Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial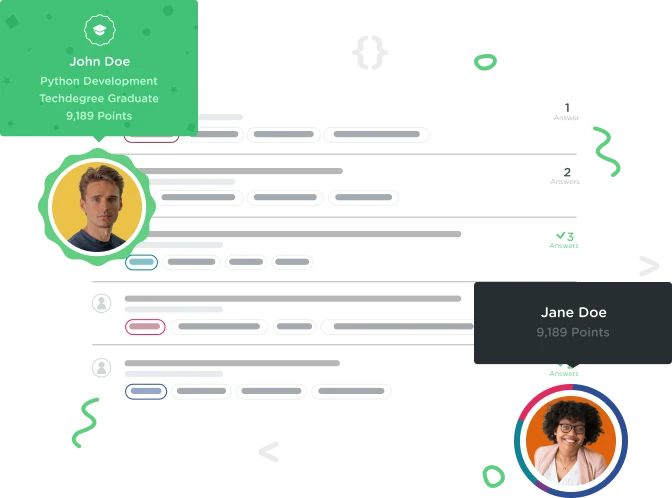

Eris Ramirez
5,916 Points" Make sure that the initializer returns a valid instance given a valid dictionary "what this means?
I need to know what that Bummer! means so I can finish this code I have tried a bunch of differents codes but I still don't get it
struct Book {
let title: String
let author: String
let price: String?
let pubDate: String?
init?(dict: [String : String]){
self.title = "er"
self.author = "Eris Ramirez"
self.price = nil
self.pubDate = nil
return nil
}
}
let instance = ["title": "Los que ganan" , "author": "Eris Ramirez", "price": "150", "pubDate": "nil"]
Book(dict: instance)
3 Answers
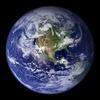
tromben98
13,273 PointsHi Eris!
You need to configure your initializer using a guard statement. Then you can access the dictionary values using the right keys, for example: dict["title"].
Best regards, Jonas
struct Book {
let title: String
let author: String
let price: String?
let pubDate: String?
init?(dict: [String : String]){
guard let title = dict["title"], let author = dict["author"] else {
return nil
}
self.title = title
self.author = author
self.price = dict["price"]
self.pubDate = dict["pubDate"]
}
}
let instance = ["title": "Los que ganan" , "author": "Eris Ramirez", "price": "150", "pubDate": "nil"]
Book(dict: instance)

Ignazio Calo
Courses Plus Student 1,819 PointsThe challenge is to write a "failable" initializer or, in other words, an initializer that can give you back a valid object or nil
if something is wrong.
If you look at your code you're always returning nil
no matter if the dict
parameter is valid or not.
So what you need to do is to check if the dict
contains the values that are required to create your Book, and return nil
if one of the two values are missing, or return a valid object in the other case.
You also need to check only two fields on the dictionary, because only the title
and author
are mandatory, the other two properties price
and pubDate
are optional therefore even if you don't have any value inside the dictionary for those properties you can still return a valid object.
Of course the solution posted from tromben98 works fine, I just wanted add more context.
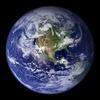
tromben98
13,273 PointsGood work Ignazio!
I wasn't sure how to express myself, Thanks!

Eris Ramirez
5,916 PointsThanks a lot to everyone It works perfectly