Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial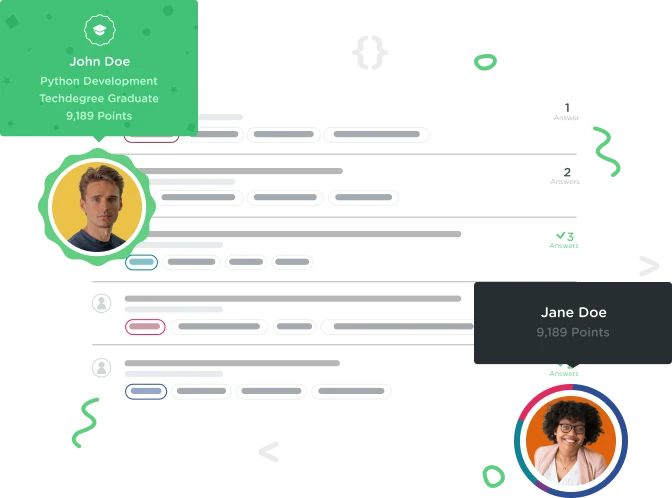
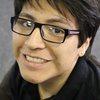
niels Lostaunau
638 Pointsis it possible to tell if the stars are overlapping from looking at the ordinates?
even though I don't see the stars overlapping it still tells "bummer"
var game;
var player;
var platforms;
var badges;
var stars;
var poisons;
var cursors;
var jumpButton;
var scoreText;
var livesText;
var finalMessage;
var won = false;
var gameOver = false;
var currentScore = 0;
var lives = 3;
var winningScore = 100;
function createStars() {
stars = game.add.physicsGroup();
starCreate(425, 100, 'star');
starCreate(315, 100, 'star');
starCreate(175, 200, 'star');
starCreate(300, 250, 'star');
starCreate(340, 290, 'star');
starCreate(300, 330, 'star');
}
function createPoisons() {
poisons = game.add.physicsGroup();
poisonCreate(675, 275, 'poison');
poisonCreate(450, 375, 'poison');
poisonCreate(375, 475, 'poison');
}
function createPlatforms() {
platforms = game.add.physicsGroup();
platforms.create(300, 150, 'platform');
platforms.create(400, 250, 'platform');
platforms.create(100, 250, 'platform');
platforms.create(500, 350, 'platform');
platforms.create(400, 450, 'platform');
platforms.create(300, 550, 'platform');
platforms.setAll('body.immovable', true);
}
function starCreate(left, top, starImage) {
var star = stars.create(left, top, starImage);
star.animations.add('spin');
star.animations.play('spin', 8, true);
}
function poisonCreate(left, top, poisonImage) {
var poison = poisons.create(left, top, poisonImage);
poison.animations.add('bubble');
poison.animations.play('bubble', 8, true);
}
function starCollect(player, star) {
star.kill();
currentScore = currentScore + 20;
if (currentScore === winningScore) {
won = true;
}
}
function poisonCollect(player, poison) {
poison.kill();
lives = lives + 1;
if (lives === 0) {
player.kill();
gameOver = true;
}
}
window.onload = function () {
game = new Phaser.Game(800, 600, Phaser.AUTO, '', { preload: preload, create: create, update: update, render: render });
function preload() {
game.stage.backgroundColor = '#89889c';
//Load images
game.load.image('platform', 'platform.png');
//Load spritesheets
game.load.spritesheet('player', 'mikethefrog.png', 50, 60);
game.load.spritesheet('poison', 'poison.png', 24, 62);
game.load.spritesheet('star', 'star.png', 54, 52);
game.load.spritesheet('badge', 'badge.png', 42, 54);
}
function create() {
player = game.add.sprite(150, 600, 'player');
player.animations.add('walk');
player.anchor.setTo(0.5, 1);
game.physics.arcade.enable(player);
player.body.collideWorldBounds = true;
player.body.gravity.y = 500;
createStars();
createPoisons();
createPlatforms();
cursors = game.input.keyboard.createCursorKeys();
jumpButton = game.input.keyboard.addKey(Phaser.Keyboard.SPACEBAR);
scoreText = game.add.text(16, 16, "SCORE: " + currentScore, { font: "24px Arial", fill: "white" });
livesText = game.add.text(685, 16, "LIVES: " + lives, { font: "24px Arial", fill: "white" });
finalMessage = game.add.text(game.world.centerX, 250, "", { font: "48px Arial", fill: "white" });
finalMessage.anchor.setTo(0.5, 1);
}
function update() {
scoreText.text = "SCORE: " + currentScore;
livesText.text = "LIVES: " + lives;
game.physics.arcade.collide(player, platforms);
game.physics.arcade.overlap(player, stars, starCollect);
game.physics.arcade.overlap(player, poisons, poisonCollect);
player.body.velocity.x = 0;
if (cursors.left.isDown) {
player.animations.play('walk', 10, true);
player.body.velocity.x = -350;
player.scale.x = - 1;
}
else if (cursors.right.isDown) {
player.animations.play('walk', 10, true);
player.body.velocity.x = 350;
player.scale.x = 1;
}
else {
player.animations.stop();
}
if (jumpButton.isDown && (player.body.onFloor() || player.body.touching.down)) {
player.body.velocity.y = -400;
}
if (won) {
finalMessage.text = "YOU WIN!!!";
}
if (gameOver) {
finalMessage.text = "GAME OVER!!!";
}
}
function render() {
}
};
2 Answers
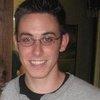
David Evans
10,490 PointsHi Niels,
With a little math it is possible to see if the stars overlap, there are a few parts you need to take into account.
function createStars() {
stars = game.add.physicsGroup();
starCreate(425, 100, 'star');
starCreate(315, 100, 'star');
starCreate(175, 200, 'star');
starCreate(300, 250, 'star');
starCreate(340, 290, 'star');
starCreate(300, 330, 'star');
}
This is where you're physically creating the stars. The first parameter you're passing into the starCreate function is the left position. The second parameter is the top position.
You can see this by looking at the function further down in the code:
function starCreate(left, top, starImage) {
var star = stars.create(left, top, starImage);
star.animations.add('spin');
star.animations.play('spin', 8, true);
}
Now this alone will give you a good guess of whether or not they are overlapping, but if the stars are relatively small in width and height, you might not know.
It looks like you're working with Phaser JS framework and in your preload function you have this line:
game.load.spritesheet('star', 'star.png', 54, 52);
From the documentation in Phaser, you'll see that 54 relates to the width and 52 relates to the height of the image.
Documentation: https://phaser.io/examples/v2/loader/load-spritesheet
If you take the width of the star being 54px and add it to the 1stparameter you're passing into starCreate() and the height of 52px and pass it into the 2nd parameter into starCreate() you can get a pretty good idea of whether they're overlapping or not.
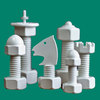
Steven Parker
229,771 PointsThey may not appear to overlap visually, but you have to account for their full dimensions (54x52, disregard the fact that parts of them are transparent).
So if you compute the outlines of the two new ones, you can see they do overlap in a corner.