Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial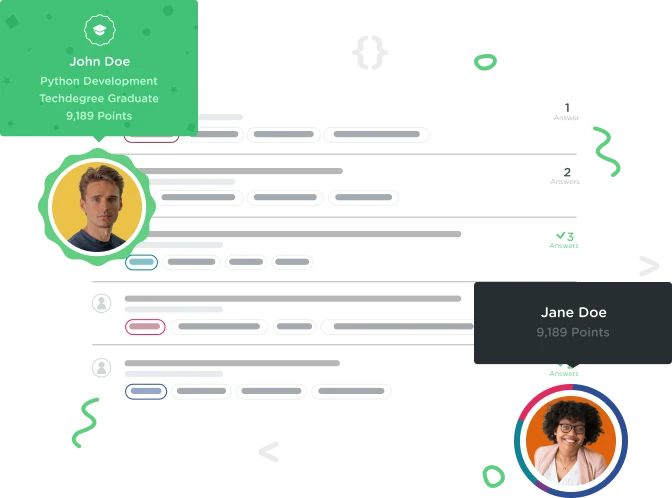

Damon Holaday
10,503 PointsKeep getting Uncaught SyntaxError: Unexpected end of input at the end of my quiz answer.
My code is below. It looks like everything is correct except the apparent unexpected end of input error.
// 1. Create a multidimensional array to hold quiz questions and answers const questions = [ ['What planet is closest to earth?','Mercury'], ['What planet is farthest from earth?','Saturn'], ['What planet is halfway between the sun and Pluto?','Uranus'], ['Is the Sun a planet?','Its a Star'] ];
// 2. Store the number of questions answered correctly const correctAnswers = 0; const correct = []; const incorrect =[];
/*
-
Use a loop to cycle through each question
- Present each question to the user
- Compare the user's response to answer in the array
- If the response matches the answer, the number of correctly answered questions increments by 1 */
for ( let i = 0; i < questions.length; i++ ) { let question = questions[i][0]; let answer = answers[i][0]; let response = prompt(question);
if ( response === answer ){ correctAnswers++; correct.push(question); } else { incorrect.push(question); }
// 4. Display the number of correct answers to the user
function createListItems( arr ) {
let items = '';
for ( let i = 0; i < arr.length; i++ ) {
items += <li>${ arr[i]}</li>
;
}
return items;
}
let html = ` <h1>You got ${correctAnswers} question(s) correct</h1> <h2>You got these questions right:</h2> <ol>${ createListItems(correct) }</ol>
<h2>You got these questions wrong:</h2> <ol>${ createListItems(incorrect) }</ol> `;
document.querySelector('main').innerHTML = html;
3 Answers
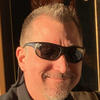
Peter Vann
36,427 PointsHi Damon!
You have a few slight oversights.
I looked at your code, did some testing, fixed several problems, and got it working.
I added comments to point out my changes
Here's the updated code:
<!DOCTYPE html>
<html>
<body>
<main></main><!-- YOUR RESULT WILL PRINT HERE -->
<script>
// 1. Create a multidimensional array to hold quiz questions and answers
const questions = [
['What planet is closest to earth?', 'Mercury'],
['What planet is farthest from earth?', 'Saturn'],
['What planet is halfway between the sun and Pluto?', 'Uranus'],
['Is the Sun a planet?', 'It is a Star'] // Changed Its to It is
];
// 2. Store the number of questions answered correctly
let correctAnswers = 0; // Used let instead of const
let correct = []; // Used let instead of const
let incorrect = []; // Used let instead of const
/*
Use a loop to cycle through each question
Present each question to the user
Compare the user's response to answer in the array
If the response matches the answer, the number of correctly answered questions increments by 1 */
for (let i = 0; i < questions.length; i++) {
let question = questions[i][0];
let answer = questions[i][1]; // Changed answers[i][0] to questions[i][1];
let response = prompt(question);
if ( response === answer ){
correctAnswers++;
correct.push(question);
} else {
incorrect.push(question);
}
} // You were missing this curly brace. THIS WAS CAUSING THE "Unexpected end of input" ERROR.
// 4. Display the number of correct answers to the user
function createListItems( arr ) {
let items = '';
for ( let i = 0; i < arr.length; i++ ) {
items += `<li>${arr[i]}</li>`; // You were missing backticks here
}
return items;
}
// I tightened this code up just slightly (removed some spaces)
let html = `<h1>You got ${correctAnswers} question(s) correct</h1><h2>You got these questions right:</h2> <ol>${ createListItems(correct) }</ol><h2>You got these questions wrong:</h2> <ol>${ createListItems(incorrect) }</ol>`;
document.querySelector('main').innerHTML = html;
</script>
</body>
</html>
You can test it here:
Copy all the HTML, paste it in the left pane (replace all the existing code), and run it.
Get used to relying on the console for debugging, if you haven't already.
One thing a did to test/fix things is delete most of the code until the errors went away and then added back each next block of code until an error popped up, and that helped me pinpoint each error systematically.
Let me know if you have further questions.
I hope that helps.
Stay safe and happy coding!

Damon Holaday
10,503 PointsThanks Peter!
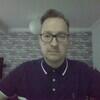
Brian Wright
6,770 PointsGreat answer from peter. Before running any code in the browser I always open my developer console at the bottom of the page. It will point you straight to the line where you have the problem in most cases.