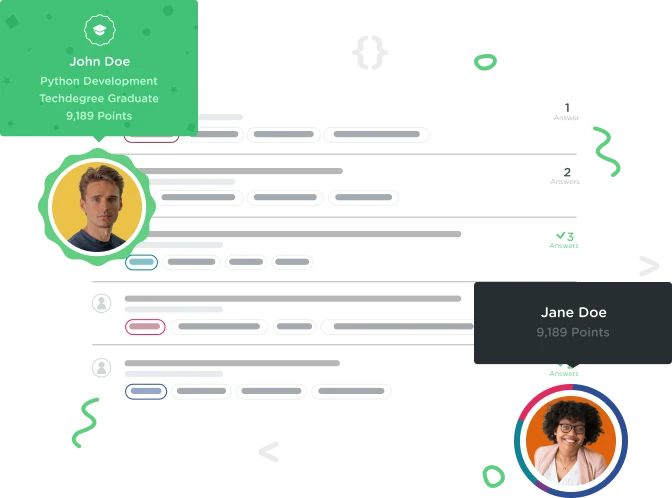
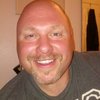
Eric Wolkoff
4,475 Pointsemulator opens but when trying to open Funfact app an error message appears saying the funfact app cannot open.
The funfact app on my emulator was working great! Im at the stage where it is suposed to display a number from 0-2. I clicked save, then clicked play button and thats when I clicked on funfact app and an error message comes up and says the funfact app cannot open. Here is my code? did I miss something? Help
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import java.util.Random;
public class FunFactActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_fact);
//Declare our View variables and assign the Views from the layout file
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
// The button was clicked, so update the fact label with a new fact
String fact = "";
// Randomly select a fact
Random randomGenerator = new Random(); //Construct a new Random number generator
int randomNumber = randomGenerator.nextInt(3);
fact = randomNumber + "";
// Update the label with our dynmaic fact
factLabel.setText(fact);
}
};
showFactButton.setOnClickListener(listener);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.fun_fact, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
7 Answers
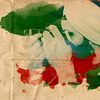
Gunjeet Hattar
14,483 PointsHi Eric,
Just tried with your code, it works fine. So I don't think anything is wrong there.
So was the emulator working fine initially when you started this ? Did you clean the project, closed the emulator and then tried again?
Here is a quick video I created that could be of some help Youtube Video
As an alternative I would suggest you could use the Geny Motion emulator. Its really simple to set up and has lesser lags as compared to the default emulator by android.
And yes its free! :)
Hope it helps.
Treehouse video tutorials on Geny Motion
Ask again if you need help
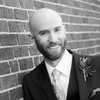
Mark Josephsen
8,803 PointsHave you tried restarting the emulator?
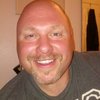
Eric Wolkoff
4,475 Pointsyes. several times.
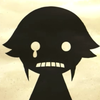
Shaun Snaith
Courses Plus Student 3,024 PointsDo you have any errors in the AVD Manager? The place where you created the emulator.
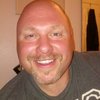
Eric Wolkoff
4,475 PointsNo errors in the AVD Manager. Thanks...did you look at my code? Is the problem in there?
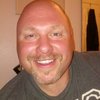
Eric Wolkoff
4,475 PointsIm going to try genymotion. nothing seems to help. The emulator was working great wen I first started. I updated the sdk manager in android studio and all of these problems occurred. it said to install packages so i did. Im hoping I dont have to uninstall and reinstall.

Bryan Mularcik
1,145 PointsI know this might be slightly outside the scope of the issue, but instead of using the emulator maybe you might consider using a real android device?? It's really easy as long as you can get the drivers (Windows?) for your OS. I have an old Samsung Galaxy S2 Epic Touch running Cyanogenmod (Android 4.2) that I use and it runs WAY faster than trying to use an emulator. It's also a lot cooler to see your code running on a real device... There are a couple caveats, such as you have to enable developer mode on the device which is different on each device but you can usually google it and find how to do that - typically it involves something like going to the "About Device" section in the settings and tapping on the model number 7 times in a row or whatever - kind of like an "Easter Egg". Anyway - if you choose to get an Android device for the purpose of testing your code, I would highly recommend using a Nexus (Google) device or a Samsung device - they always have readily available and up to date drivers for your operating system which is SEVERELY important if your using a Windows 8/8.1 machine to run Android Studio...