Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial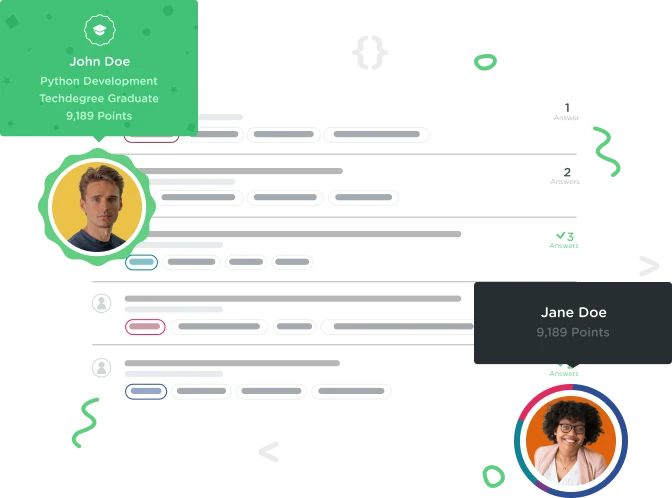

Andre Kucharzyk
4,479 PointsEven though I called assist method I get error message: "Did you remember to call the assist method on the Customer...
Even though I called assist method i get error message: "Did you remember to call the assist method on the CustomerSupportRep?"
import java.util.ArrayDeque;
import java.util.Queue;
public class CallCenter {
Queue<CustomerSupportRep> mSupportReps;
public CallCenter(Queue<CustomerSupportRep> queue) {
mSupportReps = queue;
}
public void acceptCustomer(Customer customer) {
CustomerSupportRep csr;
while (mSupportReps.poll() == null) {
playHoldMusic();
}
/********************************************
* TODO (1)
* Wait until there is an available rep in the queue.
* While there is not one available, playHoldMusic
* HINT: That while assignmentcheck loop syntax we used to
* read files seems pretty similar
********************************************
*/
csr = new CustomerSupportRep("Dre");
csr.assist(customer);
/********************************************
* TODO (2)
* After we have assigned the rep, call the
* assist method and pass in the customer
********************************************
*/
mSupportReps.add(csr);
/********************************************
* TODO (3)
* Since the customer support rep is done with
* assisting, put them back into the queue.
********************************************
*/
}
public void playHoldMusic() {
System.out.println("Smooooooth Operator.....");
}
}
import java.util.List;
import java.util.ArrayList;
public class CustomerSupportRep {
private String mName;
private List<Customer> mAssistedCustomers;
public CustomerSupportRep(String name) {
mName = name;
mAssistedCustomers = new ArrayList<Customer>();
}
public void assist(Customer customer) {
System.out.printf("Hello %s, my name is %s, how can I assist you.%n",
customer.getName(),
mName);
System.out.println("...");
System.out.println("Is there anything else I can help you with?");
mAssistedCustomers.add(customer);
}
public List<Customer> getAssistedCustomers() {
return mAssistedCustomers;
}
}
public class Customer {
private String mName;
public Customer(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
3 Answers

Yanuar Prakoso
15,196 PointsHi Andre
The main problem is : You create a new csr using this code:
csr = new CustomerSupportRep("Dre");
This is not allowed thus You must delete that line (that part of the code). You can only use csr that is in the queue. That means you can only poll one csr from the queue if one is available. To do this you just add while assignment that was shown by Craig (if I am not mistaken) in the course video. Like this:
while ((csr = mSupportReps.poll()) == null) {//<- this is how to do while assignment
playHoldMusic();
}
Do you notice how I assigning csr using the poll method while testing it for TRUE or FALSE in the while statement? That is how you should do it.
I hope this can help a little

Andre Kucharzyk
4,479 Pointsdodders I will do it for you. Yanuar is really good at explaining java.
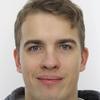
Jaanus R
1,720 PointsYanuar answered to your question.
Because you assign csr to be "Dre" you mess up autocheck at csr.assign(customer).
So the problem lies in self assigned csr as he said.
dodders
Python Development Techdegree Graduate 38,680 Pointsdodders
Python Development Techdegree Graduate 38,680 PointsAlthough not my question I just wanted to say thank you for this answer. It was an excellent explanation. Sadly I cannot click Best Answer for you!