Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial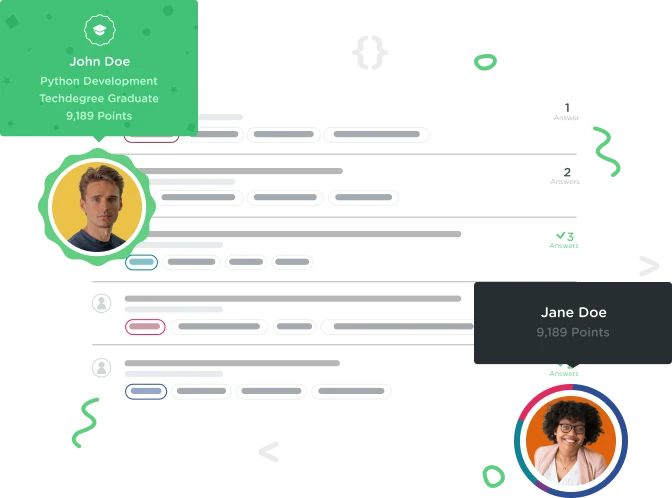

Eric Gentry
6,197 PointsIn the normalizeDiscountCode verify that only letters or the $ character are used. If any other character is used,
I am confused on what I need to do on this section to complete it.
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
private String normalizeDiscountCode(String discountCode) {
return discountCode.toUpperCase();
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
1 Answer

Boban Talevski
24,793 PointsHi, I've been asked to answer this question.
So, here goes:
We are creating a for each loop which will loop over every character in the discountCode string inside normalizeDiscountCode method. We do this by using the toCharArray() method which will create an array of all the chars of the passed in discountCode parameter. We'll loop over this array.
Inside the loop, for every character we then check if the character is a letter or the symbol $. If that turns out to be false (notice the ! sign before the whole expression), we throw a new IllegalArgumentException with the requested message.
If all the characters are actually letters or the symbol $ that exception never gets raised and the method completes the usual way and returns the discountCode uppercased as in the Task 1 of the challenge.
This is the code:
private String normalizeDiscountCode(String discountCode) {
for (char c : discountCode.toCharArray()) {
if (!(Character.isLetter(c) || c == '$')) {
throw new IllegalArgumentException("Invalid discount code");
}
}
return discountCode.toUpperCase();
}
Hope it helps.
Martin Svensen
7,464 PointsMartin Svensen
7,464 PointsThank you. It worked