Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial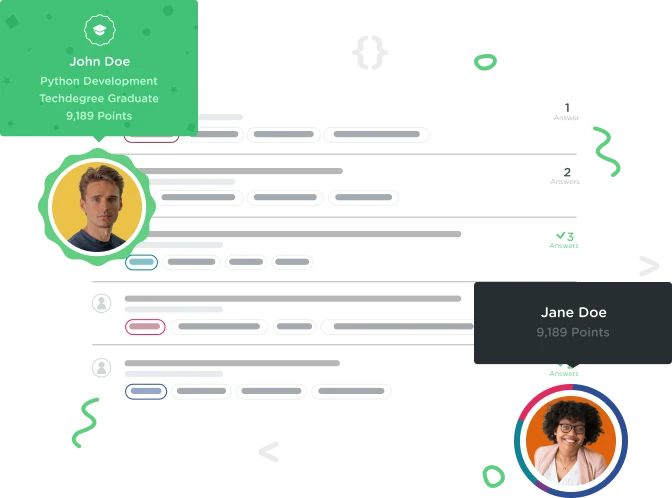

gabrielalcaraz
7,694 PointsI entered "2". I expected "You entered 2" but got "Value is out of bounds!" instead.
I need help with this problem
int value = int.Parse(Console.ReadLine());
try
{
if (value > 0 || value < 20)
{
throw new System.Exception();
}
else
{
Console.WriteLine(string.Format("You entered {0}",value));
}
}
catch (Exception e)
{
Console.WriteLine("Value is out of bounds!");
}
1 Answer

Ross Nation
4,678 PointsHey so there are a few things here to look at. :) What you're currently saying in your if statement is
if the value is more than 0, or the value is less than 20, throw an exception
This basically means, any value you input will throw an exception because all values are either greater than 0 OR less than 20.
There are a few ways to fix this, depending on how you want to approach it. You could change your code to say
if the value is more than 0 AND the value is less than 20, Console.Writeline($"You entered {value}"), else throw exception
Or you could change the direction of your greater/less than symbols. So instead you'd be saying:
if the value is less than 0 OR the value is greater than 20, throw new exception
Hopefully that makes sense. :) Keep at it!