Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial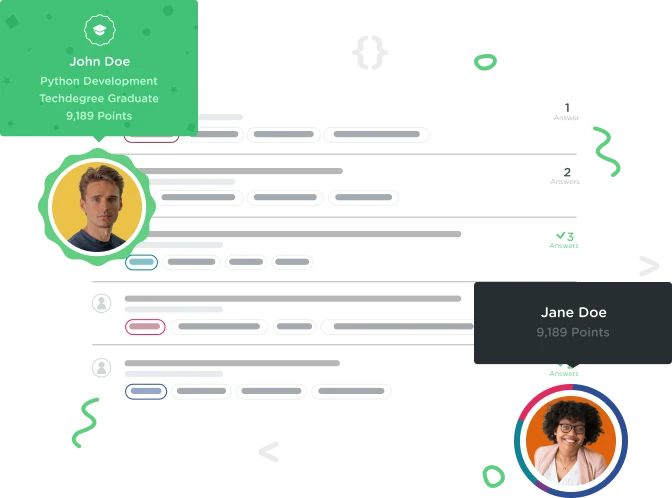

mostafa dahab
2,811 Pointsfirst 4 and last 4 items in the iterable
re watch video and can't get it
4 Answers
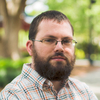
Kenneth Love
Treehouse Guest TeacherYou're expected to use slices. So the first four items would be [:4]
, from 0 to 4 (0, 1, 2, 3). The last four would be [-4:]
or get the length of the list, subtract 4, and go from there to the end :]
. If you have to send them back together, you can add slices together.
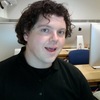
Nathan Smutz
9,492 PointsI imagine it's the "last 4" that's tricky.
Say
a = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> # What we want is [0, 1, 2, 3] and [6, 7, 8, 9]
>>> # Now the first part isn't that bad.
... # We can get an element pretty easy
... a[0]
0
>>> a[1]
1
>>> a[2]
2
>>> a[0], a[1], a[2]
(0, 1, 2)
>>> # Now there's a shorthand for grabbing a "slice" of an iterable:
... a[0:4]
[0, 1, 2, 3]
>>> # This grabbed elements a[0] up through a[3]
... # Notice 4 is one-greater than the last element
... # You've probably noticed this is pretty common
... # in anything that iterates.
...
>>> # There's another trick with slices.
... # If you leave off one or the other of the numbers, it "slices"
... # from the beginning (no left number [ :3])
>>> a[ :3]
[0, 1, 2]
>>> # or stops slicing
... # with the end (no right number [3: ])
>>> a[3: ]
[3, 4, 5, 6, 7, 8, 9]
>>> # So our first part is done
... # We want the first four elements
... # Those are elements 0-3
... # So we just take a slice up to (not including)
... # element 4:
... a[:4]
[0, 1, 2, 3]
>>>
>>> # Now the last part is kind of done if we know how many
... # elements are in the iterable and don't mind some arithmetic.
... # However there's an easier (and more elegant) way:
... # Lets look at our list again:
>>> a
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
# Now watch this:
... a[-1]
9
>>> a[9]
9
# See both a[9] and a[-1] point to the last item in our list...
>>> a[-2]
8
>>> a[-3]
7
>>> # As you can see, negative indices are counted backwards
... # from the end of the iterable; starting with -1
... # as the last element.
... # So what we want for the last 4 elements is:
... a[-4], a[-3], a[-2], a[-1]
(6, 7, 8, 9)
>>> # Using slice notation, we use the "lower"
... # index first like usual; but since going one index
... # higher than -1 (0) wont work here we'll use the no-upper-bound
... # trick and leave off the second index in the slice [-4: ]
... # so the slice will take elements up to and including the last one:
... a[-4: ]
[6, 7, 8, 9]
>>> # So our first four and last four elements are:
... a[:4] + a[-4:]
[0, 1, 2, 3, 6, 7, 8, 9]
Ta Da!
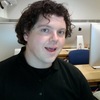
Nathan Smutz
9,492 PointsSorry. I put this together offline before I saw wthe other answers. Maybe it'll be useful to somebody.

mostafa dahab
2,811 Pointsgreat great explain thank you !! (Y)

mostafa dahab
2,811 Pointsit's helpful ! it's takes me into deep understand what's going ... thanks man :D

mostafa dahab
2,811 Pointsyes ,, i know that [:4] the first 4 items and [-4:] the last 4 items but i think there is a slice that do it in one time
return lst[:4] + lst[-4:] //correct answer
thanks a lot <3
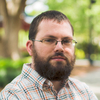
Kenneth Love
Treehouse Guest TeacherI don't know of any way to do it in one single slice since there's a gap in there.

mostafa dahab
2,811 Pointsthis is what i'am understand from question ,, now it's clear thanks :)

Jordan Kehrer
7,871 PointsWhy does return list[:4].extend(list[-4:]) not work?
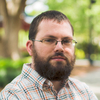
Kenneth Love
Treehouse Guest TeacherBecause .extend()
doesn't return a list for the return
to return. No actions on lists, since they're mutable, other than .copy()
return a list, they just modify the list they're called on in-place.