Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial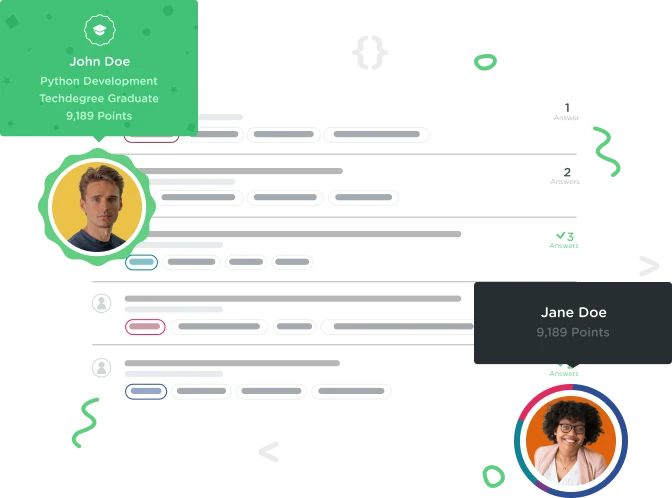
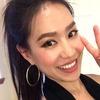
Grace Ji
5,402 PointsI don't understand this part: var result = dice.roll();
at 1:50 on the video, Andrew changes var result = 1; to var result = dice.roll(); I thought I have to bring the whole variable into var result like this. var result = var dice; variable can't have another variable as a property?
2 Answers

Aaron Price
5,974 PointsI haven't been following the series, but I'm pretty sure I can guess what's tripping you up. It's a lot deeper than you might expect, but if you can master this it'll take you far! Consider the following:
// In the following code, we ask for the value of bar. You get an error: "Bar wasn't DEFINED". One of the bad parts of js is that in this case it SHOULD say "not DECLARED", but doesn't. It says "not DEFINED".
bar
// Here we DECLARE bar without DEFINING it.
var bar
// Here we ask for the VALUE of bar again. It's undefined but not an error, because it was already DECLARED
bar
// Here we DECLARE foo, and DEFINE it by ASSIGNING it to a VALUE
var foo = "hello"
// Here we ask for the VALUE of foo, and get back the string "hello"
foo
// Here we're trying to DECLARE two different variables. That doesn't make sense, and will error
var arr = var foo
// Here we DECLARE arr and DEFINE it by ASSIGNING it to the VALUE of foo, which is the string "hello"
var arr = foo
// Therefore, asking for the value of arr will return the string "hello"
arr
Now your question was similar, just with objects and functions. Side note on semantics: a method is just a function attached to an object. Note that calling a function or method by it's name will return the function itself. Calling it with parenthesis attached will give the RETURN VALUE of the function.
// Let's define the object dice
dice = {
roll: function() { return 42; }
}
// Here we ask for the OBJECT dice. It is { roll: function(){ return 42; } }
dice
// Here we ask for the RETURN VALUE of the method roll. It is 42.
dice.roll()
// Here we ask for the method itself. It is function() { return 42; }
dice.roll
//Now finally your answer should be clearer. The following gives an error, you're trying to declare two things at once
var result = var dice
// Below, result is assigned to the object itself.
var result = dice
// below, we ask for the value of result, which is an object. { roll: function(){ return 42; } }
result
// Next, result is reassigned to the method itself.
var result = dice.roll
// We call it, get back the value, which is a function. function(){ return 42; }
result
// Finally, result is reassigned to the RETURN VALUE of the method.
var result = dice.roll()
// we call result, and get back the number 42.
result

Neil McPartlin
14,662 PointsAaron Price, take a bow. Nice work.
...but if you can master this it'll take you far!
Oh so true. Many thanks.

Rafael de Oliveira
11,174 Points"dice" is a object. "roll()" is a function of the object (a method). So, "dice.roll()" will give you a number automatically.
Wesley Pak
4,277 PointsWesley Pak
4,277 PointsRemember from the previous video that we had created inside the object, 'dice', well there was a function inside it named roll, that is where it came from. dice.roll is not a variable. it is just a function inside a variable, you dont have to use the this method on it, by saying just the name, it does the same thing. Boom 10 year child solved the case.