Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial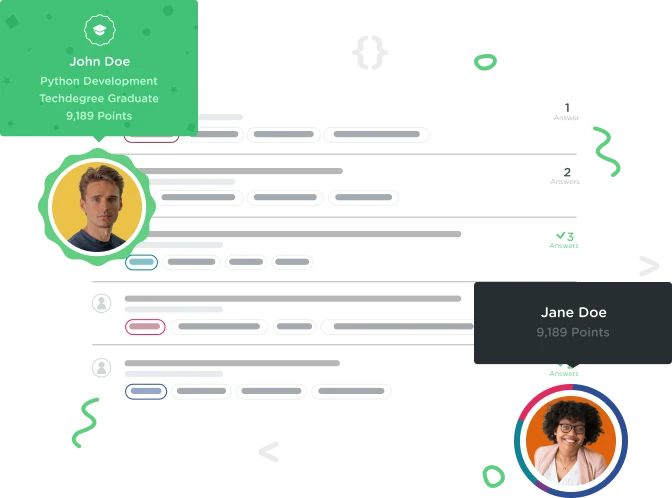
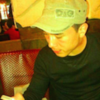
Jonathan Kim
Courses Plus Student 10,765 PointsFailure/Error: click_link "Edit" Capybara::ElementNotFound: Unable to find link "Edit"
todo_items_controller.rb
class TodoItemsController < ApplicationController
def index
@todo_list = TodoList.find(params[:todo_list_id])
end
def new
@todo_list = TodoList.find(params[:todo_list_id])
@todo_item = @todo_list.todo_items.new
end
def create
@todo_list = TodoList.find(params[:todo_list_id])
@todo_item = @todo_list.todo_items.new(todo_item_params)
if @todo_item.save
flash[:success] = "Added todo list item."
redirect_to todo_list_todo_items_path
else
flash[:error] = "There was a problem adding that todo list item."
render action: :new
end
end
def edit
@todo_list = TodoList.find(params[:todo_list_id])
@todo_item = @todo_list.todo_items.find(params[:id])
end
def update
@todo_list = TodoList.find(params[:todo_list_id])
@todo_item = @todo_list.todo_items.find(params[:id])
if @todo_item.update_attributes(todo_item_params)
flash[:success] = "Saved todo list item."
redirect_to todo_list_todo_items_path
else
flash[:error] = "That todo item could not be saved."
render action: :edit
end
end
def url_options
{ todo_list_id: params[:todo_list_id] }.merge(super)
end
private
def todo_item_params
params[:todo_item].permit(:content)
end
end
index.html.erb
<h1><%= @todo_list.title %></h1>
<ul class="todo_items">
<% @todo_list.todo_items.each do |todo_item| %>
<li id="<%= dom_id(todo_item) %>">
<%= todo_item.content %></li>
<%= link_to "Edit", edit_todo_list_todo_item_path(todo_item) %>
<% end %>
</ul>
<p>
<%= link_to "New Todo Item", new_todo_list_todo_item_path %>
</p>
edit.html.erb
<%= form_for [@todo_list, @todo_item] do |form| %>
<% if @todo_item.errors.any? %>
<div id="error_explanation">
<h2><%= pluralize(@todo_item.errors.count, "error") %> prohibited this todo_item from being saved:</h2>
<ul>
<% @todo_item.errors.full_messages.each do |message| %>
<li><%= message %></li>
<% end %>
</ul>
</div>
<% end %>
<%= form.label :content %>
<%= form.text_field :content %>
<%= form.submit "Save" %>
<% end %>
edit_spec.rb
require 'spec_helper'
describe "Editing todo items" do
let!(:todo_list) { TodoList.create(title: "Grocery list", description: "Groceries")}
let!(:todo_item) { todo_list.todo_items.create(content: "Milk")}
def visit_todo_list(list)
visit "/todo_lists"
within "#todo_list_#{list.id}" do
click_link "List Items"
end
end
it "is successful with valid content" do
visit_todo_list(todo_list)
within("#todo_item_#{todo_item.id}") do
click_link "Edit"
end
fill_in "Content", with: "Lots of Milk"
click_button "Save"
expect(page).to have_content("Saved todo list item.")
todo_item.reload
expect(todo_item.content).to eq("Lots of Milk")
end
end
I cannot figure out what is wrong here.. any one have solution?
1 Answer

Seth Kroger
56,414 PointsIn index.html.erb you end the li before the "Edit" link so the link will be outside the dom_id, not within. The li should encompass both the todo item and it's edit link.
<ul class="todo_items">
<% @todo_list.todo_items.each do |todo_item| %>
<li id="<%= dom_id(todo_item) %>">
<%= todo_item.content %>
<%= link_to "Edit", edit_todo_list_todo_item_path(todo_item) %>
</li>
<% end %>
</ul>
Jonathan Kim
Courses Plus Student 10,765 PointsJonathan Kim
Courses Plus Student 10,765 Pointswow... a very simple error got my whole day ruined.. it is my birthday too..lol Hey Seth, thank you very much and now I can enjoy my birthday!