Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial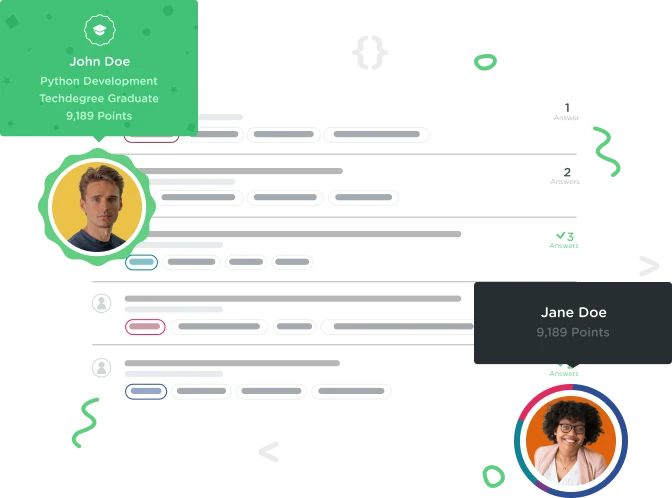

ahmed alghafli
3,446 Pointshave added a method drive that defines a parameter laps. These high-powered GoKarts use 1 energy bar off their battery
what should I do
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
3 Answers

Clayton Perszyk
Treehouse Moderator 48,735 Pointscreate another drive method like this:
public void drive() {
}
You'll notice there are no arguments for this drive method; after adding this method you will have two drive methods in your GoKart class, but they have different signatures (in this case one method takes an argument and the other doesn't), so they won't be in conflict with one another.
Inside the new drive method you want to call the original drive method and pass it an int value of 1:
drive(1);
Combine those two snippets together and the code should pass.

Mahdi Darwish
945 PointsWorks great! Thanks
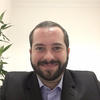
Augusto Hernandes
1,818 PointsI still don't understand :(

Clayton Perszyk
Treehouse Moderator 48,735 PointsYou want to create another public void function named drive that doesn't take any arguments; within that function you call the original drive method, passing in the number 1 as the argument for laps. Because the new function has a different method signature, it won't conflict with the original function. Here's a link to the wikipedia page for method signatures: http://en.wikipedia.org/wiki/Type_signature#Method_signature

ahmed alghafli
3,446 PointsI am still confused

John Weland
42,478 Pointsahmed alghafli what is happening here in this answer above is
there is an existing method called drive that takes in an int called laps, and for every lap it decrements the mBarsCount by 1.
then you have to create a new method called drive that takes in to parameters, that method drive that you created calls the existing method drive by the number you pass into it like so.
//existing method
public void drive(int laps) {
mBarsCount -= laps; //decrement mBarsCount 1 time for each laps
}
// New method you create
public void drive() {
drive(1); // this calls the existing drive method where 1 is the number of laps
}
ahmed alghafli
3,446 Pointsahmed alghafli
3,446 Pointshave added a method drive that defines a parameter laps. These high-powered GoKarts use 1 energy bar off their battery per lap. Using method signatures, add a new default method called drive that performs just 1 lap and takes no arguments. this is the question