Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial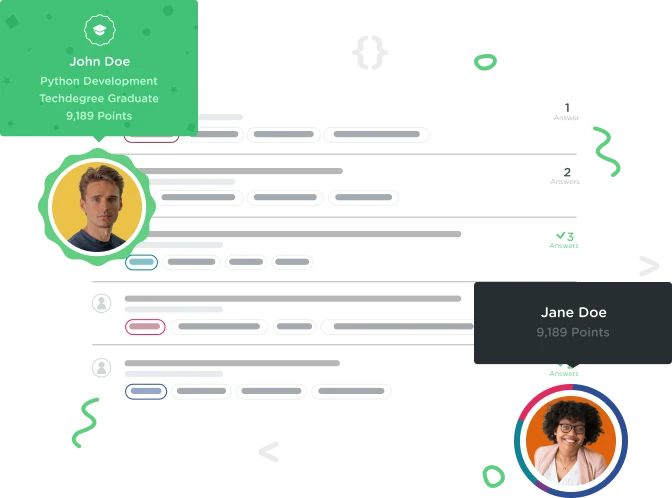

Larry Singleton
18,946 PointsEntity Framework Basics
Question:
In Course.cs add data annotation attributes to the Title property in order to make the following model refinements:
A value will be required and the associated database column will not allow null values
The string value will not exceed 200 characters in length and the associated database column data type will be nvarchar(200)
Code Given:
Course.cs
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class Course
{
public Course()
{
Students = new List<CourseStudent>();
}
public int Id { get; set; }
public int TeacherId { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public int Length { get; set; }
public Teacher Teacher { get; set; }
public ICollection<CourseStudent> Students { get; set; }
}
}
Error:
Bummer! Did you add the 'using' directive for the System.ComponentModel.DataAnnotations namespace?
My Answer:
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class Course
{
public Course()
{
Students = new List<CourseStudent>();
}
public int Id { get; set; }
public int TeacherId { get; set; }
public string Title { get; set; }
[Required, StringLength(200)]
public string Description { get; set; }
public int Length { get; set; }
public Teacher Teacher { get; set; }
public ICollection<CourseStudent> Students { get; set; }
}
}
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class Course
{
public Course()
{
Students = new List<CourseStudent>();
}
public int Id { get; set; }
public int TeacherId { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public int Length { get; set; }
public Teacher Teacher { get; set; }
public ICollection<CourseStudent> Students { get; set; }
}
}
7 Answers

Larry Singleton
18,946 PointsMy apologies Im frustrated and taking it out on you
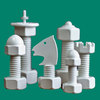
Steven Parker
230,274 PointsLarry Singleton — If you're no longer frustrated, perhaps you would select "best answer" as the one that was most helpful to resolving the issue.

Larry Singleton
18,946 PointsIf the namespace is needed it should either be already there or a part of the question or asked for in the question. It confuses those of us trying to figure this out. I've had a lot of issues in this course with questions not being specific and found myself over doing answers. It's quite irritating. It will be covered in the course review. I do realize it may be me which would be nothing new.
Thanks for the guidance, I will retry the challenge using your suggestions.
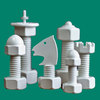
Steven Parker
230,274 PointsTo me, having to add the namespace seemed like a realistic real-world scenario one might encounter in development.

Amit Baniya
13,505 Pointsusing System.ComponentModel.DataAnnotations; using System.Collections.Generic;
namespace Treehouse.CodeChallenges { public class Course { public Course() { Students = new List<CourseStudent>(); }
public int Id { get; set; }
public int TeacherId { get; set; }
[Required,StringLength(200)]
public string Title { get; set; }
[Required,StringLength(200)]
public string Description { get; set; }
public int Length { get; set; }
public Teacher Teacher { get; set; }
public ICollection<CourseStudent> Students { get; set; }
}
}

Amit Baniya
13,505 PointsGet right answer which I have posted above.
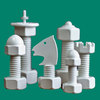
Steven Parker
230,274 PointsThat namespace is required when using data annotations.
So it's not unrelated, it's an important part of the challenge.
Also, the annotations must come before the item they apply to. You've applied them to the Description here instead of the Title.

Larry Singleton
18,946 PointsWhere is this explained?
A property named Grade of type decimal
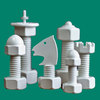
Steven Parker
230,274 PointsOut of context that sounds like just a basic object property, like this:
public decimal Grade { get; set; }

Larry Singleton
18,946 PointsIt should be a part of the question if its required, not assumed the student knows to place it there.
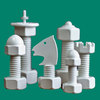
Steven Parker
230,274 PointsThat's a good point, and I've seen that done in other challenges. You might want to make a suggestion to Support about that.

Larry Singleton
18,946 PointsI'll add it in the course review I'll be filling out after this course is over. Again, thank you for all your assistance you are making this a bit clearer. It's still muddy waters tho =P
Larry Singleton
18,946 PointsLarry Singleton
18,946 PointsThe error appears to be asking me to add a line of code that has nothing to do with what is asked by the question, is this the case or am I missing something?